How to Use Ruby Functions for Regular Expressions
If you’re just starting out with Ruby, or even if you’re a seasoned veteran, the use of regular expressions (regex) can significantly improve your skill set. Regular expressions allow us to manipulate strings and search for patterns in an exceptionally powerful manner. This blog post will guide you through how to use Ruby functions for regular expressions effectively. Buckle up and let’s dive in!
Understanding Regular Expressions
Before we start, it’s essential to understand what regular expressions are. A regular expression is a sequence of characters that forms a search pattern. This pattern can be used for performing ‘search’ or ‘find and replace’ operations on strings. Regular expressions can range from being simple, for example, searching for a particular word in a text, to more complex patterns like email validation.
In Ruby, regular expressions are represented by the `Regexp` class. For example, `/ruby/` is a regular expression that matches the string ‘ruby’.
Creating Regular Expressions in Ruby
In Ruby, there are multiple ways to create regular expressions. One common way is using the `/pattern/` syntax. For example:
```ruby re = /ruby/
You can also use the `%r{pattern}` syntax when your pattern includes forward slashes. For example:
```ruby re = %r{/usr/bin}
Additionally, you can use the `Regexp.new` method:
```ruby re = Regexp.new('ruby')
Matching Regular Expressions
To match a regular expression with a string in Ruby, you use the `match` method. This method returns a `MatchData` object if a match is found, and `nil` otherwise. Here’s an example:
```ruby re = /ruby/ if match = re.match("I love ruby!") puts "Matched: #{match}" else puts "No match" end
The `=~` operator is another way to match a regular expression. It returns the index of the start of the match, or `nil` if there’s no match:
```ruby re = /ruby/ index = re =~ "I love ruby!" puts index # outputs 7
Regular Expression Modifiers
Ruby provides several modifiers to adjust the behavior of regular expressions:
– `i` for case-insensitive matching: `/ruby/i` will match ‘Ruby’, ‘RUBY‘, etc.
– `m` for multiline mode: `/a.b/m` will match ‘a\nb‘.
– `x` for extended mode, which ignores whitespace and allows comments: `/ a b x /x` will match ‘abx’.
– `o` for performing `#{}` substitutions only once, the first time the literal is evaluated.
Useful Regular Expression Methods
Ruby provides a range of useful methods for working with regular expressions.
`match`
As we’ve seen, this method checks whether a string matches a regular expression.
```ruby re = /ruby/ puts re.match("ruby") # outputs #<MatchData "ruby"> ```
`scan`
The `scan` method returns an array of all matches in a string.
```ruby re = /ruby/ puts "I love ruby, ruby is great!".scan(re) # outputs ["ruby", "ruby"]
`gsub` and `sub`
The `gsub` method replaces all occurrences of the regular expression in a string with the provided replacement. The `sub` method does the same but only for the first occurrence.
```ruby puts "I love ruby, ruby is great!".gsub(/ruby/, 'Ruby') # outputs "I love Ruby, Ruby is great!"
Practical Examples
Validating Email Address
Here’s an example of how we can use regular expressions to validate email addresses:
```ruby def valid_email?(email) re = /\A[\w+\-.]+@[a-z\d\-]+(\.[a-z\d\-]+)*\.[a-z]+\z/i !!email.match(re) end
Finding Words in a String
This example demonstrates how to find all occurrences of specific words in a string:
```ruby def find_words(text, words) words.map { |word| text.scan(/\b#{Regexp.escape(word)}\b/i) }.flatten end
Conclusion
Regular expressions are a powerful tool for string manipulation in Ruby. Their versatility allows for complex pattern searching and matching, significantly extending the functionality of your Ruby scripts. As with any powerful tool, mastering regular expressions may take some time, but the effort will undoubtedly pay off.
Remember, the best way to learn is by doing. Try to incorporate regular expressions into your next Ruby project. Experiment with different patterns, methods, and see the magic unfold. With regular expressions in your toolbox, you are well-equipped to tackle even the most complex text processing tasks. Happy coding!
Table of Contents
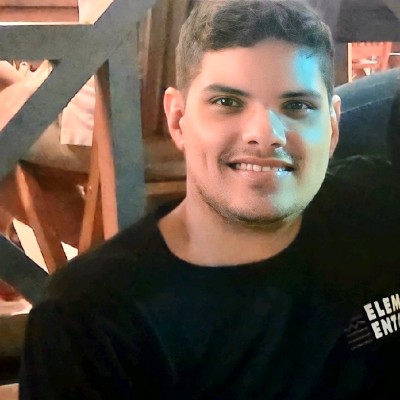
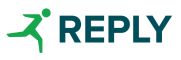