How to Use Ruby Functions for String Manipulation
Ruby is a high-level, interpreted programming language that is often praised for its elegance and readability. Among its many features is its powerful set of string manipulation methods. In this post, we will explore how to use Ruby functions to perform various string manipulation tasks, including string interpolation, string concatenation, string conversion, pattern matching, and more.
String Interpolation
String interpolation is a feature that allows you to insert variables or expressions within a string, and the string will include the variable’s value. In Ruby, the syntax for string interpolation is `#{expression}`.
name = "Alice" puts "Hello, #{name}" # => "Hello, Alice"
String Concatenation
In Ruby, you can combine or concatenate two or more strings using the `+` operator or `concat` method. The `+` operator returns a new string, while the `concat` method modifies the original string.
str1 = "Hello" str2 = ", World" puts str1 + str2 # => "Hello, World" str1.concat(str2) puts str1 # => "Hello, World"
String Conversion
Ruby provides the `to_s`, `to_i`, and `to_f` methods to convert variables to string, integer, and float types, respectively.
num = 5 puts num.to_s # => "5"
Case Conversion
There are various methods available in Ruby for converting the case of a string. The `upcase` method converts all the characters of a string to uppercase, the `downcase` method converts them to lowercase, and the `capitalize` method converts the first character of a string to uppercase and the rest to lowercase.
str = "Hello World" puts str.upcase # => "HELLO WORLD" puts str.downcase # => "hello world" puts str.capitalize # => "Hello world"
Substring
There are a few ways to extract a substring in Ruby, including using array-like indexing or the `slice` method.
str = "Hello, World" puts str[0,5] # => "Hello" puts str.slice(7,5) # => "World"
Pattern Matching and Substitution
Ruby’s pattern matching capabilities are quite powerful. It uses regular expressions (RegEx) to match patterns in strings. You can use the `match` method to find a pattern, and the `gsub` method to substitute a pattern with a new string.
str = "The quick brown fox jumps over the lazy dog" puts str.match(/quick/) # => "quick" puts str.gsub(/fox/, "cat") # => "The quick brown cat jumps over the lazy dog"
Stripping Whitespace
Ruby provides `strip`, `lstrip`, and `rstrip` methods to remove whitespace from both ends, the left end, or the right end of a string, respectively.
str = " Hello, World " puts str.strip # => "Hello, World" puts str.lstrip # => "Hello, World " puts str.rstrip # => " Hello, World"
Splitting and Joining Strings
The `split` method breaks a string into an array of substrings based on a delimiter, and the `join` method combines an array of strings into a single string.
str = "Hello, World" puts str.split(", ") # => ["Hello", "World"] arr = ["Hello", "World"] puts arr.join(", ") # => "Hello, World"
String Encoding
Ruby has a powerful system for managing string encodings. You can get the current encoding of a string with the `encoding` method, and change its encoding with the `force_encoding` or `encode` method.
str = "Hello, World" puts str.encoding.name # => "UTF-8" puts str.force_encoding("ASCII-8BIT").encoding.name # => "ASCII-8BIT"
Conclusion
Ruby’s array of string manipulation methods make it a powerful language for text processing. From basic operations like concatenation and case conversion, to more advanced tasks like pattern matching and encoding changes, Ruby offers a rich set of tools for manipulating strings.
While we’ve covered many common string manipulation methods here, there are many more available in Ruby. Always remember to explore the official Ruby documentation to discover more functions and how they can serve your particular needs. Happy coding!
Table of Contents
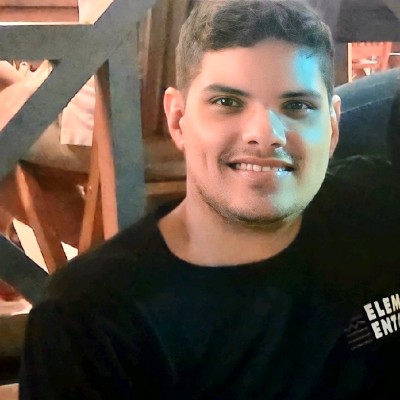
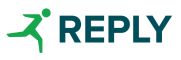