How to Use Ruby Functions for SMS Sending and Receiving
In today’s fast-paced digital world, communication is key. Whether you want to send automated notifications, provide real-time updates to your users, or simply engage with your audience, sending and receiving SMS messages can be a powerful tool. Ruby, with its elegant and expressive syntax, offers a fantastic environment for building SMS functionality into your applications. In this guide, we’ll explore how to use Ruby functions for SMS sending and receiving, using the popular Twilio API.
Table of Contents
1. Why Ruby for SMS?
Ruby is a versatile and user-friendly programming language known for its simplicity and readability. Its rich ecosystem of libraries and gems makes it an excellent choice for web development and integration tasks like SMS communication. When combined with Twilio, a powerful cloud communications platform, Ruby becomes an even more compelling choice for sending and receiving SMS messages.
Using Ruby with Twilio allows you to create applications that can:
- Send automated SMS notifications to users.
- Implement two-factor authentication via SMS.
- Build SMS-based chatbots and customer support systems.
- Manage SMS marketing campaigns.
- and much more.
In this guide, we’ll walk you through the process of sending and receiving SMS messages using Ruby and Twilio, so you can harness the full potential of this dynamic duo.
2. Getting Started with Twilio
Before you can start sending and receiving SMS messages with Ruby, you need to set up a Twilio account and configure your development environment.
2.1. Signing Up for Twilio
If you haven’t already, head over to the Twilio website and sign up for an account. Twilio offers a free trial with a balance of virtual currency (Twilio credit) that you can use to test and experiment with their services.
Once you’ve signed up, you’ll need to retrieve your Twilio Account SID and Auth Token from the Twilio dashboard. These credentials will be essential for authenticating your Ruby application with Twilio.
2.2. Setting Up Your Environment
To work with Ruby and Twilio, you’ll need to ensure that you have Ruby installed on your system. You can download Ruby from the official Ruby website.
Next, you’ll want to create a new directory for your Ruby SMS project and initialize a new Ruby project with Bundler. If you don’t have Bundler installed, you can do so with the following command:
bash gem install bundler
Now, navigate to your project directory and create a Gemfile with the following content:
ruby source 'https://rubygems.org' gem 'twilio-ruby'
This Gemfile specifies that your project depends on the twilio-ruby gem, which is the official Twilio gem for Ruby. To install the gem, run:
bash bundle install
With your environment set up, you’re ready to start sending SMS messages!
3. Sending SMS Messages
Sending SMS messages with Ruby and Twilio is straightforward. First, you need to install the Twilio Ruby gem, and then you can use it to send SMS messages programmatically.
3.1. Installing the Twilio Ruby Gem
We’ve already added the twilio-ruby gem to your project’s Gemfile. Now, let’s install it by running:
bash bundle install
This command will fetch and install the gem and its dependencies.
3.2. Sending Your First SMS
To send an SMS message, you’ll need your Twilio Account SID and Auth Token. Replace ‘YOUR_ACCOUNT_SID’ and ‘YOUR_AUTH_TOKEN’ with your actual credentials in the code snippet below:
ruby require 'twilio-ruby' # Your Twilio Account SID and Auth Token account_sid = 'YOUR_ACCOUNT_SID' auth_token = 'YOUR_AUTH_TOKEN' # Initialize the Twilio client client = Twilio::REST::Client.new(account_sid, auth_token) # Send an SMS message message = client.messages.create( from: '+1234567890', # Your Twilio phone number to: '+0987654321', # Recipient's phone number body: 'Hello from Ruby and Twilio!' ) puts "SMS sent with SID: #{message.sid}"
This code creates a Twilio client, specifies the sender’s phone number (Twilio phone number) and the recipient’s phone number, and sends the SMS message with the specified body.
When you run this script, you should see a confirmation message with the SID (Service Identifier) of the sent SMS.
You’ve just sent your first SMS message using Ruby and Twilio!
4. Receiving SMS Messages
Now that you know how to send SMS messages, let’s explore how to receive them using Ruby and Twilio. To receive SMS messages, you’ll need to set up a webhook and handle incoming messages.
4.1. Configuring a Webhook
A webhook is a URL where Twilio will send incoming SMS messages. To configure a webhook, you’ll need a public URL for your Ruby application. One way to achieve this during development is by using a tool like ngrok.
Download and install ngrok from their website.
Start ngrok with the following command:
bash ngrok http 3000
This command will create a secure public URL that forwards incoming requests to your local development server running on port 3000.
Take note of the ngrok-generated public URL; you’ll need it for the next steps.
4.2. Handling Incoming SMS
With your webhook URL in hand, you can configure Twilio to send incoming SMS messages to your Ruby application. In your Twilio dashboard, go to the “Phone Numbers” section and select the phone number you want to use for receiving SMS messages.
Under the “Messaging” section, find the “A Message Comes In” option and set it to “Webhook.” Enter your ngrok-generated public URL followed by /sms/receive as the webhook URL, like so:
arduino http://your-ngrok-url.ngrok.io/sms/receive
Save your changes.
Now, let’s create a Ruby route to handle incoming SMS messages. In your Ruby application, add the following code:
ruby require 'sinatra' require 'twilio-ruby' # Your Twilio Account SID and Auth Token account_sid = 'YOUR_ACCOUNT_SID' auth_token = 'YOUR_AUTH_TOKEN' # Initialize the Twilio client client = Twilio::REST::Client.new(account_sid, auth_token) # Define a route for receiving SMS messages post '/sms/receive' do message_body = params['Body'] sender_number = params['From'] # You can process the incoming message here # For now, let's just echo it back client.messages.create( from: '+1234567890', # Your Twilio phone number to: sender_number, # Reply to the sender body: "You said: #{message_body}" ) # Send a response back to Twilio status 204 end
In this code, we’ve created a Sinatra route at /sms/receive that listens for incoming POST requests from Twilio. When Twilio receives an SMS on your designated phone number, it sends a POST request to your webhook URL, and your Ruby application handles it by echoing the received message back to the sender.
Now, when someone sends an SMS to your Twilio phone number, they’ll receive an automated reply with their message.
Congratulations! You’ve set up a webhook to receive and respond to SMS messages with Ruby and Twilio.
5. Advanced SMS Handling
While the basics of sending and receiving SMS messages are covered, there are many advanced features and techniques you can explore to enhance your Ruby and Twilio SMS integration.
5.1. Storing SMS in a Database
For many applications, it’s essential to store incoming SMS messages in a database for later analysis or reference. You can use Ruby’s database libraries like ActiveRecord or Sequel to accomplish this. Here’s a high-level overview of how you can store SMS messages in a PostgreSQL database:
- Set up a PostgreSQL database for your Ruby application.
- Create a database table to store SMS messages with columns for the message text, sender’s phone number, and a timestamp.
- Modify your /sms/receive route to save incoming SMS messages to the database before sending a response.
5.2. Replying to SMS
Instead of echoing incoming SMS messages, you can create more sophisticated SMS chatbots or interactive SMS applications. Twilio allows you to receive user input through SMS and respond accordingly. You can implement features like automated customer support, surveys, and more.
To reply intelligently to incoming SMS messages, you’ll need to parse and understand user input. You can use natural language processing (NLP) libraries like wit.ai or Dialogflow to extract meaning from text messages and generate context-aware responses.
Conclusion
Ruby and Twilio make a formidable pair when it comes to handling SMS communication in your applications. In this guide, you’ve learned how to send and receive SMS messages using Ruby, Twilio, and a webhook. You’ve also explored some advanced topics like database storage and intelligent SMS replies.
With this knowledge, you have the tools to build powerful SMS-based features that can enhance user experiences, automate notifications, and engage with your audience in a whole new way. So, what are you waiting for? Dive into the world of Ruby and Twilio and start creating SMS magic today!
Table of Contents
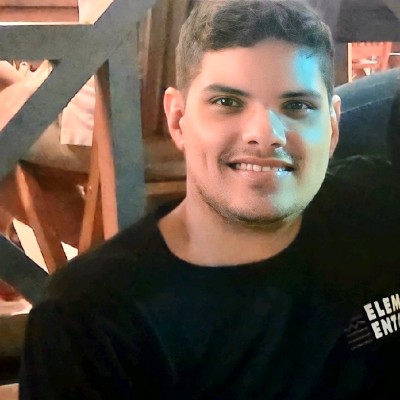
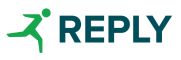