How to Use Ruby Functions for XML Parsing and Generation
XML (eXtensible Markup Language) has become a widely used format for representing and exchanging structured data. When working with XML files, it’s essential to have efficient tools for parsing and generating XML data. Ruby, a powerful and expressive programming language, provides robust functionality for XML manipulation through its built-in libraries and functions.
In this guide, we will explore the various methods and techniques available in Ruby for XML parsing and generation. We will cover both the basics and more advanced features, allowing you to handle XML data with ease and efficiency. Whether you’re a seasoned Ruby developer or just starting your XML journey, this blog will provide valuable insights and practical examples to enhance your XML handling skills.
Parsing XML with Ruby
Ruby provides several methods for parsing XML documents. Let’s explore the different techniques and options available:
1. Basic XML Parsing
To parse an XML document in Ruby, we can use the ‘REXML’ library. Consider the following XML file, ‘data.xml’, that we want to parse:
xml <?xml version="1.0" encoding="UTF-8"?> <bookstore> <book> <title>Ruby Programming</title> <author>John Smith</author> </book> <book> <title>Web Development with Ruby on Rails</title> <author>Jane Doe</author> </book> </bookstore>
To parse this XML and extract the data, we can use the following Ruby code:
ruby require 'rexml/document' # Read XML file file = File.read('data.xml') xml = REXML::Document.new(file) # Access root element root = xml.root # Iterate over book elements xml.elements.each('//book') do |book| title = book.elements['title'].text author = book.elements['author'].text puts "Title: #{title}, Author: #{author}" end
In the code snippet above, we first require the ‘rexml/document’ library to work with XML using REXML. We then read the XML file using File.read and create a new REXML::Document object with the file content.
We can access the root element using xml.root. In this example, the root element is <bookstore>. To iterate over each <book> element, we use xml.elements.each(‘//book’) and extract the title and author using the elements method.
2. Navigating XML Trees
XML documents often have complex hierarchical structures. Ruby provides convenient methods to navigate through XML trees.
Let’s consider the following XML structure:
xml <catalog> <book id="1"> <title>Book 1</title> <author>Author 1</author> <price>19.99</price> </book> <book id="2"> <title>Book 2</title> <author>Author 2</author> <price>29.99</price> </book> </catalog>
To access specific elements or attributes, we can use the following methods:
ruby # Accessing elements catalog = xml.root book = catalog.elements['book'] title = book.elements['title'].text # Accessing attributes book_id = book.attributes['id'] puts "Book ID: #{book_id}, Title: #{title}"
In the code snippet above, we access the <catalog> element using xml.root. We then access the first <book> element using catalog.elements[‘book’] and extract the <title> element using book.elements[‘title’].text.
To access attributes, we can use the attributes method. In this example, we retrieve the id attribute of the <book> element using book.attributes[‘id’].
3. Handling XML Attributes
XML elements can have attributes that provide additional information. Ruby allows us to access and modify these attributes easily.
Consider the following XML structure:
xml <product id="123" category="electronics"> <name>Smartphone</name> <price>599.99</price> </product>
To access and modify attributes, we can use the following code:
ruby product = xml.root product_id = product.attributes['id'] category = product.attributes['category'] # Update attributes product.attributes['id'] = 456 product.attributes['category'] = 'gadgets' puts "Product ID: #{product_id}, Category: #{category}"
In the code snippet above, we access the id and category attributes of the <product> element using product.attributes[‘id’] and product.attributes[‘category’], respectively.
To update attribute values, we can simply assign new values to them using product.attributes[‘id’] = 456 and product.attributes[‘category’] = ‘gadgets’.
Generating XML with Ruby
Apart from parsing XML, Ruby allows us to generate XML data programmatically. This is useful when creating XML documents from scratch or dynamically generating XML based on other data sources.
1. Creating XML Documents
To create a new XML document, we can use the ‘REXML’ library’s classes and methods.
ruby require 'rexml/document' # Create a new XML document xml = REXML::Document.new # Add the root element root = xml.add_element('catalog') # Add child elements book1 = root.add_element('book') book1.add_element('title').text = 'Book 1' book1.add_element('author').text = 'Author 1' book1.add_element('price').text = '19.99' book2 = root.add_element('book') book2.add_element('title').text = 'Book 2' book2.add_element('author').text = 'Author 2' book2.add_element('price').text = '29.99' # Print the XML puts xml.to_s
In the code snippet above, we create a new REXML::Document object using REXML::Document.new. We then add the root element, ‘catalog’, using xml.add_element(‘catalog’). We add child elements to the root element using root.add_element(‘book’) and set their text content using the text property.
To print the XML, we use xml.to_s, which converts the XML document to a string.
2. Adding Elements and Attributes
When generating XML, we can add elements and attributes to existing XML documents using various methods provided by Ruby.
Consider the following XML structure:
xml <catalog> <book id="1"> <title>Book 1</title> <author>Author 1</author> </book> </catalog>
To add a new book to the catalog, we can use the following code:
ruby catalog = xml.root # Add a new book element new_book = catalog.add_element('book') # Add attributes to the new book new_book.attributes['id'] = 2 # Add child elements to the new book new_book.add_element('title').text = 'Book 2' new_book.add_element('author').text = 'Author 2' puts xml.to_s
In the code snippet above, we access the root element, ‘catalog’, using xml.root. We then add a new book element using catalog.add_element(‘book’). We can set attributes for the new book using new_book.attributes[‘id’] = 2.
To add child elements to the new book, we use new_book.add_element(‘title’).text = ‘Book 2’ and new_book.add_element(‘author’).text = ‘Author 2’.
3. Writing XML to Files
Once we have generated or modified an XML document, we may want to save it to a file for future use or further processing. Ruby provides methods to write XML data to files easily.
Consider the following example, where we want to write an XML document to a file:
ruby require 'rexml/document' # Create a new XML document xml = REXML::Document.new root = xml.add_element('catalog') book = root.add_element('book') book.add_element('title').text = 'Book 1' book.add_element('author').text = 'Author 1' # Write XML to a file File.open('output.xml', 'w') do |file| file.write(xml.to_s) end
In the code snippet above, after generating the XML document, we open a new file, ‘output.xml’, in write mode using File.open(‘output.xml’, ‘w’). We then write the XML document to the file using file.write(xml.to_s). Finally, we close the file.
The resulting XML document will be written to ‘output.xml’.
Advanced XML Manipulation
Ruby offers additional advanced techniques for XML manipulation, including XPath querying, modifying existing XML, and transforming XML with XSLT.
1. XPath and XML Queries
XPath is a powerful language used to navigate and query XML documents. Ruby supports XPath queries through the ‘rexml/xpath’ library.
Consider the following XML structure:
xml <catalog> <book id="1"> <title>Book 1</title> <author>Author 1</author> </book> <book id="2"> <title>Book 2</title> <author>Author 2</author> </book> </catalog>
To perform XPath queries on this XML document, we can use the following code:
ruby require 'rexml/document' require 'rexml/xpath' # Read XML file file = File.read('data.xml') xml = REXML::Document.new(file) # Perform XPath query titles = REXML::XPath.match(xml, '//book/title') # Print the titles titles.each do |title| puts title.text end
In the code snippet above, after parsing the XML document, we require the ‘rexml/xpath’ library. We then use REXML::XPath.match to perform an XPath query on the XML document, searching for all ‘title’ elements under ‘book’ elements.
The result is a collection of ‘title’ elements, which we iterate over and print their text content.
2. Modifying Existing XML
Ruby allows us to modify existing XML documents by adding, removing, or updating elements and attributes.
Consider the following XML structure:
xml <catalog> <book id="1"> <title>Book 1</title> <author>Author 1</author> </book> <book id="2"> <title>Book 2</title> <author>Author 2</author> </book> </catalog>
To update an attribute and add a new element to this XML document, we can use the following code:
ruby require 'rexml/document' # Read XML file file = File.read('data.xml') xml = REXML::Document.new(file) # Find the book with id=2 book = REXML::XPath.first(xml, '//book[@id="2"]') # Update an attribute book.attributes['id'] = 3 # Add a new element book.add_element('price').text = '29.99' # Write the modified XML to a file File.open('output.xml', 'w') do |file| file.write(xml.to_s) End
In the code snippet above, we first read the XML file and parse it into an XML document. We then use XPath to find the book element with an id attribute equal to 2 using REXML::XPath.first. We can update the attribute value by assigning a new value to it using book.attributes[‘id’] = 3.
To add a new element, we use book.add_element(‘price’).text = ‘29.99’. Here, we add a ‘price’ element with the text content set to ‘29.99’.
Finally, we write the modified XML document to a file.
3. Transforming XML with XSLT
XSLT (eXtensible Stylesheet Language Transformations) is a powerful language used to transform XML documents into different structures or formats. Ruby provides support for XSLT transformations through the ‘nokogiri’ gem.
To perform an XSLT transformation on an XML document, we need an XSLT stylesheet. Let’s consider the following XSLT stylesheet, ‘transform.xsl’:
xslt <xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> <xsl:output method="xml" indent="yes"/> <xsl:template match="/"> <catalog> <xsl:for-each select="//book"> <book> <title> <xsl:value-of select="title"/> </title> <author> <xsl:value-of select="author"/> </author> </book> </xsl:for-each> </catalog> </xsl:template> </xsl:stylesheet>
This stylesheet transforms an XML document by extracting book elements and their corresponding title and author elements.
To perform the transformation in Ruby, we can use the following code:
ruby require 'nokogiri' # Read XML file file = File.read('data.xml') xml = Nokogiri::XML(file) # Read XSLT file stylesheet = File.read('transform.xsl') xslt = Nokogiri::XSLT(stylesheet) # Perform the transformation result = xslt.transform(xml) # Print the transformed XML puts result.to_xml
In the code snippet above, we first read the XML and XSLT files. We parse the XML document using Nokogiri::XML and create an XSLT object using Nokogiri::XSLT with the XSLT stylesheet.
We then perform the transformation by calling xslt.transform on the XML document. The result is an XML object representing the transformed XML.
Finally, we can print the transformed XML using result.to_xml.
Conclusion
In this comprehensive guide, we have explored the power and versatility of Ruby functions for XML parsing and generation. We learned how to leverage the ‘REXML’ library to parse and navigate XML trees effectively, handle XML attributes, and generate XML documents programmatically.
Additionally, we delved into more advanced techniques, such as performing XPath queries to extract specific XML elements, modifying existing XML documents by adding, updating, or removing elements and attributes, and transforming XML using XSLT with the ‘nokogiri’ gem.
With this knowledge, you can confidently work with XML data using Ruby, manipulate XML structures efficiently, and automate XML-related tasks in your projects. The flexibility and simplicity of Ruby’s XML functions make it a valuable tool in your programming arsenal.
So go ahead, apply what you’ve learned, and unlock the full potential of Ruby for XML parsing and generation!
Table of Contents
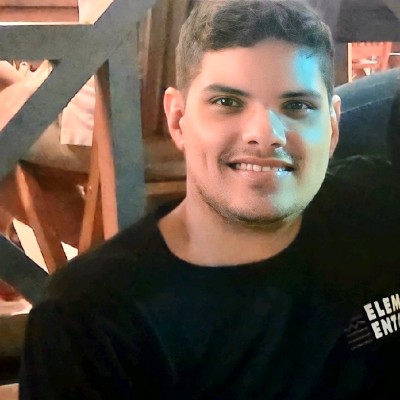
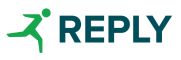