How to Use Ruby Functions for Data Scraping and Crawling
In the ever-expanding digital landscape, data is king. Whether you’re a business looking to gather competitive intelligence or a researcher in need of a vast dataset, web scraping and crawling are essential skills. In this guide, we’ll delve into the world of data scraping and crawling using Ruby, a versatile and dynamic programming language. You’ll learn how to harness Ruby functions to efficiently collect, process, and store data from websites. So, let’s embark on this data-driven journey and unlock the potential of Ruby for web scraping.
Table of Contents
1. What is Data Scraping and Crawling?
1.1. Understanding the Basics
Data scraping and crawling are techniques used to extract data from websites and web pages. These techniques have numerous applications, from collecting market data for business analysis to scraping content for research purposes. Let’s briefly differentiate between these two terms:
- Data Scraping: Involves extracting specific data or information from web pages. For example, scraping product details from an e-commerce website.
- Data Crawling: Involves systematically browsing and indexing web pages across a website or multiple websites. It’s often used by search engines to index web content.
1.2. Legal and Ethical Considerations
Before diving into web scraping and crawling, it’s crucial to understand the legal and ethical aspects:
- Respect Terms of Service: Always review a website’s terms of service or use policy. Some websites explicitly prohibit scraping, and violating these terms can lead to legal consequences.
- Respect Robots.txt: Websites may use a robots.txt file to indicate which parts of their site are off-limits to web crawlers. Always honor the directives specified in this file.
- Rate Limiting: Web scraping can put a strain on a website’s server. Implement rate limiting to avoid overwhelming the server and getting banned.
Now that we have a foundational understanding, let’s explore how Ruby can help us in this data extraction journey.
2. Setting Up Your Environment
2.1. Installing Ruby
The first step in your web scraping adventure with Ruby is to install the language itself. You can download Ruby from the official website (https://www.ruby-lang.org/). Once Ruby is installed, you’ll have access to its powerful standard library and an active community of developers.
2.2. Installing Necessary Gems
Gems are packages or libraries in the Ruby ecosystem that extend its functionality. Two essential gems for web scraping are net/http and nokogiri.
2.3. Using Bundler
Bundler is a dependency manager for Ruby projects that simplifies gem installation. Create a Gemfile in your project directory and add the following:
ruby source 'https://rubygems.org' gem 'nokogiri' gem 'httparty'
Then, run bundle install to install these gems along with their dependencies.
2.4. Choosing the Right Development Tools
For web scraping, choosing the right development tools is crucial. You can use any text editor or integrated development environment (IDE) for writing Ruby code. Popular choices include Visual Studio Code, Sublime Text, and RubyMine. Additionally, consider using version control systems like Git to track your project’s changes.
Now that we have our environment set up, let’s move on to making HTTP requests using Ruby.
3. Making HTTP Requests with Ruby
3.1. Using Net::HTTP
Ruby’s standard library includes the net/http module, which allows you to make HTTP requests easily. Here’s a simple example of how to make a GET request to a website:
ruby require 'net/http' url = URI.parse('https://example.com') http = Net::HTTP.new(url.host, url.port) request = Net::HTTP::Get.new(url) response = http.request(request) puts response.body
In this code snippet:
- We require the net/http library.
- We parse the URL we want to fetch.
- We create an HTTP object.
- We create an HTTP GET request.
- We send the request and store the response in the response variable.
- Finally, we print the response body.
This is a basic example, but it demonstrates how to make HTTP requests using Ruby’s standard library. However, when it comes to web scraping, you’ll often need more advanced functionality, such as handling cookies, sessions, and managing redirects. This is where third-party libraries like HTTParty come in handy.
3.2. Leveraging Third-Party Libraries (e.g., HTTParty)
HTTParty is a popular gem for making HTTP requests in Ruby. It simplifies the process and provides a more intuitive API for working with web services. Here’s an example of how to use HTTParty to make a GET request:
ruby require 'httparty' response = HTTParty.get('https://example.com') puts response.body
HTTParty also provides options for handling JSON, parsing XML, and handling various authentication methods. It’s a versatile choice for web scraping projects.
With the ability to make HTTP requests in place, we can move on to the next step: parsing HTML with Nokogiri.
4. Parsing HTML with Nokogiri
4.1. Installing Nokogiri
Nokogiri is a powerful and popular gem for parsing HTML and XML documents in Ruby. To install it, add it to your Gemfile as mentioned earlier and run bundle install. Once installed, you can start using Nokogiri to navigate and extract data from HTML documents.
4.2. Selecting and Extracting Data
Nokogiri allows you to select and extract data from HTML documents using CSS or XPath selectors. Here’s an example of extracting all the links from a webpage:
ruby require 'nokogiri' require 'open-uri' url = 'https://example.com' html = URI.open(url) doc = Nokogiri::HTML(html) # Extract all links using CSS selector links = doc.css('a') # Print the href attribute of each link links.each do |link| puts link['href'] end
In this code:
- We require the nokogiri and open-uri libraries.
- We open the URL and read its content.
- We parse the HTML content with Nokogiri.
- We select all <a> elements using the CSS selector a.
- We iterate over the links and print their href attributes.
- Nokogiri’s ability to parse and traverse the Document Object Model (DOM) simplifies data extraction from HTML documents.
4.3. Navigating the Document Object Model (DOM)
Nokogiri provides various methods for navigating and manipulating the DOM. Here are some commonly used methods:
- css: Select elements using CSS selectors.
- xpath: Select elements using XPath expressions.
- at_css and at_xpath: Select the first matching element.
- text: Get the text content of an element.
- attr: Get the value of an attribute.
These methods, combined with your knowledge of CSS or XPath, empower you to extract specific data from web pages efficiently.
With data extraction under control, let’s explore how to handle the collected data and store the results.
5. Handling Data and Storing Results
Data scraping often involves collecting large amounts of unstructured data. To make this data useful, you’ll need to clean it and store it in an organized manner. Here are some key steps to consider:
5.1. Data Cleaning and Transformation
Data from websites can be messy and inconsistent. Before storing it, consider applying data cleaning and transformation techniques. Ruby’s string manipulation functions, regular expressions, and libraries like StringScanner can be invaluable for cleaning and formatting data.
5.2. Storing Data (e.g., CSV, JSON, Databases)
Once your data is cleaned and structured, you’ll want to store it for further analysis or use. Common data storage options include:
- CSV (Comma-Separated Values): Ideal for tabular data.
- JSON (JavaScript Object Notation): A lightweight and human-readable format suitable for various data types.
- Databases: Use databases like PostgreSQL, MySQL, or SQLite to store structured data efficiently.
Here’s an example of how to store scraped data in a CSV file using Ruby’s CSV library:
ruby require 'csv' # Sample data data = [ ['Name', 'Age'], ['Alice', 25], ['Bob', 30] ] # Save data to a CSV file CSV.open('data.csv', 'w') do |csv| data.each do |row| csv << row end end
This code creates a CSV file and writes data to it.
Now that we know how to handle data, let’s tackle more advanced web scraping challenges.
6. Managing Pagination and Infinite Scrolling
6.1. Pagination Strategies
Many websites divide content across multiple pages. To scrape all the data, you’ll need to implement a pagination strategy. This typically involves:
- Identifying the pagination elements (e.g., “Next” buttons).
- Constructing URLs for each page.
- Iterating through the pages to scrape data.
Here’s a simplified example of how to scrape data from multiple pages with pagination:
ruby require 'nokogiri' require 'open-uri' # Base URL with pagination base_url = 'https://example.com/page/' # Iterate through multiple pages (1..5).each do |page_number| url = "#{base_url}#{page_number}" html = URI.open(url) doc = Nokogiri::HTML(html) # Extract and process data from the current page # ... end
This code iterates through pages by changing the page number in the URL and scraping data from each page.
6.2. Dealing with Infinite Scrolling
Some websites use infinite scrolling to load content dynamically as the user scrolls down. To scrape such websites, you’ll need to automate scrolling and retrieve data as it becomes available. Tools like Selenium WebDriver can help automate browser actions for this purpose.
Managing pagination and infinite scrolling effectively is essential for comprehensive web scraping.
7. Handling Authentication and Cookies
7.1. Logging in with Ruby
If the website you’re scraping requires user authentication, you can use Ruby to simulate the login process. Here’s a simplified example using the httparty gem:
ruby require 'httparty' # Define login credentials username = 'your_username' password = 'your_password' # Create a session session = HTTParty.post('https://example.com/login', body: { username: username, password: password }) # Use the session to make authenticated requests response = session.get('https://example.com/secure-page') puts response.body
In this example:
- We send a POST request to the login page with the username and password.
- We store the session information.
- We use the session to make authenticated requests to protected pages.
7.2. Managing Cookies
Some websites use cookies to track sessions. You can handle cookies in Ruby using libraries like http-cookie. Here’s a basic example of storing and sending cookies:
ruby require 'http-cookie' require 'net/http' # Create a cookie jar cookie_jar = HTTP::CookieJar.new # Add a cookie to the jar cookie = HTTP::Cookie.new('session_id', '12345', domain: 'example.com', path: '/') cookie_jar.add(cookie) # Send a request with the cookie url = URI.parse('https://example.com') http = Net::HTTP.new(url.host, url.port) request = Net::HTTP::Get.new(url) request['Cookie'] = HTTP::Cookie.cookie_value(cookie_jar.cookies(url)) response = http.request(request) puts response.body
This code creates a cookie jar, adds a cookie, and sends a GET request with the cookie attached.
8. Error Handling and Robustness
Web scraping often involves dealing with unpredictable factors like network issues, changes in website structure, or unexpected data formats. Implementing error handling and robustness measures is crucial for the reliability of your scraper.
8.1. Handling Different Response Codes
HTTP responses can have various status codes (e.g., 200 for success, 404 for not found, 503 for service unavailable). Implement error handling to deal with different response codes gracefully. For example:
ruby require 'net/http' begin url = URI.parse('https://example.com') http = Net::HTTP.new(url.host, url.port) request = Net::HTTP::Get.new(url) response = http.request(request) if response.code == '200' # Process the data else puts "HTTP Error: #{response.code}" end rescue StandardError => e puts "Error: #{e.message}" end
8.2. Implementing Retry Mechanisms
To handle transient errors, consider implementing retry mechanisms. If a request fails, you can wait for a brief period and then retry. Libraries like retryable can simplify this process:
ruby require 'net/http' require 'retryable' Retryable.retryable(tries: 3, sleep: 2) do begin url = URI.parse('https://example.com') http = Net::HTTP.new(url.host, url.port) request = Net::HTTP::Get.new(url) response = http.request(request) if response.code == '200' # Process the data else puts "HTTP Error: #{response.code}" raise StandardError, "HTTP Error: #{response.code}" end rescue StandardError => e puts "Error: #{e.message}" raise e end end
In this code, the request will be retried up to three times with a two-second delay between attempts.
9. Respecting Robots.txt and Rate Limiting
9.1. Understanding Robots.txt
Robots.txt is a standard used by websites to communicate with web crawlers. It tells crawlers which parts of a site can be crawled and which should be avoided. It’s crucial to respect robots.txt to maintain a good relationship with website owners.
Before scraping a website, check its robots.txt file to see if there are any restrictions on crawling. You can do this manually by visiting https://example.com/robots.txt, or you can programmatically retrieve and parse it.
9.2. Implementing Rate Limiting
Rate limiting is a strategy to prevent overloading a website’s server with too many requests. It’s essential for responsible web scraping. Here’s a simple example of how to implement rate limiting in Ruby:
ruby require 'net/http' require 'timeout' # Define the rate limit (requests per second) rate_limit = 2 # List of URLs to scrape urls = [ 'https://example.com/page1', 'https://example.com/page2', # Add more URLs here ] # Iterate through the URLs urls.each do |url| start_time = Time.now begin response = Net::HTTP.get_response(URI(url)) # Process the response rescue StandardError => e puts "Error: #{e.message}" ensure elapsed_time = Time.now - start_time sleep_time = 1.0 / rate_limit - elapsed_time sleep(sleep_time) if sleep_time > 0 end end
In this code:
- We define the rate limit as two requests per second.
- We iterate through a list of URLs, making requests.
- After each request, we calculate the elapsed time and sleep to respect the rate limit.
Conclusion
In this comprehensive guide, we’ve explored how to use Ruby functions for data scraping and crawling. From making HTTP requests to parsing HTML with Nokogiri, handling data, managing pagination, dealing with authentication, and implementing error handling and rate limiting, you now have a solid foundation for web scraping with Ruby.
Remember to always respect the legality and ethics of web scraping, adhere to website policies, and be a responsible scraper. As you continue your web scraping journey, you’ll encounter various challenges and opportunities to refine your skills. Happy scraping!
Now that you’ve learned how to use Ruby functions for data scraping and crawling, it’s time to put your knowledge into practice and start extracting valuable data from the web.
Table of Contents
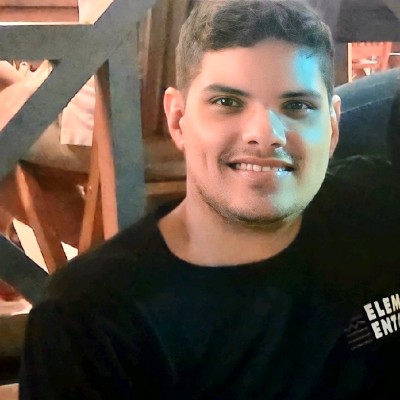
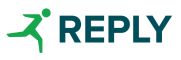