How to Use Ruby Functions for Web Scraping
Web scraping has become an indispensable tool for gathering data from websites, providing valuable insights, and automating various tasks. Ruby, a dynamic and expressive programming language, offers a wide range of tools and libraries that make web scraping a breeze. In this blog post, we will explore the power of Ruby functions and how they can be utilized to enhance your web scraping endeavors. By the end of this guide, you’ll have the knowledge to wield Ruby functions effectively and efficiently for your web scraping projects.
Understanding Web Scraping
Web scraping is the process of automatically extracting data from websites. It involves fetching web pages, parsing their HTML structure, and extracting relevant information. This data can then be stored, analyzed, or used for various purposes like research, monitoring, or data-driven decision making.
Introducing Ruby Functions
Ruby, known for its elegance and readability, provides a robust set of functions that can greatly simplify the web scraping process. Functions in Ruby allow you to encapsulate reusable blocks of code, making your scripts more modular and maintainable. By organizing your scraping logic into functions, you can improve code readability, reduce duplication, and enhance overall code structure.
Let’s dive into some practical examples of utilizing Ruby functions for web scraping.
Code Sample 1: Basic Function Structure
ruby def scrape_website(url) # Code to scrape the website goes here # ... end # Call the function with a specific URL scrape_website("https://example.com")
In the above code snippet, we define a function named scrape_website that accepts a URL as a parameter. Inside the function, you can implement your scraping logic, including making HTTP requests, parsing HTML, and extracting data. Once the function is defined, you can call it with different URLs to scrape various websites.
Setting Up Your Environment
Before we start exploring web scraping with Ruby functions, let’s ensure that our development environment is properly configured. Here are the steps to get started:
- Install Ruby: If you don’t have Ruby installed, visit the official Ruby website and download the latest stable version for your operating system.
- Install Required Gems: Gems are Ruby libraries that provide additional functionality. Two essential gems for web scraping are nokogiri and httparty. Install them by running the following commands in your terminal:
bash gem install nokogiri gem install httparty
With Ruby and the necessary gems installed, we’re ready to proceed with our web scraping journey.
Making HTTP Requests
To scrape a website, we first need to fetch its HTML content. Ruby offers several options for making HTTP requests, such as the built-in Net::HTTP library or the more user-friendly HTTParty gem. Let’s explore the latter.
Code Sample 2: Making an HTTP Request with HTTParty
ruby require 'httparty' def fetch_html(url) response = HTTParty.get(url) response.body end # Call the function with a specific URL html_content = fetch_html("https://example.com") puts html_content
In the above code, we include the httparty gem and define a function named fetch_html that takes a URL as a parameter. The HTTParty.get(url) method sends a GET request to the specified URL and returns a response object. We then retrieve the HTML body of the response using response.body and assign it to the html_content variable.
You can now use the html_content variable to parse the HTML and extract the desired data.
Parsing HTML with Nokogiri
To extract information from HTML, we need a reliable parsing library. Nokogiri is a popular gem that provides powerful tools for working with HTML and XML documents. Let’s explore how to parse HTML using Nokogiri.
Code Sample 3: Parsing HTML with Nokogiri
ruby require 'nokogiri' require 'open-uri' def parse_html(html) doc = Nokogiri::HTML(html) # Code to work with the parsed document goes here # ... end # Call the function with HTML content parsed_document = parse_html(html_content)
In the above code, we include the nokogiri gem and define a function named parse_html that accepts the HTML content as a parameter. We create a Nokogiri document using Nokogiri::HTML(html) and assign it to the doc variable. The doc object represents the parsed HTML document, allowing us to traverse its structure and extract the desired data.
Extracting Data with CSS Selectors
CSS selectors are a powerful tool for targeting specific elements within an HTML document. Nokogiri leverages CSS selectors to simplify the process of data extraction. Let’s see how we can extract data using CSS selectors.
Code Sample 4: Extracting Data with CSS Selectors
ruby def extract_data(parsed_doc) # Select and extract specific elements using CSS selectors title = parsed_doc.css('h1').text description = parsed_doc.css('.description').text # Process and manipulate the extracted data # ... end # Call the function with the parsed document extracted_data = extract_data(parsed_document)
In the above code, we define a function named extract_data that takes the parsed document as a parameter. We use CSS selectors within the css method to target specific elements and extract their contents. In this example, we extract the text of the <h1> element and the element with the class name “description.”
Once the data is extracted, you can further process and manipulate it according to your requirements.
Processing and Manipulating Data
Once we have extracted the desired data from the HTML document, we often need to process and manipulate it further. Ruby provides a wide range of built-in functions and methods that can be utilized for data transformation. Let’s explore some common techniques.
Code Sample 5: Processing and Manipulating Data
ruby def process_data(data) # Remove leading/trailing whitespace cleaned_data = data.strip # Convert to uppercase uppercase_data = cleaned_data.upcase # Split into an array of words words = uppercase_data.split # Perform additional processing/manipulation # ... end # Call the function with extracted data processed_data = process_data(extracted_data)
In the above code, we define a function named process_data that accepts the extracted data as a parameter. We apply various data manipulation techniques, such as removing leading/trailing whitespace using strip, converting the data to uppercase using upcase, and splitting it into an array of words using split. You can customize the processing steps according to your specific requirements.
Handling Pagination and Dynamic Content
Many websites paginate their content or load data dynamically through JavaScript. To scrape such websites effectively, we need to handle pagination and retrieve dynamically loaded content. Ruby provides libraries like mechanize and watir that can help automate interactions with web pages.
Code Sample 6: Handling Pagination and Dynamic Content with Mechanize
ruby require 'mechanize' def scrape_multiple_pages(base_url, num_pages) agent = Mechanize.new num_pages.times do |page| url = "#{base_url}/page/#{page+1}" page = agent.get(url) # Extract and process data from the page # ... end end # Call the function with a base URL and the number of pages to scrape scrape_multiple_pages("https://example.com/articles", 3)
In the above code, we include the mechanize gem and define a function named scrape_multiple_pages that accepts a base URL and the number of pages to scrape. We create a Mechanize object, which acts as a web browser, and iterate over the desired number of pages. Within the loop, we construct the URL for each page, fetch its content using agent.get(url), and proceed to extract and process the data as needed.
Dealing with Anti-Scraping Measures
Websites often implement measures to prevent or discourage web scraping, such as CAPTCHAs, rate limiting, or obfuscated HTML structures. While it’s important to respect a website’s terms of service, there are techniques you can employ to overcome some of these challenges.
Code Sample 7: Using Proxies with Mechanize
ruby require 'mechanize' def scrape_with_proxies(url, proxies) agent = Mechanize.new proxies.each do |proxy| agent.set_proxy(proxy[:ip], proxy[:port]) page = agent.get(url) # Extract and process data from the page # ... end end # Call the function with a URL and a list of proxies proxies = [ { ip: '123.456.789.1', port: '8080' }, { ip: '987.654.321.0', port: '8888' } ] scrape_with_proxies("https://example.com", proxies)
In the above code, we modify the scrape_with_proxies function to accept a URL and a list of proxies. We use the set_proxy method of the Mechanize object to set the proxy configuration for each request. By rotating through different proxies, you can mitigate IP blocking or rate-limiting issues.
Storing and Analyzing Scraped Data
Once the data is scraped, it’s crucial to store and analyze it effectively. Ruby provides various options for data storage, such as saving to CSV files, databases like MySQL or PostgreSQL, or cloud-based storage solutions. Additionally, you can utilize data analysis and visualization libraries like Pandas or Matplotlib to gain insights from your scraped data.
Code Sample 8: Storing Scraped Data in a CSV File
ruby require 'csv' def store_data_as_csv(data, filename) CSV.open(filename, 'w') do |csv| data.each do |row| csv << row end end end # Call the function with the data and a filename store_data_as_csv(scraped_data, 'data.csv')
In the above code, we use the built-in CSV library to store the scraped data in a CSV file. The CSV.open method allows us to create or open a file, and we iterate over the data, writing each row to the file using the << operator.
Conclusion
Web scraping with Ruby functions opens up a world of possibilities for extracting and processing data from websites. With the versatility of Ruby functions, you can create modular and maintainable scraping scripts, efficiently handle complex scenarios, and unlock the potential of web data for your projects. Armed with the knowledge and code samples provided in this guide, you’re well on your way to becoming a proficient web scraper using Ruby. Happy scraping!
Remember to be mindful of legal and ethical considerations while scraping websites and always respect the website’s terms of service and policies.
Table of Contents
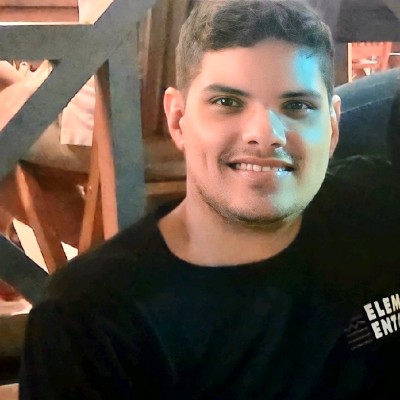
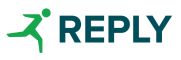