How to Use Ruby Functions for Payment Gateway Integration
In today’s digital age, online payments have become an integral part of many businesses. Whether you run an e-commerce store, a subscription-based service, or a donation platform, integrating payment gateways is essential for processing transactions securely and efficiently. Ruby, a dynamic and versatile programming language, offers a powerful way to accomplish this task through functions. In this comprehensive guide, we will explore how to use Ruby functions for payment gateway integration, step by step.
Table of Contents
1. Why Payment Gateway Integration Matters
Before diving into the technical details, it’s crucial to understand the significance of payment gateway integration. Payment gateways are the digital counterparts of physical card terminals. They securely transmit payment information between customers, businesses, and financial institutions, ensuring that transactions are completed accurately and safely. Here are a few reasons why payment gateway integration is essential:
1.1. Enhanced Customer Experience
Integrating a payment gateway into your application enhances the customer experience by providing a seamless and convenient way to make payments. Customers can quickly and securely purchase products or services without leaving your platform.
1.2. Security and Compliance
Payment gateways adhere to strict security standards, such as PCI DSS (Payment Card Industry Data Security Standard), to protect sensitive financial information. By integrating a trusted payment gateway, you can ensure your application complies with these regulations, reducing the risk of data breaches and fraud.
1.3. Global Reach
Payment gateways support various payment methods and currencies, making it easier for your business to reach a global audience. You can cater to customers worldwide and expand your market presence without worrying about currency conversion and payment processing.
1.4. Efficient Transaction Processing
Payment gateways facilitate real-time transaction processing, allowing you to receive payments quickly. This efficiency is crucial for businesses that rely on cash flow to operate smoothly.
Now that you understand why payment gateway integration is vital, let’s move on to the practical aspect: how to use Ruby functions to achieve this seamlessly.
2. Choosing the Right Payment Gateway
The first step in payment gateway integration is selecting the right payment gateway for your business needs. Different gateways offer various features, pricing models, and geographic coverage. Popular payment gateways that Ruby developers commonly integrate include:
2.1. Stripe
Stripe is known for its developer-friendly API and comprehensive documentation. It supports a wide range of payment methods and is an excellent choice for startups and established businesses alike.
2.2. PayPal
PayPal is a globally recognized payment gateway with a massive user base. Integrating PayPal can help you reach a vast customer audience, especially if you operate internationally.
2.3. Braintree
Braintree, owned by PayPal, provides a robust and scalable payment solution. It’s an excellent choice for businesses that require in-app payments or subscription billing.
2.4. Square
Square offers both online and in-person payment solutions, making it suitable for businesses with physical storefronts and online operations.
Once you’ve chosen the payment gateway that suits your business, you can start the integration process.
3. Setting Up Your Ruby Environment
Before you can use Ruby functions for payment gateway integration, ensure you have Ruby and any necessary gems (libraries) installed. You’ll also need an active developer account with your chosen payment gateway to access their API credentials.
3.1. Installing Ruby
If you don’t already have Ruby installed, you can download it from the official website (https://www.ruby-lang.org/en/documentation/installation/) or use a version management tool like RVM (Ruby Version Manager) or rbenv.
3.2. Installing Required Gems
Use the gem command to install any gems required for your chosen payment gateway. For example, if you’re using Stripe, you can install the Stripe gem:
ruby gem install stripe
3.3. Obtaining API Credentials
Log in to your payment gateway’s developer portal to obtain API credentials, including API keys and secrets. These credentials will authenticate your application with the gateway’s servers, allowing you to send payment requests securely.
Now that your environment is set up let’s start building Ruby functions for payment gateway integration.
4. Creating a Ruby Function for Payment Processing
In this section, we will create a Ruby function that handles payment processing using your chosen payment gateway. We’ll provide an example using the Stripe payment gateway, but the general approach applies to other gateways as well.
4.1. Require the Payment Gateway Gem
In your Ruby script or application, begin by requiring the payment gateway gem you installed earlier. For Stripe, use the following line of code:
ruby require 'stripe'
4.2. Configure API Credentials
Next, configure the API credentials you obtained from your payment gateway’s developer portal. These credentials authenticate your application with the gateway’s servers. For Stripe, set your API key as follows:
ruby Stripe.api_key = 'your_stripe_api_key_here'
4.3. Create a Payment Function
Now, let’s create a Ruby function that handles payment processing. This function will take parameters such as the payment amount, currency, and customer information. Here’s a simplified example:
ruby def process_payment(amount, currency, customer_email, card_token) begin # Create a new customer customer = Stripe::Customer.create( email: customer_email, source: card_token ) # Create a charge charge = Stripe::Charge.create( amount: amount * 100, # Amount in cents currency: currency, customer: customer.id ) # Payment successful return charge rescue Stripe::StripeError => e # Handle payment failure puts "Payment failed: #{e.message}" return nil end end
In this example, we first create a customer using the customer’s email and a card token representing their payment method. Then, we create a charge with the specified amount and currency, associating it with the customer we just created. If the payment is successful, the function returns the charge object; otherwise, it handles the payment failure.
4.4. Calling the Payment Function
To process payments in your application, call the process_payment function and pass the required parameters. For example:
ruby # Replace these values with actual data amount = 50.00 # Payment amount in your currency currency = 'USD' customer_email = 'customer@example.com' card_token = 'tok_visa' # Replace with an actual card token # Process the payment charge = process_payment(amount, currency, customer_email, card_token) if charge puts "Payment successful! Charge ID: #{charge.id}" # Add your code to handle the successful payment here else puts "Payment failed." # Add your code to handle payment failure here end
This code snippet demonstrates how to call the process_payment function and handle the payment result. If the payment is successful, it prints the charge ID; otherwise, it indicates that the payment failed.
5. Handling Payment Gateway Responses
Payment gateways return various responses that you should handle in your Ruby application. Here are some common responses and how to handle them:
5.1. Successful Payment
If the payment is successful, the payment gateway will typically return a confirmation or transaction ID. You can save this ID in your database for future reference and send a confirmation email to the customer.
5.2. Payment Declined
If the payment is declined, the gateway will provide a reason for the decline. It could be due to insufficient funds, an expired card, or other issues. Inform the customer about the decline and provide guidance on resolving the issue.
5.3. Errors and Exceptions
Payment gateways may also return errors or exceptions, such as network issues or invalid API requests. Ensure your application logs these errors for debugging purposes and handles them gracefully by notifying the user or retrying the transaction.
6. Testing Your Integration
Before deploying your payment gateway integration to a production environment, it’s crucial to test it thoroughly. Most payment gateways offer sandbox or test modes that allow you to simulate payments without involving real money. Here’s how to test your Ruby payment gateway integration:
6.1. Use Test API Credentials
Switch your payment gateway configuration to use test API credentials instead of live ones. These credentials are provided by the gateway for testing purposes.
6.2. Simulate Different Scenarios
Test your integration with various scenarios, including successful payments, declined payments, and network errors. Ensure your application handles each scenario correctly and provides appropriate feedback to the user.
6.3. Check for Security Vulnerabilities
Perform security testing to identify and fix any vulnerabilities in your payment gateway integration. This includes checking for data encryption, input validation, and proper error handling.
6.4. Monitor Performance
Evaluate the performance of your payment gateway integration under different load conditions. Ensure it can handle a high volume of transactions without performance degradation.
7. Deploying Your Payment Gateway Integration
Once you’ve thoroughly tested your payment gateway integration, you can deploy it to your production environment. Here are some best practices for a smooth deployment:
7.1. Rollout Plan
Plan your deployment carefully, considering the impact on your users. It’s often a good idea to deploy during low-traffic periods to minimize disruption.
7.2. Monitoring and Error Handling
Implement monitoring and error tracking to quickly identify and resolve any issues that may arise in the production environment. Use tools like log analyzers and error tracking services to stay on top of potential problems.
7.3. Compliance and Security
Ensure that your production environment complies with security standards and regulations, such as PCI DSS. Regularly update and patch your systems to protect customer data.
7.4. Customer Communication
Notify your customers about the new payment gateway integration and any changes in the payment process. Provide clear instructions on how to use the new system.
Conclusion
Integrating payment gateways into your Ruby applications is a crucial step for any online business. With the right choice of payment gateway and the use of Ruby functions, you can provide a secure and seamless payment experience for your customers. Remember to test your integration thoroughly and follow best practices for deployment and maintenance to ensure a smooth and reliable payment processing system for your business.
In this guide, we’ve covered the essential steps for using Ruby functions for payment gateway integration, from choosing the right gateway to deploying your solution in a production environment. By following these steps and best practices, you can unlock the full potential of online payments for your business, ultimately contributing to its success and growth.
Table of Contents
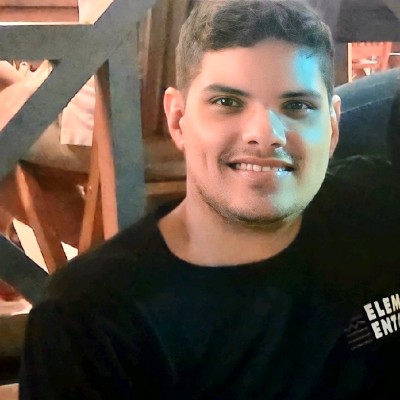
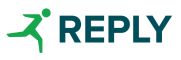