How to Use Ruby Functions for Geocoding and Mapping
In today’s digital age, location-based services have become an integral part of numerous applications, ranging from e-commerce and social networking to navigation and local search. One of the fundamental tasks in building such applications is geocoding, the process of converting addresses or place names into geographic coordinates (latitude and longitude). Additionally, mapping functionality is crucial for visualizing and utilizing this geographical data effectively. Ruby, a versatile and expressive programming language, offers a wealth of tools and libraries for geocoding and mapping. In this comprehensive guide, we will explore how to use Ruby functions to master geocoding and mapping in your projects.
Table of Contents
1. Understanding Geocoding
1.1. What is Geocoding?
Geocoding is the process of converting human-readable addresses, such as “123 Main Street, Cityville,” into geographic coordinates, specifically latitude and longitude. These coordinates pinpoint the exact location of a place on the Earth’s surface. Geocoding plays a vital role in location-based applications, enabling developers to map addresses, find nearby points of interest, and calculate distances between locations.
1.2. Why is Geocoding Important?
Geocoding is essential for various reasons:
- Location Services: It powers location-based services like ride-sharing apps (Uber, Lyft), food delivery services (Uber Eats, DoorDash), and map applications (Google Maps, Apple Maps).
- Search Functionality: Geocoding allows users to search for places based on their addresses or names. It enhances search accuracy and relevance.
- Route Planning: It assists in calculating the shortest or fastest route between two points, taking traffic conditions into account.
- Spatial Analytics: Geocoding is crucial for analyzing spatial data, such as demographics, market trends, and customer locations.
Now that we’ve established the significance of geocoding, let’s dive into using Ruby to perform this task efficiently.
2. Setting Up Your Environment
Before we start geocoding and mapping with Ruby, you need to set up your development environment. This includes installing Ruby and choosing a geocoding service to provide the necessary data.
2.1. Installing Ruby
If you haven’t already, install Ruby on your system. You can download the latest version from the official website (https://www.ruby-lang.org/en/downloads/) or use a version manager like RVM (Ruby Version Manager) or rbenv for better control over Ruby versions.
To check if Ruby is installed, open your terminal and run:
bash ruby -v
You should see the installed Ruby version displayed.
2.2. Choosing a Geocoding Service
To perform geocoding, you’ll need access to a geocoding service that can convert addresses into coordinates. There are several popular geocoding services available, each with its own API and pricing model. Some well-known options include:
- Google Maps Geocoding API: Offers precise geocoding and reverse geocoding capabilities. Requires an API key.
- OpenStreetMap Nominatim API: Provides open-source geocoding data but has usage limits.
- Mapbox Geocoding API: Offers geocoding and geospatial capabilities with a free tier.
For this tutorial, we’ll use the Geocoder gem, which supports multiple geocoding providers and simplifies the integration process.
3. Geocoding with Ruby
3.1. Geocoder Gem
The Geocoder gem is a popular Ruby library that simplifies geocoding and reverse geocoding tasks. It supports various geocoding providers, including Google Maps, OpenStreetMap, and Mapbox. To get started, you’ll need to add the Geocoder gem to your Ruby project’s Gemfile and run bundle install.
Here’s how you can add the Geocoder gem to your Gemfile:
ruby # Gemfile gem 'geocoder'
Now, let’s explore a basic geocoding example using the Geocoder gem.
3.2. Basic Geocoding Example
Suppose you have an address, and you want to obtain its coordinates. You can achieve this with the Geocoder gem as follows:
ruby require 'geocoder' address = '1600 Amphitheatre Parkway, Mountain View, CA' coordinates = Geocoder.coordinates(address) if coordinates latitude, longitude = coordinates puts "Latitude: #{latitude}" puts "Longitude: #{longitude}" else puts "Geocoding failed for the address: #{address}" end
In this code snippet:
- We require the Geocoder library.
- We define an address as a string.
- We use Geocoder.coordinates(address) to obtain the latitude and longitude for the given address.
- We check if the geocoding was successful and print the coordinates if it was.
3.3. Handling Errors
It’s essential to handle errors when performing geocoding. Geocoding services may occasionally return errors due to invalid addresses or rate limits. Here’s how you can handle errors gracefully:
ruby require 'geocoder' address = 'Invalid Address' coordinates = Geocoder.coordinates(address) if coordinates latitude, longitude = coordinates puts "Latitude: #{latitude}" puts "Longitude: #{longitude}" else error_message = Geocoder::Lookup.get(:google).send(:result, Geocoder::Query.new(address)) puts "Geocoding failed with error: #{error_message}" end
In this updated code:
- We use an intentionally invalid address to simulate a geocoding failure.
- We capture the error message using the Geocoder gem’s error handling mechanism.
Now that you’ve learned how to perform geocoding with Ruby using the Geocoder gem, let’s move on to mapping with Ruby.
4. Mapping with Ruby
Mapping is the visual representation of geographic data. It enables users to interact with location-based information effectively. For mapping in Ruby, we’ll leverage the Leaflet.js library, a powerful JavaScript library for creating interactive maps.
4.1. Leaflet.js: A JavaScript Library
Leaflet.js is a widely used open-source JavaScript library for interactive maps. It is lightweight, highly customizable, and easy to integrate into web applications. Leaflet.js provides various features, including map rendering, markers, popups, and support for different tile providers.
To use Leaflet.js in your Ruby project, you’ll need to include the Leaflet library in your HTML file and set up a container div to hold the map. Here’s a basic example:
html <!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css" /> <script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"></script> </head> <body> <div id="map" style="height: 400px;"></div> <script> var map = L.map('map').setView([51.505, -0.09], 13); L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png').addTo(map); </script> </body> </html>
In this HTML example:
- We include the Leaflet CSS and JavaScript files from a content delivery network (CDN).
- We create a div element with the ID “map” to serve as the map container.
- We initialize a Leaflet map and set its initial view to a specific latitude and longitude.
- We add a tile layer from OpenStreetMap to render the map.
4.2. Integrating Leaflet.js with Ruby
To integrate Leaflet.js into your Ruby application, you can create a Ruby template (e.g., using ERB) that generates the necessary HTML and JavaScript code. Here’s a simplified example using Sinatra, a lightweight Ruby web framework:
ruby require 'sinatra' get '/' do erb :index end
And the corresponding views/index.erb template:
erb <!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css" /> <script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"></script> </head> <body> <div id="map" style="height: 400px;"></div> <script> var map = L.map('map').setView([51.505, -0.09], 13); L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png').addTo(map); </script> </body> </html>
In this example, we use Sinatra to serve the HTML template. You can adapt this approach to your Ruby web framework of choice.
4.3. Creating Interactive Maps
Leaflet.js allows you to create interactive maps with various features, including markers, popups, and custom overlays. Here’s an example of adding a marker to the map:
html <script> var map = L.map('map').setView([51.505, -0.09], 13); L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png').addTo(map); var marker = L.marker([51.505, -0.09]).addTo(map); marker.bindPopup('Hello, Leaflet!').openPopup(); </script>
In this code snippet:
- We add a marker at a specific latitude and longitude.
- We bind a popup to the marker, which displays a message when clicked.
With Leaflet.js, you can create highly interactive maps for your Ruby web applications, enhancing the user experience.
5. Real-World Applications
Now that you have a grasp of geocoding and mapping in Ruby, let’s explore real-world applications that leverage these capabilities.
5.1. Building a Location-Based Web App
Imagine you want to develop a location-based web application that helps users find nearby restaurants. You can use geocoding to convert user-entered addresses into coordinates and then use those coordinates to search for restaurants within a certain radius. With Leaflet.js, you can display the restaurants on a map, allowing users to visualize their locations.
Here’s a simplified example of how you might structure this application:
- Frontend: Collects user addresses, sends them to the server.
- Server: Receives user addresses, performs geocoding, and queries a restaurant database to find nearby restaurants.
- Frontend: Displays the restaurants on a Leaflet.js map.
This application would offer users a convenient way to discover restaurants in their vicinity.
5.2. Geofencing and Proximity Alerts
Geofencing involves defining virtual boundaries around real-world geographical areas. You can use geofencing in Ruby applications to trigger actions when a device or user enters or exits a geofenced area. For instance, a retail app can send a notification to a user when they approach a nearby store.
Here’s a high-level overview of implementing geofencing with Ruby:
- Define Geofences: Create geofence areas with specific coordinates and radiuses.
- Track User Locations: Continuously track the user’s location using geolocation services.
- Check for Geofence Crossing: Monitor the user’s position and trigger actions when they enter or exit a geofenced area.
This functionality can be achieved using Ruby frameworks, such as Ruby on Rails, along with geolocation libraries and databases for storing geofence data.
6. Best Practices
Before concluding, let’s discuss some best practices for geocoding and mapping in Ruby:
6.1. Geocoding Accuracy
- Use Reliable Geocoding Services: Choose a reputable geocoding service provider to ensure accurate and up-to-date location data.
- Handle Ambiguities: Some addresses may have multiple valid interpretations. Implement logic to handle ambiguities gracefully.
- Rate Limiting: Be aware of rate limits imposed by geocoding services and implement appropriate rate-limiting strategies in your code.
6.2. Performance Optimization
- Caching: Implement caching mechanisms to reduce the number of geocoding requests. Store previously geocoded addresses and reuse the results when possible.
- Batch Geocoding: If you need to geocode multiple addresses, consider using batch geocoding to minimize API calls.
- Client-Side Rendering: For interactive maps, offload rendering to the client-side whenever possible to reduce server load and improve responsiveness.
Conclusion
In this guide, we’ve explored how to harness the power of Ruby functions for geocoding and mapping. You’ve learned the significance of geocoding, set up your development environment, and used the Geocoder gem to perform geocoding in Ruby. Additionally, we’ve integrated Leaflet.js to create interactive maps and discussed real-world applications.
With this knowledge, you can build location-based features in your Ruby applications, whether you’re developing a local search tool, a delivery service, or a geofencing system. By following best practices for geocoding accuracy and performance optimization, you can create robust and efficient location-aware applications that delight your users and provide valuable location-based services.
Table of Contents
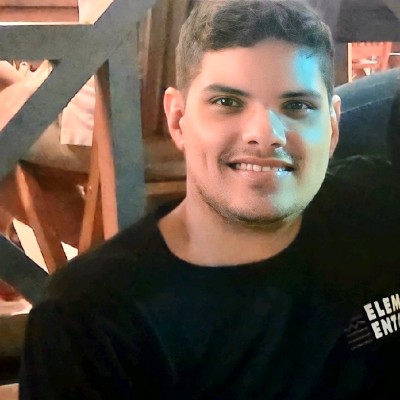
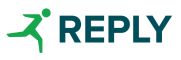