How to Use Ruby Functions for Image Manipulation
Image manipulation is an essential aspect of modern web development and graphic design. Whether you’re building a website, creating digital art, or optimizing images for better user experience, having a solid understanding of image manipulation can significantly enhance your skills. Ruby, a powerful and flexible programming language, offers a range of functions and libraries that make image manipulation a breeze. In this blog, we’ll explore how to use Ruby functions for image manipulation, from basic operations to advanced techniques, accompanied by code samples for hands-on learning.
Table of Contents
1. Understanding Image Manipulation:
1.1. What is Image Manipulation?
Image manipulation refers to the process of altering digital images to achieve desired visual effects or improvements. It involves various operations, such as resizing, cropping, applying filters, adding text, and more. Image manipulation is widely used in web development, graphic design, digital art, and photo editing. It enables developers and designers to create visually appealing content, optimize images for faster loading, and enhance the overall user experience.
1.2. Why Use Ruby for Image Manipulation?
Ruby is a versatile and user-friendly programming language known for its simplicity and readability. It offers several libraries and gems specifically designed for image manipulation, making it an excellent choice for developers and designers working on Ruby-based projects. The ease of integration with popular image processing libraries like ImageMagick and RMagick, as well as its extensive community support, makes Ruby a powerful tool for image manipulation tasks.
2. Getting Started with Ruby for Image Manipulation:
2.1. Installing Ruby and Required Libraries:
Before diving into image manipulation with Ruby, ensure you have Ruby installed on your system. You can download the latest version from the official Ruby website (https://www.ruby-lang.org). Additionally, you’ll need the appropriate libraries to perform image processing tasks efficiently. Two popular choices are RMagick and MiniMagick, which provide extensive functionality and are easy to use.
To install RMagick, use the following command:
ruby gem install rmagick
For MiniMagick, use:
ruby gem install minimagick
2.2. Loading and Saving Images:
Once you have Ruby and the required libraries installed, you can start loading and saving images. Both RMagick and MiniMagick allow you to read images from files or URLs and save them in different formats.
Using RMagick:
ruby require 'rmagick' # Load an image image = Magick::Image.read('input.jpg').first # Save the image in a different format image.write('output.png')
Using MiniMagick:
ruby require 'mini_magick' # Load an image image = MiniMagick::Image.open('input.jpg') # Save the image in a different format image.format('png') image.write('output.png')
3. Basic Image Operations with Ruby Functions:
3.1. Resizing and Scaling Images:
One of the most common image manipulation tasks is resizing or scaling images. It is crucial for creating thumbnails, adjusting image dimensions, and optimizing images for various display devices.
Using RMagick:
ruby require 'rmagick' image = Magick::Image.read('input.jpg').first # Resize the image to a specific width and height resized_image = image.resize_to_fill(300, 200) resized_image.write('resized_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' image = MiniMagick::Image.open('input.jpg') # Resize the image to a specific width and height image.resize '300x200' image.write('resized_output.jpg')
3.2. Cropping and Rotating Images:
Cropping allows you to remove unwanted parts of an image, while rotation enables you to change the image’s orientation.
Using RMagick:
ruby require 'rmagick' image = Magick::Image.read('input.jpg').first # Crop the image starting from the top-left corner, width, and height cropped_image = image.crop(50, 50, 200, 150) cropped_image.write('cropped_output.jpg') # Rotate the image by a specified angle (in degrees) rotated_image = image.rotate(45) rotated_image.write('rotated_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' image = MiniMagick::Image.open('input.jpg') # Crop the image starting from the top-left corner, width, and height image.crop '200x150+50+50' image.write('cropped_output.jpg') # Rotate the image by a specified angle (in degrees) image.rotate '45' image.write('rotated_output.jpg')
4. Flipping and Mirroring Images:
Flipping and mirroring are useful techniques to create symmetrical or mirror-like effects in images.
Using RMagick:
ruby require 'rmagick' image = Magick::Image.read('input.jpg').first # Flip the image horizontally flipped_image = image.flip flipped_image.write('flipped_output.jpg') # Mirror the image vertically mirrored_image = image.mirror mirrored_image.write('mirrored_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' image = MiniMagick::Image.open('input.jpg') # Flip the image horizontally image.flip image.write('flipped_output.jpg') # Mirror the image vertically image.flop image.write('mirrored_output.jpg')
5. Applying Filters and Effects to Images:
5.1. Adding Text and Watermarks:
Adding text or watermarks to images is a common practice for branding and copyright protection.
Using RMagick:
ruby require 'rmagick' image = Magick::Image.read('input.jpg').first # Annotate the image with text annotated_image = image.annotate('My Text', 0, 0, 0, 0, 'Arial') do self.fill = 'white' self.stroke = 'black' self.stroke_width = 2 self.pointsize = 36 self.gravity = Magick::CenterGravity end # Add a watermark image to the bottom-right corner watermark = Magick::Image.read('watermark.png').first watermarked_image = image.composite(watermark, Magick::SouthEastGravity, Magick::OverCompositeOp) watermarked_image.write('annotated_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' image = MiniMagick::Image.open('input.jpg') # Annotate the image with text image.combine_options do |c| c.gravity 'Center' c.pointsize 36 c.draw "text 0,0 'My Text'" c.fill 'white' c.stroke 'black' c.strokewidth 2 end # Add a watermark image to the bottom-right corner image.composite(MiniMagick::Image.open('watermark.png'), 'output.jpg') do |c| c.gravity 'SouthEast' end
5.2. Applying Color Filters:
Color filters can significantly alter the appearance of an image by changing its color balance or adding visual effects.
Using RMagick:
ruby require 'rmagick' image = Magick::Image.read('input.jpg').first # Apply a grayscale filter grayscale_image = image.quantize(256, Magick::GRAYColorspace) grayscale_image.write('grayscale_output.jpg') # Apply a sepia filter sepia_image = image.colorize(90, 50, 30, 'yellow') sepia_image.write('sepia_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' image = MiniMagick::Image.open('input.jpg') # Apply a grayscale filter image.colorspace 'Gray' image.write('grayscale_output.jpg') # Apply a sepia filter image.modulate '90,50,30' image.write('sepia_output.jpg')
5.3. Creating Image Thumbnails:
Thumbnails are smaller versions of images used for previews and faster loading.
Using RMagick:
ruby require 'rmagick' image = Magick::Image.read('input.jpg').first # Create a thumbnail with specific dimensions thumbnail_image = image.thumbnail(100, 100) thumbnail_image.write('thumbnail_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' image = MiniMagick::Image.open('input.jpg') # Create a thumbnail with specific dimensions image.resize '100x100' image.write('thumbnail_output.jpg')
5. Advanced Image Manipulation Techniques:
5.1. Image Blending and Compositing:
Image blending and compositing involve combining multiple images to create unique visual effects.
Using RMagick:
ruby require 'rmagick' # Load two images to blend image1 = Magick::Image.read('image1.jpg').first image2 = Magick::Image.read('image2.jpg').first # Blend the two images with a specific alpha value (0.0 - 1.0) blended_image = image1.composite(image2, 0, 0, Magick::OverCompositeOp) blended_image.write('blended_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' # Open two images to blend image1 = MiniMagick::Image.open('image1.jpg') image2 = MiniMagick::Image.open('image2.jpg') # Blend the two images with a specific alpha value (0 - 100) image1.composite(image2) do |c| c.compose 'Over' c.alpha '50%' end image1.write('blended_output.jpg')
5.2. Extracting Image Channels:
You can extract individual color channels from an image, such as the red, green, or blue channel.
Using RMagick:
ruby require 'rmagick' image = Magick::Image.read('input.jpg').first # Extract the red channel of the image red_channel = image.channel(Magick::RedChannel) red_channel.write('red_channel_output.jpg')
Using MiniMagick:
ruby require 'mini_magick' image = MiniMagick::Image.open('input.jpg') # Extract the red channel of the image image.channel 'R' image.write('red_channel_output.jpg')
5.3. Face Detection and Recognition:
Advanced image manipulation can involve face detection and recognition using specialized libraries like OpenCV, but Ruby provides integration options for these tasks as well.
6. Working with External Image Processing Libraries:
6.1. RMagick: Ruby’s Swiss Army Knife for Image Processing:
RMagick is a comprehensive Ruby binding to the ImageMagick image-processing library. It provides a vast array of functionalities for image manipulation, from basic operations to advanced tasks.
6.2. MiniMagick: A Lightweight Alternative:
MiniMagick is a minimalistic and easy-to-use Ruby library that leverages the command-line tools of ImageMagick. Although it offers fewer features than RMagick, it is a lightweight alternative for simple image processing tasks.
6.3. ImageMagick Integration:
Both RMagick and MiniMagick rely on ImageMagick, a powerful and widely used open-source software suite for image processing tasks. To use RMagick or MiniMagick effectively, ensure you have ImageMagick installed on your system.
7. Best Practices for Image Manipulation in Ruby:
7.1. Memory Management and Performance Optimization:
Image manipulation tasks can be memory-intensive, so it’s essential to manage memory efficiently. Avoid unnecessary duplication of images and ensure you release resources promptly when you no longer need them.
7.2. Error Handling and Exception Control:
Image manipulation operations may encounter errors, such as missing files or unsupported formats. Implement proper error handling and exception control to provide meaningful feedback to users and prevent application crashes.
7.3. Automation and Batch Processing:
For tasks that involve processing a large number of images, consider automating the process with batch processing techniques. This can significantly save time and effort when dealing with multiple images.
Conclusion:
Image manipulation is a powerful skill that can elevate your web development and graphic design projects to new heights. Ruby, with its numerous functions and libraries, offers a user-friendly and effective way to perform image manipulation tasks. In this guide, we covered the basics of image manipulation with Ruby, explored various functions and techniques, and introduced you to popular image processing libraries like RMagick and MiniMagick. Armed with this knowledge, you can now confidently create stunning visual content and optimize images like a pro. So, go ahead and unleash your creativity with Ruby’s image manipulation capabilities!
Table of Contents
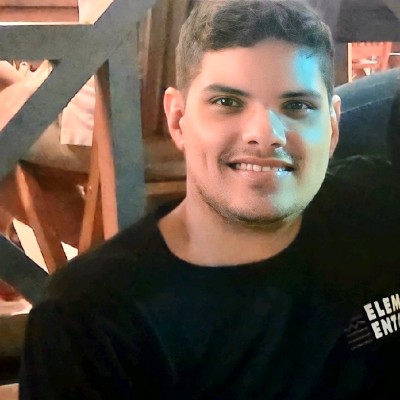
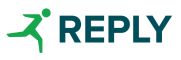