How to Use Ruby Functions for Recommendation Systems
In today’s digital landscape, recommendation systems are essential for delivering personalized content to users. From e-commerce platforms suggesting products to streaming services recommending movies, these systems enhance user experience and drive engagement. Ruby, with its simplicity and versatility, is an excellent choice for implementing recommendation algorithms. This blog will explore how to use Ruby functions to build basic recommendation systems, offering practical examples and key concepts.
Understanding Recommendation Systems
Recommendation systems use algorithms to suggest relevant items to users. They can be broadly categorized into three types:
- Content-Based Filtering: Recommends items similar to those a user has liked in the past.
- Collaborative Filtering: Recommends items based on the preferences of similar users.
- Hybrid Systems: Combine both content-based and collaborative filtering methods.
Implementing Content-Based Filtering
Content-based filtering recommends items similar to those a user has interacted with, based on item attributes. For example, in a movie recommendation system, if a user likes action movies, the system will suggest other action movies.
Example: Content-Based Filtering in Ruby
```ruby Sample dataset of movies movies = [ { title: "Action Movie 1", genre: "Action" }, { title: "Action Movie 2", genre: "Action" }, { title: "Romantic Movie 1", genre: "Romance" }, { title: "Comedy Movie 1", genre: "Comedy" } ] def recommend_movies(user_genre, movies) movies.select { |movie| movie[:genre] == user_genre } end User's preferred genre user_genre = "Action" recommended_movies = recommend_movies(user_genre, movies) puts recommended_movies ```
This code defines a list of movies with their genres and a function `recommend_movies` that filters movies based on the user’s preferred genre.
Implementing Collaborative Filtering
Collaborative filtering relies on user interactions, such as ratings or clicks, to recommend items. It assumes that users with similar behavior will like similar items. This method can be user-based or item-based.
Example: User-Based Collaborative Filtering in Ruby
```ruby Sample user ratings for items user_ratings = { alice: { "Movie 1" => 5, "Movie 2" => 3 }, bob: { "Movie 1" => 4, "Movie 3" => 4 }, charlie: { "Movie 2" => 5, "Movie 3" => 2 } } def find_similar_users(target_user, user_ratings) target_ratings = user_ratings[target_user] similarities = {} user_ratings.each do |user, ratings| next if user == target_user similarities[user] = calculate_similarity(target_ratings, ratings) end similarities.sort_by { |_, similarity| -similarity }.to_h end def calculate_similarity(ratings1, ratings2) common_items = ratings1.keys & ratings2.keys return 0 if common_items.empty? ratings1 = common_items.map { |item| ratings1[item] } ratings2 = common_items.map { |item| ratings2[item] } dot_product = ratings1.zip(ratings2).map { |x, y| x y }.sum magnitude1 = Math.sqrt(ratings1.map { |x| x2 }.sum) magnitude2 = Math.sqrt(ratings2.map { |x| x2 }.sum) dot_product / (magnitude1 magnitude2) end similar_users = find_similar_users(:alice, user_ratings) puts similar_users ```
In this example, `find_similar_users` identifies users similar to a target user based on their ratings, using cosine similarity. This function can help in finding users with similar tastes and recommending items they liked.
Implementing Hybrid Systems
Hybrid systems combine content-based and collaborative filtering methods to provide more accurate recommendations. For instance, a system might use collaborative filtering to identify similar users and then apply content-based filtering to recommend items within a specific genre.
Conclusion
Ruby offers powerful tools for building recommendation systems, allowing developers to implement various algorithms with ease. Whether you’re using content-based filtering, collaborative filtering, or a hybrid approach, Ruby’s flexibility and robust library support make it a great choice for creating personalized user experiences.
Further Reading
- [Introduction to Recommendation Systems](https://towardsdatascience.com/introduction-to-recommender-systems-6c66cf15ada)
- [Collaborative Filtering in Ruby](https://www.rubydoc.info/gems/collaborative-filtering/0.1.1)
- [Data Science with Ruby](https://pragprog.com/titles/dspruby/data-science-with-ruby/)
By mastering these techniques, you can enhance your application’s user experience and engagement, providing tailored recommendations that meet users’ preferences and needs. Happy coding!
Table of Contents
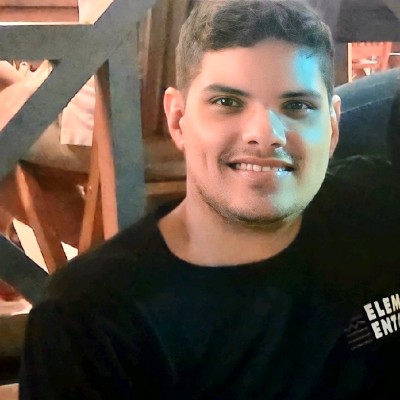
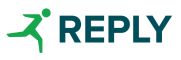