How to Use Ruby Functions for PDF Generation
In the modern digital age, generating PDF documents is a crucial aspect of many applications. Whether it’s generating invoices, reports, or other printable content, developers often need a straightforward and efficient way to create PDF files. Ruby, a popular and dynamic programming language, offers an array of powerful functions and libraries to accomplish this task with ease. In this blog post, we’ll explore how to use Ruby functions for PDF generation, step by step.
Table of Contents
1. Prerequisites
Before we dive into PDF generation, make sure you have Ruby installed on your system. Additionally, you’ll need a code editor and basic knowledge of Ruby programming.
2. Setting Up the Environment
First, let’s set up our development environment. Open your terminal or command prompt and create a new directory for our project:
bash mkdir pdf_generation_project cd pdf_generation_project
Next, initialize a new Ruby project by creating a Gemfile:
bash touch Gemfile
Edit the Gemfile and add the following lines to include the required gems:
ruby source 'https://rubygems.org' gem 'prawn' gem 'prawn-table'
Save the Gemfile and install the gems using Bundler:
bash bundle install
Great! Now we have all the necessary dependencies installed to start generating PDFs using Ruby.
3. Getting Started with Prawn
Prawn is a feature-rich and easy-to-use Ruby library for PDF generation. It allows us to create PDF documents from scratch, providing full control over the content and layout. Let’s create a simple PDF that says “Hello, PDF Generation!” using Prawn:
ruby require 'prawn' Prawn::Document.generate('hello_pdf.pdf') do text 'Hello, PDF Generation!' end
Save the code above into a file named pdf_generator.rb, and run the script:
bash ruby pdf_generator.rb
You’ll find a new PDF file, hello_pdf.pdf, in the same directory, containing our greeting message.
4. Adding Content to the PDF
Prawn provides various methods to add different types of content to the PDF, such as text, images, tables, and more. Let’s explore some of these functionalities through examples.
4.1. Adding Text
To add text to the PDF, use the text method as shown in the previous example. You can customize the font, size, and style using additional options:
ruby require 'prawn' Prawn::Document.generate('text_example.pdf') do text 'Hello, PDF Generation!', size: 24, style: :bold, align: :center, color: '0077FF' text 'Welcome to PDF Generation with Ruby!', size: 18, style: :italic, align: :center, color: '00BB00' end
4.2. Adding Images
To include images in the PDF, use the image method. Make sure to provide the correct file path:
ruby require 'prawn' Prawn::Document.generate('image_example.pdf') do image 'path/to/image.png', position: :center, vposition: :center, fit: [300, 200] end
4.3. Creating Tables
Prawn allows us to create tables with ease using the make_table method from the prawn-table gem:
ruby require 'prawn' require 'prawn/table' Prawn::Document.generate('table_example.pdf') do data = [['Name', 'Age', 'Occupation'], ['John Doe', 30, 'Developer'], ['Jane Smith', 25, 'Designer'], ['Bob Johnson', 40, 'Manager']] table(data, header: true, width: 400, cell_style: { size: 12 }) end
5. Styling the PDF
A well-designed PDF can leave a lasting impression on the readers. Prawn provides extensive styling options to enhance the visual appeal of your documents.
5.1. Fonts and Styles
You can use custom fonts and styles in your PDFs. First, download the font file (e.g., custom_font.ttf) and save it in the project directory. Then, utilize the font method to apply the custom font:
ruby require 'prawn' Prawn::Document.generate('styled_pdf.pdf') do font 'custom_font.ttf' do text 'This text uses a custom font!' end text 'This text has a different style.', style: :italic end
5.2. Colors and Backgrounds
Adding colors to your PDF can make it visually appealing. Use the fill_color and text_color methods to apply colors to text and shapes:
ruby require 'prawn' Prawn::Document.generate('colored_pdf.pdf') do fill_color 'FF0000' text 'This text is in red color.' fill_color '00FF00' text 'This text is in green color.' end
6. Page Layout and Numbering
Controlling the page layout and numbering is essential when generating multi-page PDFs. Prawn provides methods to set page size, margins, and page numbering.
6.1. Page Size and Margins
You can set custom page sizes and margins for your PDF:
ruby require 'prawn' Prawn::Document.generate('page_layout.pdf', page_size: 'A4', left_margin: 50, right_margin: 50, top_margin: 100, bottom_margin: 100) do text 'This PDF uses custom page size and margins.' end
6.2. Page Numbering
Page numbering helps readers navigate through lengthy PDFs. Prawn offers an easy way to add page numbers:
ruby require 'prawn' Prawn::Document.generate('numbered_pages.pdf') do 10.times do |i| start_new_page text "This is page #{i + 1}" end end
Conclusion
In this blog post, we explored the power of Ruby functions for PDF generation using the Prawn library. We learned how to set up the environment, create basic PDFs, add content, apply styling, and control page layout. With these tools at your disposal, you can effortlessly generate professional-looking PDF documents tailored to your application’s needs. Happy coding and happy PDF generation!
Table of Contents
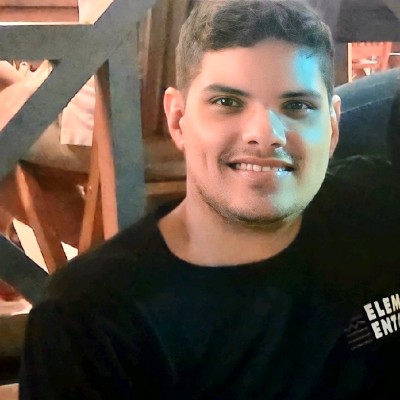
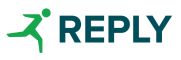