10 Ruby Gems for API Development
Ruby is a powerful and elegant programming language, known for its simplicity and productivity. It has gained immense popularity in web development, particularly for building APIs (Application Programming Interfaces). APIs play a vital role in modern software development, enabling seamless communication and data exchange between different applications. To expedite and enhance API development, the Ruby community has developed numerous gems. In this blog, we will explore the ten essential Ruby gems that can significantly boost your API development journey.
Table of Contents
1. Grape
Grape is a flexible and RESTful API micro-framework for Ruby, designed to make API development straightforward and efficient. It is built on top of Rack and provides a concise DSL (Domain-Specific Language) for defining API endpoints, versioning, and parameter validation. Grape excels at building scalable APIs and offers support for various formats such as JSON and XML.
Installation:
ruby gem install grape
Usage:
ruby require 'grape' class MyAPI < Grape::API format :json get '/hello' do { message: 'Hello, World!' } end end
2. Jbuilder
Jbuilder is a gem that simplifies JSON object creation in Ruby. It provides a clean and expressive DSL to generate complex JSON structures from Ruby objects. With Jbuilder, you can easily format your API responses and handle relationships between models, making it an excellent choice for API view rendering.
Installation:
ruby gem install jbuilder
Usage:
ruby require 'jbuilder' json = Jbuilder.new do |json| json.name 'John Doe' json.email 'john@example.com' json.address do json.city 'New York' json.zip_code '10001' end end puts json.target!
3. Rack CORS
Cross-Origin Resource Sharing (CORS) is a crucial aspect of modern web applications, allowing secure cross-origin requests. Rack CORS is a gem that helps you handle CORS-related issues in your Ruby API. It enables you to specify which origins are allowed to access your API and which HTTP methods are supported.
Installation:
ruby gem install rack-cors
Configuration:
ruby # In config.ru require 'rack/cors' use Rack::Cors do allow do origins '*' resource '*', headers: :any, methods: %i[get post put delete options] end end
4. ActiveModel::Serializers
ActiveModel::Serializers is a powerful gem that provides a simple and customizable way to serialize Ruby objects into JSON or XML for API responses. It works seamlessly with Rails and offers various options to control the serialization process, such as associations, includes, and root elements.
Installation:
ruby gem 'active_model_serializers'
Configuration:
ruby # In config/application.rb config.active_model_serializers = { default_serializer: MyDefaultSerializer, adapter: :json_api } Usage: ruby Copy code # app/serializers/user_serializer.rb class UserSerializer < ActiveModel::Serializers::Model attributes :id, :name, :email end
5. Rack-Attack
Security is a primary concern when exposing APIs to the internet. Rack-Attack is a gem that provides throttling and blocking capabilities to protect your API from abusive requests and potential DDoS attacks. It allows you to define custom rules to limit access based on IP addresses, request rates, or other criteria.
Installation:
ruby gem install rack-attack
Configuration:
ruby # In config/application.rb config.middleware.use Rack::Attack
Usage:
ruby # In config/initializers/rack_attack.rb Rack::Attack.throttle('req/ip', limit: 100, period: 1.hour) do |req| req.ip end
6. Versionist
As your API evolves, you may need to handle different versions of your endpoints. Versionist is a gem that simplifies API versioning in Ruby, making it easier to maintain backward compatibility while introducing new features.
Installation:
ruby gem 'versionist'
Usage:
ruby # In config/routes.rb MyAPI::Application.routes.draw do api_version(module: 'V1', header: 'Accept', value: 'application/vnd.myapi.v1+json', default: true) do resources :products end api_version(module: 'V2', header: 'Accept', value: 'application/vnd.myapi.v2+json') do resources :products, only: [:index, :show] end end
7. Faraday
Faraday is a flexible and widely used HTTP client library that allows you to make HTTP requests from your Ruby API. It provides a simple and consistent interface to interact with various APIs and supports multiple adapters like Net::HTTP and Typhoeus.
Installation:
ruby gem 'faraday'
Usage:
ruby require 'faraday' response = Faraday.get('https://api.example.com/users') do |req| req.headers['Authorization'] = 'Bearer YOUR_ACCESS_TOKEN' req.params['page'] = 1 end puts response.body
8. ActiveModel::Serializers::JSON
ActiveModel::Serializers::JSON is an extension of ActiveModel::Serializers that optimizes the JSON serialization process. It delivers better performance compared to the default serialization in Rails, especially when dealing with large datasets.
Installation:
ruby gem 'active_model_serializers-json'
Usage:
ruby # In config/application.rb config.active_model_serializers.jsonapi_pagination = :paged
9. Gibbon
Gibbon is a gem that facilitates seamless integration with the Mailchimp API, allowing you to manage your email campaigns and subscribers easily. It provides a straightforward interface for sending and tracking emails, making it a popular choice for email marketing applications.
Installation:
ruby gem 'gibbon'
Usage:
ruby require 'gibbon' gibbon = Gibbon::Request.new(api_key: 'YOUR_MAILCHIMP_API_KEY') gibbon.lists.retrieve
10. Rails::API
Rails::API is a slimmed-down version of Ruby on Rails, specifically designed for building APIs. It removes unnecessary middleware and functionalities to keep your API lightweight and fast. If your primary focus is on API development and you don’t need the full features of Rails, Rails::API is an excellent option.
Installation:
ruby gem 'rails-api'
Usage:
ruby class MyAPI < Rails::API get '/hello' do { message: 'Hello, API World!' } end end
Conclusion
Ruby’s vibrant ecosystem of gems plays a crucial role in simplifying and enhancing API development. The ten gems we explored in this blog cover a wide range of functionalities, including API routing, serialization, security, versioning, and external API integration. Incorporating these gems into your Ruby API project will undoubtedly streamline your development process and help you deliver robust and efficient APIs.
Remember, the key to effective API development is understanding your project requirements and selecting the most suitable gems that align with your goals. Happy coding!
Table of Contents
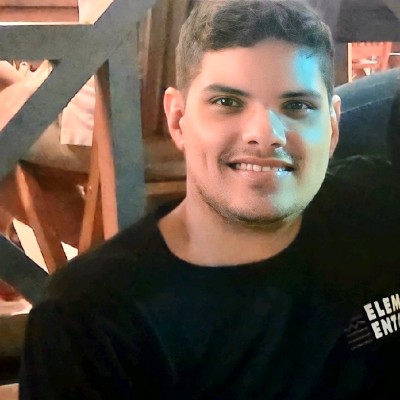
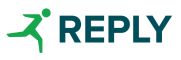