How to Use Ruby Functions for Cryptography and Security
In an increasingly digital world, ensuring the security of your data and communications is paramount. Whether you’re working on a web application, handling sensitive information, or simply want to protect your users’ privacy, cryptography is an essential tool. Ruby, a dynamic and versatile programming language, provides a range of functions and libraries for implementing cryptographic techniques effectively.
Table of Contents
In this comprehensive guide, we will delve into the world of Ruby cryptography and security functions. We will cover encryption, hashing, digital signatures, and more, offering practical examples and explanations to help you fortify your applications against potential threats.
1. Understanding the Basics of Cryptography
1.1 What Is Cryptography?
Cryptography is the science of securing communication and information by converting it into an unreadable format, known as ciphertext, and then decrypting it back to its original form, known as plaintext. This process involves the use of mathematical algorithms and keys to ensure the confidentiality, integrity, and authenticity of data.
Cryptography serves various purposes, including:
Confidentiality: Ensuring that unauthorized users cannot access sensitive information.
Integrity: Verifying that data has not been tampered with during transmission or storage.
Authenticity: Confirming the identity of the sender or source of the data.
1.2 Types of Cryptographic Techniques
There are two main types of cryptographic techniques:
- Symmetric Cryptography: In symmetric cryptography, the same key is used for both encryption and decryption. This means that the sender and the receiver must have access to the same secret key. It’s efficient for encrypting large amounts of data.
- Asymmetric Cryptography: Asymmetric cryptography uses a pair of keys: a public key for encryption and a private key for decryption. Information encrypted with the public key can only be decrypted with the corresponding private key. This approach is commonly used for secure communication and digital signatures.
1.3 The Role of Ruby in Cryptography
Ruby provides a robust set of libraries and functions for implementing cryptographic techniques. These built-in capabilities make it easier for Ruby developers to secure their applications without having to reinvent the wheel. Let’s explore some of the key cryptography functions Ruby has to offer.
2. Encryption in Ruby
Encryption is a fundamental aspect of cryptography. It allows you to protect sensitive data by converting it into an unreadable format, which can only be deciphered with the correct key. Ruby supports both symmetric and asymmetric encryption.
2.1 Symmetric Encryption
Symmetric encryption, as the name suggests, uses the same key for both encryption and decryption. Ruby’s OpenSSL library provides a straightforward way to perform symmetric encryption.
ruby require 'openssl' # Generate a random key and IV (Initialization Vector) key = OpenSSL::Cipher.new('AES-256-CFB').random_key iv = OpenSSL::Cipher.new('AES-256-CFB').random_iv # Your plaintext data plaintext = 'This is a secret message.' # Encrypt the data cipher = OpenSSL::Cipher.new('AES-256-CFB').encrypt cipher.key = key cipher.iv = iv encrypted_data = cipher.update(plaintext) + cipher.final # Decrypt the data decipher = OpenSSL::Cipher.new('AES-256-CFB').decrypt decipher.key = key decipher.iv = iv decrypted_data = decipher.update(encrypted_data) + decipher.final puts "Original data: #{plaintext}" puts "Encrypted data: #{encrypted_data.unpack('H*')[0]}" puts "Decrypted data: #{decrypted_data}"
In this example, we generate a random key and initialization vector (IV) for AES-256-CFB encryption. We then encrypt and decrypt a sample message using the same key and IV.
2.2 Asymmetric Encryption
Asymmetric encryption uses a pair of keys: a public key for encryption and a private key for decryption. Ruby’s OpenSSL library also supports asymmetric encryption.
ruby require 'openssl' # Generate a key pair key_pair = OpenSSL::PKey::RSA.generate(2048) # Your plaintext data plaintext = 'This is a confidential message.' # Encrypt with the public key encrypted_data = key_pair.public_encrypt(plaintext, OpenSSL::PKey::RSA::PKCS1_OAEP_PADDING) # Decrypt with the private key decrypted_data = key_pair.private_decrypt(encrypted_data, OpenSSL::PKey::RSA::PKCS1_OAEP_PADDING) puts "Original data: #{plaintext}" puts "Encrypted data: #{encrypted_data.unpack('H*')[0]}" puts "Decrypted data: #{decrypted_data}"
In this example, we generate an RSA key pair and use the public key to encrypt the message and the private key to decrypt it.
2.3 Using OpenSSL for Encryption
OpenSSL is a widely-used open-source library that provides cryptographic functions for various programming languages, including Ruby. It offers robust encryption algorithms and is suitable for a wide range of security-related tasks. When using OpenSSL with Ruby, ensure that you have the OpenSSL library installed on your system.
To install the OpenSSL gem in your Ruby project, you can use the following command:
shell gem install openssl
Once installed, you can use OpenSSL in your Ruby applications to implement encryption and other cryptographic operations.
3. Hashing for Data Integrity
Hashing is another essential aspect of cryptography, primarily used to verify data integrity. Hash functions take an input (or ‘message’) and return a fixed-size string of bytes, typically a hexadecimal number. Even a small change in the input data will result in a significantly different hash value. This property makes hash functions useful for checking data integrity.
3.1 What Is Hashing?
Hashing serves several purposes in cryptography and computer science:
- Data Integrity: Hashes are used to verify that data has not been altered during transmission or storage. If the hash value of received data matches the hash value originally computed, the data is likely intact.
- Password Storage: Hash functions are used to securely store passwords. Instead of storing plain text passwords, a system stores their hashes. During login, the system hashes the entered password and compares it to the stored hash.
- Cryptographic Signatures: Hash functions are used in creating digital signatures, a way to verify the authenticity and integrity of a message or document.
3.2 Using Ruby’s Digest Library
Ruby provides a Digest library that allows you to easily calculate hash values using various algorithms, such as MD5, SHA-1, and SHA-256. Here’s a simple example using SHA-256:
ruby require 'digest' data = 'Hello, world!' sha256_hash = Digest::SHA256.hexdigest(data) puts "Data: #{data}" puts "SHA-256 Hash: #{sha256_hash}"
In this example, we use the Digest::SHA256 class to calculate the SHA-256 hash of the input data. The resulting hash value is a 64-character hexadecimal string.
3.3 Salting Your Hashes
When hashing passwords, it’s essential to add a salt to the input data before hashing it. A salt is a random value that is unique for each user. Adding a salt helps protect against dictionary attacks and rainbow table attacks.
Here’s how you can hash a password with a salt using Ruby’s Digest library:
ruby require 'securerandom' require 'digest' # Generate a random salt salt = SecureRandom.hex(16) # 128 bits of randomness # User's password password = 'my_secure_password' # Combine the password and salt salted_password = "#{password}#{salt}" # Hash the salted password hashed_password = Digest::SHA256.hexdigest(salted_password) puts "Salt: #{salt}" puts "Hashed Password: #{hashed_password}"
In this example, we use the SecureRandom module to generate a random salt. We then combine the user’s password and the salt before hashing it with SHA-256.
4. Digital Signatures with Ruby
Digital signatures are a cryptographic technique used to verify the authenticity and integrity of a message or document. They are commonly used in scenarios where it’s essential to prove that a message or document has not been altered and was indeed created by a specific entity.
4.1 Understanding Digital Signatures
Digital signatures involve two processes: signing and verifying.
- Signing: The sender uses their private key to create a digital signature for the message. This signature is unique to both the message and the sender’s private key.
- Verifying: The recipient uses the sender’s public key to verify the digital signature. If the verification succeeds, it confirms that the message was not altered during transit and was indeed sent by the sender.
4.2 Implementing Digital Signatures in Ruby
Ruby’s OpenSSL library makes it relatively straightforward to work with digital signatures. Here’s an example of how to sign and verify a message using RSA keys:
ruby require 'openssl' # Generate a key pair key_pair = OpenSSL::PKey::RSA.generate(2048) # Message to be signed message = 'This is a signed message.' # Sign the message with the private key signature = key_pair.sign(OpenSSL::Digest::SHA256.new, message) # Verify the signature with the public key verification = key_pair.verify(OpenSSL::Digest::SHA256.new, signature, message) puts "Message: #{message}" puts "Signature: #{signature.unpack('H*')[0]}" puts "Signature Verification: #{verification ? 'Valid' : 'Invalid'}"
In this example, we generate an RSA key pair, sign a message with the private key, and then verify the signature with the public key. If the verification is successful, the signature is considered valid.
4.3 Verifying Digital Signatures
Verifying digital signatures is a crucial step in ensuring the authenticity of received data. When working with digital signatures, you should always verify signatures using the sender’s public key to confirm that the data has not been tampered with and that it indeed originated from the expected source.
5. Securing Passwords
Securing user passwords is a critical aspect of application security. Storing passwords in plaintext is a significant security risk, as it exposes users to potential data breaches. Ruby provides tools and libraries to securely hash and manage passwords.
5.1 Storing Passwords Safely
When storing passwords, it’s essential to follow best practices:
- Use a Secure Hash Function: Always use a strong, cryptographically secure hash function like bcrypt or Argon2 to hash passwords. Avoid older and weaker hash functions like MD5 or SHA-1.
- Add a Salt: As mentioned earlier, adding a unique salt to each user’s password before hashing it prevents rainbow table attacks.
- Use Proper Key Derivation: Use key derivation functions (KDFs) designed for password hashing, such as bcrypt’s adaptive algorithm, which automatically adjusts the number of iterations to mitigate brute force attacks.
- Keep Password Hashes Secret: Store password hashes securely and ensure they are not accessible to unauthorized users.
5.2 Password Hashing in Ruby
Ruby provides the bcrypt gem, which is a popular choice for securely hashing passwords. Here’s how you can use it:
ruby require 'bcrypt' # User's password password = 'my_secure_password' # Generate a salt and hash the password salt = BCrypt::Engine.generate_salt hashed_password = BCrypt::Engine.hash_secret(password, salt) puts "Salt: #{salt}" puts "Hashed Password: #{hashed_password}"
In this example, we use the BCrypt::Engine to generate a salt and hash the user’s password. BCrypt automatically handles salting and uses a slow hashing algorithm to make brute force attacks more challenging.
6. Best Practices for Ruby Cryptography
While Ruby provides robust tools for implementing cryptography and enhancing security, it’s essential to follow best practices to ensure the effectiveness of your security measures.
6.1 Keep Libraries Up-to-Date
Regularly update your Ruby gems and libraries, especially those related to cryptography and security. Developers often release updates to address vulnerabilities and improve security.
6.2 Managing Keys Securely
Protect your cryptographic keys. Store them in a secure location, use hardware security modules (HSMs) if necessary, and restrict access to authorized personnel only. Keys are the foundation of your cryptographic security.
6.3 Protecting Against Common Attacks
Be aware of common security threats such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Implement appropriate security measures to mitigate these risks alongside your cryptographic solutions.
Conclusion
In this guide, we’ve explored how to use Ruby functions for cryptography and security. From encryption and hashing to digital signatures and password protection, Ruby offers a comprehensive set of tools to enhance the security of your applications.
By understanding the basics of cryptography and following best practices, you can safeguard your data, protect your users, and ensure the integrity of your applications in an ever-evolving digital landscape. Ruby’s versatility and built-in cryptographic functions make it a powerful choice for developers looking to enhance their application’s security.
Start implementing these techniques in your Ruby projects today, and stay ahead of potential security threats. Your users and data deserve nothing less than the best protection available.
Table of Contents
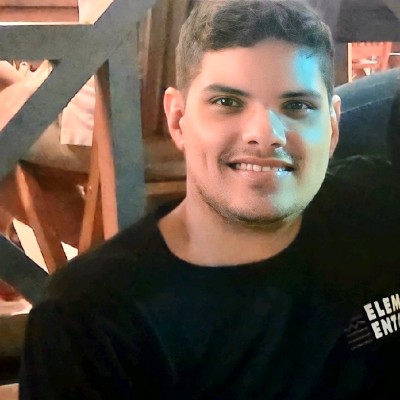
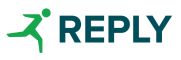