How to Use Ruby Functions for JSON Parsing and Generation
JSON (JavaScript Object Notation) has become a widely adopted format for data interchange due to its simplicity and human-readability. As a Ruby developer, having the ability to efficiently parse and generate JSON data is crucial. Ruby provides a robust set of built-in functions and libraries to simplify these tasks and streamline your JSON handling processes.
In this comprehensive guide, we will explore the ins and outs of using Ruby functions for JSON parsing and generation. Whether you’re a seasoned Ruby developer or a newcomer to the language, this tutorial will equip you with the necessary knowledge and skills to manipulate JSON data like a pro.
What is JSON?
JSON is a lightweight data-interchange format that is easy for humans to read and write, and equally simple for machines to parse and generate. It is primarily used for transmitting data between a server and a web application as an alternative to XML. JSON data is composed of key-value pairs and supports various data types such as strings, numbers, booleans, arrays, and nested objects.
In Ruby, JSON data is typically represented as a combination of hashes and arrays, mirroring the structure of the original JSON document.
JSON Parsing in Ruby
Parsing JSON is the process of converting a JSON-formatted string into a Ruby object. Ruby provides the json library, which offers convenient functions for parsing JSON data.
1. Parsing JSON from a File
To parse JSON data from a file, you can use the File and JSON modules in Ruby. The following code snippet demonstrates how to parse JSON from a file:
ruby require 'json' # Read JSON data from file json_data = File.read('data.json') # Parse JSON into a Ruby object parsed_data = JSON.parse(json_data) # Access and manipulate the parsed data # …
In this example, the File.read function is used to read the JSON data from a file named data.json. Then, the JSON.parse function is employed to convert the JSON string into a Ruby object, which can be accessed and manipulated as needed.
2. Parsing JSON from a String
If you already have the JSON data as a string, you can directly parse it using the JSON.parse function. Here’s an example:
ruby require 'json' # JSON data as a string json_data = '{"name": "John", "age": 30, "city": "New York"}' # Parse JSON into a Ruby object parsed_data = JSON.parse(json_data) # Access and manipulate the parsed data # …
In this case, the JSON.parse function converts the provided JSON string into a Ruby object, enabling you to access and manipulate the data.
Accessing JSON Data in Ruby
Once you have parsed the JSON data into a Ruby object, you can access its properties and elements using standard Ruby syntax.
1. Accessing Object Properties
To access properties within a JSON object, you can use the key names as methods or array-style indexing. Consider the following example:
ruby # Parsed JSON object data = { "name" => "John", "age" => 30, "city" => "New York" } # Access object properties name = data["name"] age = data["age"] city = data["city"] # Output the values puts "Name: #{name}" puts "Age: #{age}" puts "City: #{city}"
In this snippet, the object properties, such as “name,” “age,” and “city,” are accessed using the corresponding keys within square brackets. The values are then assigned to separate variables and displayed using the puts function.
2. Accessing Array Elements
When working with JSON arrays, you can access individual elements using zero-based indexing. Here’s an example:
ruby # Parsed JSON array data = [10, 20, 30, 40, 50] # Access array elements first_element = data[0] second_element = data[1] last_element = data[-1] # Output the values puts "First Element: #{first_element}" puts "Second Element: #{second_element}" puts "Last Element: #{last_element}"
In this code snippet, the array elements are accessed using square brackets and the corresponding indexes. The first element has an index of 0, the second element has an index of 1, and the last element can be accessed using a negative index of -1.
3. Handling Nested JSON Structures
JSON data often contains nested structures, where objects or arrays are nested within one another. Ruby allows you to navigate these nested structures using a combination of property access and indexing.
Consider the following example of a nested JSON object:
ruby # Parsed JSON object with nested structure data = { "name" => "John", "age" => 30, "address" => { "street" => "123 Main St", "city" => "New York", "country" => "USA" } } # Access nested properties street = data["address"]["street"] city = data["address"]["city"] country = data["address"]["country"] # Output the values puts "Street: #{street}" puts "City: #{city}" puts "Country: #{country}"
In this example, the nested properties of the “address” object are accessed by chaining the property names together. By accessing data[“address”], we obtain the nested object, and then we can further access its properties using additional square brackets.
Modifying JSON Data in Ruby
Ruby provides various methods to modify JSON data once it has been parsed into a Ruby object. Let’s explore some common techniques for modifying JSON data.
1. Updating Object Properties
To update a specific property within a JSON object, you can simply assign a new value to the corresponding key. Here’s an example:
ruby # Parsed JSON object data = { "name" => "John", "age" => 30, "city" => "New York" } # Update object properties data["name"] = "Jane" data["age"] = 35 # Output the updated values puts "Name: #{data["name"]}" puts "Age: #{data["age"]}" puts "City: #{data["city"]}"
In this code snippet, we update the “name” and “age” properties of the JSON object by assigning new values to the corresponding keys. The updated values are then displayed using the puts function.
2. Modifying Array Elements
When working with JSON arrays, you can modify individual elements by accessing them using their indexes and assigning new values. Consider the following example:
ruby # Parsed JSON array data = [10, 20, 30, 40, 50] # Update array elements data[0] = 100 data[2] = 300 # Output the updated values puts "First Element: #{data[0]}" puts "Third Element: #{data[2]}" puts "All Elements: #{data}"
In this snippet, we update the first and third elements of the JSON array by assigning new values to the corresponding indexes. The updated values are then displayed using the puts function.
3. Adding and Removing JSON Elements
To add new properties to a JSON object or append elements to a JSON array, you can use the standard Ruby methods available for hashes and arrays.
Consider the following example:
ruby # Parsed JSON object data = { "name" => "John", "age" => 30 } # Add a new property data["city"] = "New York" # Output the updated object puts data.to_json # Parsed JSON array data = [10, 20, 30] # Append a new element data << 40 # Output the updated array puts data.to_json
In this code snippet, we add a new property, “city,” to the JSON object by simply assigning a value to a new key. For the JSON array, we use the << operator to append a new element, 40, to the array.
Generating JSON in Ruby
Apart from parsing JSON, Ruby allows you to generate JSON data from Ruby objects, making it easy to produce JSON output for APIs and other purposes. The json library provides the necessary functions and methods to accomplish this.
1. Creating JSON Objects
To create a JSON object in Ruby, you can use a combination of hashes and arrays. Here’s an example:
ruby require 'json' # Create a JSON object json_object = { "name" => "John", "age" => 30, "city" => "New York" } # Convert the object to JSON json_data = JSON.generate(json_object) # Output the generated JSON puts json_data
In this code snippet, we define a Ruby hash with the desired key-value pairs to represent a JSON object. Then, we use the JSON.generate function to convert the Ruby object into a JSON-formatted string. Finally, the generated JSON data is displayed using the puts function.
2. Creating JSON Arrays
To create a JSON array in Ruby, you can use an array to represent the desired sequence of elements. Consider the following example:
ruby require 'json' # Create a JSON array json_array = ["apple", "banana", "orange"] # Convert the array to JSON json_data = JSON.generate(json_array) # Output the generated JSON puts json_data
In this example, we define a Ruby array containing the desired elements for the JSON array. We then use the JSON.generate function to convert the Ruby array into a JSON-formatted string. Finally, the generated JSON data is displayed using the puts function.
3. Converting Ruby Objects to JSON
Ruby objects can be directly converted to JSON using the to_json method provided by the json library. This method is available for most Ruby built-in classes and can also be implemented for custom classes by overriding the to_json method.
Here’s an example using a custom class:
ruby require 'json' class Person attr_accessor :name, :age def initialize(name, age) @name = name @age = age end def to_json(*args) { "name" => name, "age" => age }.to_json(*args) end end # Create a person object person = Person.new("John", 30) # Convert the object to JSON json_data = person.to_json # Output the generated JSON puts json_data
In this code snippet, we define a Person class with a custom to_json method. The method converts the Person object into a hash and then calls the to_json method on the hash to generate the JSON-formatted string.
Advanced JSON Manipulation in Ruby
Ruby provides additional features and techniques for advanced JSON manipulation. Let’s explore a few of them.
1. Working with Complex JSON Structures
JSON data can have complex structures with nested objects, arrays, and multiple levels of nesting. To handle such scenarios, you can combine the techniques we’ve covered earlier, including accessing properties and elements, modifying data, and generating JSON.
Here’s an example that demonstrates working with a complex JSON structure:
ruby require 'json' # Parsed JSON object with complex structure data = { "name" => "John", "age" => 30, "address" => { "street" => "123 Main St", "city" => "New York", "country" => "USA" }, "skills" => ["Ruby", "Python", "JavaScript"] } # Access and modify nested properties data["address"]["city"] = "San Francisco" data["skills"] << "Java" # Output the updated JSON puts JSON.generate(data)
In this example, we have a complex JSON object with nested properties and an array. We access and modify the “city” property within the “address” object, and also append a new skill to the “skills” array. Finally, we generate and output the updated JSON data.
2. Handling Dates and Times in JSON
When working with dates and times in JSON, it’s important to ensure proper formatting and handling. Ruby provides the Time and Date classes, which can be serialized to and deserialized from JSON.
Here’s an example that demonstrates handling dates and times in JSON:
ruby require 'json' require 'date' # Create a Ruby date object date = Date.today # Convert the date object to JSON json_data = date.to_json # Output the generated JSON puts json_data # Parse the JSON back to a Ruby date object parsed_date = JSON.parse(json_data, object_class: Date) # Output the parsed date puts parsed_date
In this code snippet, we first create a Date object representing the current date. We then convert the date object to JSON using the to_json method. The generated JSON data is displayed. Next, we parse the JSON data back into a Ruby date object using the JSON.parse function, specifying the object_class option as Date. Finally, we output the parsed date.
3. Validating JSON Data
Validating JSON data is important to ensure its integrity and adherence to a specific schema or structure. Ruby provides various JSON schema validation libraries, such as json-schema and json_schemer, which allow you to define and enforce validation rules for your JSON data.
Here’s an example that demonstrates JSON validation using the json-schema gem:
ruby require 'json' require 'json-schema' # JSON schema definition schema = { "type" => "object", "properties" => { "name" => { "type" => "string" }, "age" => { "type" => "number" }, "city" => { "type" => "string" } }, "required" => ["name", "age"] } # JSON data to validate json_data = '{"name": "John", "age": 30}' # Validate the JSON data against the schema validation_result = JSON::Validator.validate(schema, json_data) # Output the validation result puts "Validation Result: #{validation_result}"
In this code snippet, we define a JSON schema that specifies the expected structure and data types for the JSON data. We then provide a JSON string to validate against the schema using the JSON::Validator.validate method. The method returns true if the data is valid according to the schema, and false otherwise.
Best Practices for JSON Handling in Ruby
When working with JSON in Ruby, it’s essential to follow best practices to ensure efficient and reliable code. Here are a few recommendations:
1. Error Handling
Always handle potential errors when parsing or generating JSON data. Ruby provides exception handling mechanisms, such as begin, rescue, and ensure, which allow you to gracefully handle and recover from errors that may occur during JSON operations.
For example:
ruby require 'json' begin json_data = '{"name": "John", "age": 30}' parsed_data = JSON.parse(json_data) rescue JSON::ParserError => e puts "Error parsing JSON: #{e.message}" end
In this code snippet, we use a begin and rescue block to catch and handle any potential JSON::ParserError exceptions that may occur during parsing. The error message is then displayed using the puts function.
2. Performance Considerations
When dealing with large JSON data or performing frequent JSON operations, consider the performance implications of your code. Parsing or generating JSON data can be computationally expensive, so optimizing your code for efficiency is crucial.
Some performance tips include:
- Minimize unnecessary parsing and serialization by reusing parsed JSON objects whenever possible.
- Consider using Ruby’s built-in json library instead of external libraries for better performance.
- Use JSON.generate instead of to_json for improved performance when generating JSON.
Conclusion
In this comprehensive guide, we explored the power and flexibility of using Ruby functions for JSON parsing and generation. We covered various aspects, including parsing JSON from files and strings, accessing and modifying JSON data, generating JSON from Ruby objects, and advanced JSON manipulation techniques.
By leveraging the built-in functions and libraries provided by Ruby, you can efficiently work with JSON data, whether you need to consume APIs, manipulate data structures, or build web applications. With practice and hands-on experience, you’ll become proficient in JSON handling and be well-equipped to tackle real-world JSON-related tasks using Ruby.
Table of Contents
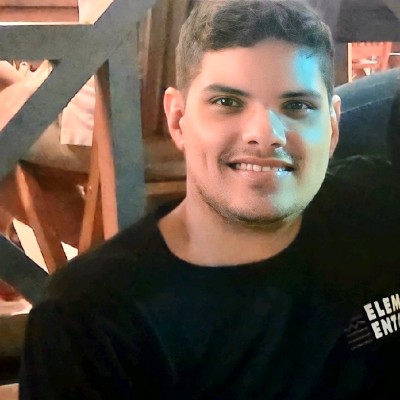
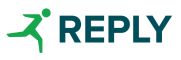