Data Extraction with Ruby CSV Parsing
CSV (Comma-Separated Values) files are a popular data interchange format used for storing tabular data. They are widely used for various purposes, such as data import/export, data analysis, and data exchange between different systems. When it comes to working with CSV files in Ruby, the language offers powerful functions and libraries that make parsing and generating CSVs a breeze.
In this comprehensive guide, we will delve into the world of CSV parsing and generation using Ruby functions. Whether you are a beginner or an experienced Ruby developer, this article will equip you with the knowledge and tools necessary to manipulate CSV files effectively. Let’s get started!
What is CSV?
CSV, or Comma-Separated Values, is a simple file format for storing tabular data. Each line in a CSV file represents a row of the table, with the values separated by commas (or other delimiters). CSV files can be opened and edited using a spreadsheet software like Microsoft Excel or Google Sheets. They provide a lightweight and universal way to exchange data between different systems.
Setting Up Your Environment
Before we dive into CSV parsing and generation with Ruby, let’s ensure that we have a suitable environment set up. Make sure you have Ruby installed on your machine, preferably the latest stable version. You can install Ruby by following the instructions provided on the official Ruby website.
Once Ruby is installed, you can check the version by opening a terminal or command prompt and running the following command:
ruby ruby -v
If you see the version information, you’re good to go!
CSV Parsing with Ruby Functions
1. Reading CSV Files
Ruby provides a convenient CSV module that makes reading and parsing CSV files a straightforward task. To begin, we need to require the CSV module in our code:
ruby require 'csv'
Now, let’s assume we have a CSV file named “data.csv” with the following content:
graphql Name,Email,Age John Doe,johndoe@example.com,25 Jane Smith,janesmith@example.com,30
To read this CSV file and access its data, we can use the CSV.foreach method:
ruby CSV.foreach('data.csv') do |row| # Access and process each row here end
The CSV.foreach method iterates over each row in the CSV file, providing us with an array representing each row. We can access the values in the row by index, like row[0], row[1], and so on.
2. Accessing CSV Data
Once we have the CSV data loaded into memory, we can access and manipulate the values as needed. Let’s enhance our previous example to print the name and email of each person in the CSV file:
ruby CSV.foreach('data.csv', headers: true) do |row| puts "Name: #{row['Name']}, Email: #{row['Email']}" end
By passing the headers: true option, we can access the values using their header names as keys in a hash-like structure. This way, the code becomes more readable and self-explanatory.
3. Manipulating CSV Data
In addition to reading and accessing CSV data, Ruby functions provide numerous methods for manipulating and transforming the CSV data. Let’s explore a few common scenarios:
3.1 Filtering CSV Rows:
Imagine we want to filter out all the rows where the age is greater than 25. We can achieve this by using the CSV.filter method:
ruby CSV.filter('data.csv', headers: true) do |row| row['Age'].to_i <= 25 end
The CSV.filter method applies the given block to each row and returns a new CSV object containing only the rows that match the condition specified in the block.
3.2 Sorting CSV Data:
To sort the CSV data based on a specific column, we can use the CSV.sort_by method. Let’s sort the CSV rows based on the “Name” column in ascending order:
ruby sorted_data = CSV.sort_by('data.csv', headers: true) { |row| row['Name'] }
The CSV.sort_by method takes a block that specifies the sorting criteria and returns a new CSV object with the rows sorted accordingly.
4. Error Handling
While parsing CSV files, it’s essential to handle potential errors gracefully. Ruby’s CSV functions provide mechanisms to handle common CSV parsing issues. For example, if a CSV file contains malformed data or non-UTF-8 characters, we can use exception handling to catch and handle such errors:
ruby begin CSV.foreach('data.csv', headers: true) do |row| # Process each row here end rescue CSV::MalformedCSVError => e puts "Error parsing CSV file: #{e.message}" end
By wrapping the CSV parsing code in a begin-rescue block, we can catch any CSV::MalformedCSVError exceptions that might occur and handle them appropriately.
CSV Generation with Ruby Functions
1. Creating CSV Files
Ruby’s CSV module not only helps with parsing CSV files but also allows us to generate CSV files from scratch. We can create a new CSV file by opening a file object with the appropriate write mode and using the CSV module to write data to it.
ruby CSV.open('new_data.csv', 'w') do |csv| # Write data to the CSV file End
In the example above, we create a new file named “new_data.csv” and open it in write mode (‘w’). The block provided to CSV.open allows us to write data to the file using the << operator or the CSV#<< method.
2. Writing Data to CSV
To write data to a CSV file, we can use the << operator or the CSV#<< method. Let’s assume we have an array of arrays representing the data we want to write to the CSV file:
ruby data = [ ['Name', 'Email', 'Age'], ['John Doe', 'johndoe@example.com', 25], ['Jane Smith', 'janesmith@example.com', 30] ]
We can write this data to a CSV file named “new_data.csv” using the following code:
ruby CSV.open('new_data.csv', 'w') do |csv| data.each do |row| csv << row end end
The csv << row statement writes each row of data to the CSV file. The resulting CSV file will contain the data in the specified tabular format.
3. Advanced CSV Generation Techniques
Ruby’s CSV module offers advanced techniques for customizing CSV generation. For instance, we can define custom headers, specify the column order, and control the formatting of the data. The CSV.open method accepts various options that allow us to modify the behavior of CSV generation.
ruby CSV.open('new_data.csv', 'w', write_headers: true, headers: ['Name', 'Email', 'Age'], col_sep: "\t") do |csv| csv << ['John Doe', 'johndoe@example.com', 25] csv << ['Jane Smith', 'janesmith@example.com', 30] end
In the example above, we enable writing headers using the write_headers: true option and provide custom headers using the headers option. We can also specify a different column separator using the col_sep option (in this case, a tab character).
Conclusion
In this guide, we explored the power of Ruby functions for CSV parsing and generation. We learned how to read and access CSV data using the CSV module’s methods. Additionally, we discovered various techniques for manipulating CSV data, handling errors, and generating CSV files from scratch.
By leveraging the capabilities of Ruby functions, you can efficiently work with CSV files, automate data processing tasks, and seamlessly exchange data between different systems. Armed with this knowledge, you can unlock the full potential of Ruby for handling CSV data and elevate your data manipulation skills to new heights.
Start experimenting with CSV parsing and generation in Ruby, and witness the simplicity and elegance of this versatile programming language. Happy coding!
Table of Contents
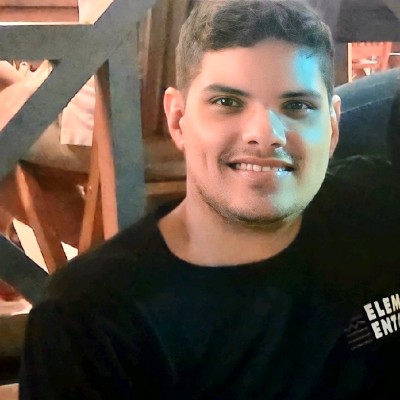
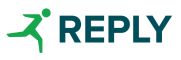