How to Use Ruby Functions for Fraud Detection and Prevention in Financial Transactions
In the realm of financial transactions, fraud detection and prevention are critical for safeguarding assets and maintaining trust. Ruby, a versatile programming language, offers a variety of functions and libraries that can help in detecting and preventing fraudulent activities. This blog explores how to use Ruby functions to implement robust fraud detection mechanisms, providing practical examples and discussing key considerations for secure financial transactions.
Introduction to Fraud Detection in Financial Transactions
Fraud detection involves identifying and preventing unauthorized or suspicious activities in financial systems. These can include fraudulent transactions, identity theft, money laundering, and more. Implementing effective fraud detection requires a combination of statistical analysis, machine learning, and domain-specific knowledge.
Key Ruby Libraries for Fraud Detection
Several Ruby libraries can assist in fraud detection, offering tools for data analysis, machine learning, and anomaly detection.
1.Ruby’s Standard Library
Ruby’s standard library includes several useful modules, such as `Date`, `Time`, and `Digest`, which can be leveraged for basic fraud detection tasks.
- SciRuby and NMatrix
SciRuby is a suite of libraries for scientific computing in Ruby. NMatrix, part of SciRuby, provides efficient matrix and linear algebra operations, useful for data analysis and machine learning.
- Rails
Ruby on Rails, while primarily a web application framework, offers powerful tools for managing databases and implementing business logic, making it suitable for developing comprehensive fraud detection systems.
Implementing Fraud Detection with Ruby
Let’s explore some practical examples of using Ruby for fraud detection.
- Example 1 Simple Anomaly Detection with Transaction Amounts
A common fraud detection technique is anomaly detection, where transactions that deviate significantly from normal patterns are flagged for review.
```ruby Example data transactions = [50, 100, 150, 120, 180, 2500, 200, 220] Define threshold for anomaly threshold = 1000 Detect anomalies anomalies = transactions.select { |amount| amount > threshold } puts "Anomalous transactions {anomalies}" ```
In this example, transactions exceeding a certain threshold are considered anomalous and potentially fraudulent. This basic method can be enhanced with more sophisticated statistical techniques or machine learning models.
- Example 2 Using Digest for Secure Data Storage
For secure storage and verification, sensitive data such as credit card numbers should be hashed. Ruby’s `Digest` module can be used for this purpose.
```ruby require 'digest' Hash sensitive data def hash_data(data) DigestSHA256.hexdigest(data) end credit_card_number = "4111111111111111" hashed_cc = hash_data(credit_card_number) puts "Hashed Credit Card {hashed_cc}" ```
This example demonstrates how to hash a credit card number for secure storage. Hashing ensures that sensitive information is not stored in plaintext, reducing the risk of data breaches.
Advanced Techniques Machine Learning with Ruby
For more advanced fraud detection, machine learning models can be employed. Ruby’s integration with machine learning frameworks like TensorFlow (via the `tensorflow` gem) allows for the development of sophisticated models.
- Example 3 Machine Learning for Fraud Detection
While Python is more commonly used for machine learning, Ruby can also be used to train and deploy models.
```ruby require 'nmatrix' Example dataset data = NMatrix.new([4, 2], [1, 1, 1, 0, 0, 1, 0, 0]) labels = NMatrix.new([4, 1], [1, 1, 0, 0]) Simple linear classifier (pseudo-code) weights = NMatrix.new([2, 1], [0.1, 0.2]) predictions = data.dot(weights) puts "Predictions {predictions.to_a}" ```
In this pseudo-code example, we use a simple linear classifier to predict labels based on input features. In practice, more complex models like neural networks or gradient boosting might be employed for higher accuracy.
Best Practices for Fraud Detection and Prevention
- Data Encryption Ensure all sensitive data is encrypted both in transit and at rest.
- Regular Monitoring Continuously monitor transactions and system logs for unusual activity.
- Machine Learning Models Use machine learning models to detect complex patterns and anomalies.
- Multi-Factor Authentication Implement multi-factor authentication to enhance security.
- Regular Audits Conduct regular security audits and vulnerability assessments.
Conclusion
Ruby provides a rich set of tools and libraries for implementing fraud detection and prevention in financial transactions. By leveraging these tools, developers can create secure systems that protect against fraudulent activities. From basic anomaly detection to advanced machine learning techniques, Ruby offers flexible solutions to enhance security in financial applications.
Further Reading
Table of Contents
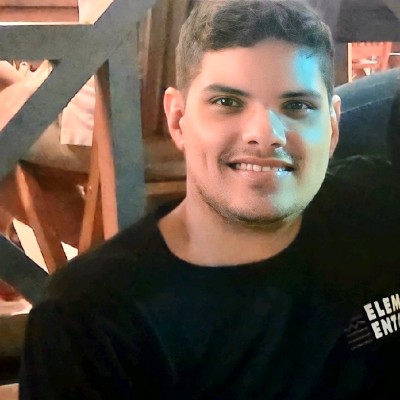
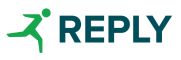