How to Use Ruby Functions for Sentiment Analysis
Sentiment analysis, also known as opinion mining, is a powerful technique that allows you to determine the sentiment or emotional tone behind a piece of text. It’s widely used in various applications, including social media monitoring, customer feedback analysis, and market research. If you’re a Ruby developer looking to incorporate sentiment analysis into your projects, you’re in the right place.
Table of Contents
In this guide, we’ll explore how to use Ruby functions for sentiment analysis. We’ll cover the basics of sentiment analysis, introduce essential Ruby libraries, and provide code samples to help you get started. Whether you’re a seasoned Ruby programmer or a newcomer to the language, you’ll find valuable insights in this tutorial.
1. Understanding Sentiment Analysis
Before diving into Ruby, it’s essential to understand what sentiment analysis is and how it works. Sentiment analysis aims to determine the emotional tone or sentiment expressed in a piece of text, which can be positive, negative, or neutral. It’s a valuable tool for businesses and researchers to gain insights from large volumes of text data.
1.1. The Importance of Sentiment Analysis
Business Insights: Companies can analyze customer reviews and social media mentions to gauge public opinion about their products or services, helping them make informed decisions.
- Customer Support: Sentiment analysis can be used to monitor customer support interactions, identifying cases where customers are dissatisfied or frustrated.
- Social Media Monitoring: Tracking sentiment on social media platforms helps organizations manage their online reputation and respond to customer feedback effectively.
- Market Research: Researchers can analyze sentiment in news articles and social media posts to understand public sentiment about specific topics or events.
Now that we understand why sentiment analysis is crucial, let’s explore how to perform it using Ruby.
2. Ruby for Sentiment Analysis
Ruby is a versatile programming language known for its simplicity and readability. It offers a range of libraries and tools that make it an excellent choice for natural language processing tasks like sentiment analysis. In this section, we’ll introduce you to two popular Ruby libraries for sentiment analysis: Sentimental and TextBlob.
2.1. Using the Sentimental Gem
The Sentimental gem is a Ruby library that provides a straightforward way to perform sentiment analysis on text data. To get started, you’ll need to install the gem:
ruby gem install sentimental
Once installed, you can use it to analyze the sentiment of a text string:
ruby require 'sentimental' analyzer = Sentimental.new analyzer.load_defaults text = "I love this product! It's fantastic." sentiment = analyzer.sentiment(text) puts "Sentiment: #{sentiment}"
The code above initializes a Sentimental analyzer, loads default sentiment analysis data, and then analyzes the sentiment of the provided text. The sentiment method returns one of three labels: “positive,” “negative,” or “neutral.”
2.2. Using the TextBlob Gem
TextBlob is another useful library for sentiment analysis in Ruby. It’s built on the NLTK and Pattern libraries and offers a more comprehensive set of natural language processing features. To use TextBlob for sentiment analysis, you’ll need to install the gem:
ruby gem install textblob
Here’s an example of how to analyze sentiment using TextBlob:
ruby require 'textblob' text = "This movie is terrible. I didn't enjoy it at all." blob = TextBlob.new(text) puts "Sentiment: #{blob.sentiment}"
The code above creates a TextBlob object from the input text and then retrieves the sentiment using the sentiment method. The sentiment is represented as a tuple, where the first value is the polarity (positive or negative), and the second value is the subjectivity (how subjective or objective the text is).
2.3. Choosing the Right Library
Both the Sentimental and TextBlob gems are excellent choices for performing sentiment analysis in Ruby. Your choice may depend on your specific requirements and the level of customization you need. Sentimental is more straightforward and suitable for quick sentiment analysis tasks, while TextBlob provides a broader range of natural language processing features.
3. Preprocessing Text Data
Before performing sentiment analysis, it’s essential to preprocess the text data to ensure accurate results. Text preprocessing involves cleaning and transforming the text to make it suitable for analysis. Common preprocessing steps include:
3.1. Lowercasing
Convert all text to lowercase to ensure uniformity and avoid treating “Good” and “good” as different words.
3.2. Removing Punctuation
Punctuation marks often don’t carry sentiment information and can be safely removed from the text.
3.3. Tokenization
Split the text into individual words or tokens. This step is crucial for analyzing the sentiment of each word.
3.4. Stopword Removal
Stopwords are common words like “the,” “and,” and “is” that don’t contribute much to sentiment analysis. Removing them can reduce noise in the analysis.
Let’s see how we can preprocess text data in Ruby:
ruby text = "This is a sample sentence for text preprocessing!" # Lowercasing text = text.downcase # Removing punctuation text = text.gsub(/[[:punct:]]/, '') # Tokenization tokens = text.split # Stopword removal (assuming a predefined list of stopwords) stopwords = ["a", "for", "is"] tokens = tokens.reject { |token| stopwords.include?(token) } puts "Processed Text: #{tokens.join(' ')}"
By preprocessing the text data, you’ll have cleaner and more meaningful input for sentiment analysis.
4. Sentiment Analysis in Practice
Now that we’ve covered the basics, let’s explore some practical examples of sentiment analysis using Ruby. We’ll use both the Sentimental and TextBlob libraries to analyze the sentiment of real-world text data.
4.1. Analyzing Social Media Sentiment
Suppose you want to analyze the sentiment of comments on a social media platform. You can retrieve these comments from an API or a database and then analyze them using Ruby. Here’s an example using the Sentimental gem:
ruby require 'sentimental' analyzer = Sentimental.new analyzer.load_defaults comments = [ "I had a great day today!", "This weather is awful.", "The event was fantastic!", "I'm feeling indifferent about this." ] comments.each do |comment| sentiment = analyzer.sentiment(comment) puts "Comment: #{comment}\nSentiment: #{sentiment}\n\n" end
In this example, we have an array of comments, and we use the Sentimental analyzer to determine their sentiment.
4.2. Analyzing Product Reviews
Another common use case for sentiment analysis is analyzing product reviews. Let’s say you have a dataset of product reviews, and you want to categorize them as positive, negative, or neutral. Here’s how you can do it with the TextBlob gem:
ruby require 'textblob' reviews = [ "This phone is amazing. I love it!", "The quality of this product is terrible.", "It's an okay product, nothing special.", "I have mixed feelings about this item." ] reviews.each do |review| blob = TextBlob.new(review) sentiment = blob.sentiment sentiment_label = if sentiment[0] > 0 "positive" elsif sentiment[0] < 0 "negative" else "neutral" end puts "Review: #{review}\nSentiment: #{sentiment_label}\n\n" end
In this example, we calculate sentiment based on the polarity value from the TextBlob analysis.
5. Customizing Sentiment Analysis
Both the Sentimental and TextBlob libraries allow you to customize sentiment analysis based on your specific needs. You can train these libraries on custom datasets or adjust their parameters to fine-tune the analysis. Customization is particularly useful when you have domain-specific text data or unique sentiment requirements.
5.1. Training a Custom Sentiment Model
To train a custom sentiment model, you’ll need labeled data where each text sample is associated with its sentiment label (positive, negative, or neutral). You can then use this data to train the library to recognize sentiment patterns specific to your domain. Training a custom model can significantly improve the accuracy of sentiment analysis for your particular use case.
5.2. Fine-Tuning Parameters
Both libraries provide options to adjust parameters like sentiment thresholds, scoring, and feature extraction methods. By fine-tuning these parameters, you can adapt the sentiment analysis to align with your project’s requirements.
Conclusion
Sentiment analysis is a valuable technique for extracting insights from text data, and Ruby offers powerful libraries like Sentimental and TextBlob to make the process easy and efficient. By understanding the basics of sentiment analysis, preprocessing text data, and customizing analysis methods, you can effectively incorporate sentiment analysis into your Ruby projects.
Remember that the choice between libraries and customization options should be based on your project’s specific needs and the complexity of the sentiment analysis task. Whether you’re analyzing social media comments, product reviews, or any other type of text data, Ruby provides the tools you need to gain valuable insights from the sentiment expressed in the text. Start harnessing the power of sentiment analysis in Ruby today to make data-driven decisions and better understand your audience’s opinions.
Sentiment analysis is a powerful tool for understanding the emotional tone behind text data. Learn how to use Ruby’s libraries, Sentimental and TextBlob, for sentiment analysis in this comprehensive guide. We cover the basics of sentiment analysis, text preprocessing, and practical examples using real-world data. Whether you’re a Ruby beginner or an experienced developer, this tutorial will help you harness the power of sentiment analysis in your projects.
Table of Contents
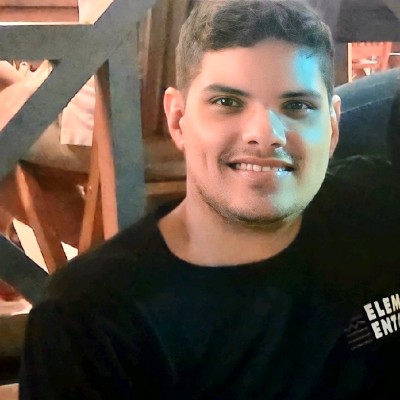
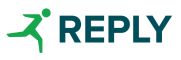