10 Ruby Gems for Caching and Performance Optimization
In the fast-paced world of web development, performance is a crucial factor that can make or break your application’s success. Slow-loading pages and high server loads can lead to dissatisfied users and lost opportunities. Fortunately, Ruby developers have a powerful arsenal of tools at their disposal to tackle these performance challenges. In this blog, we’ll explore 10 exceptional Ruby Gems that can help you optimize your application’s performance through caching and various optimization techniques. From reducing database queries to caching expensive operations, these Gems can significantly improve your app’s speed and responsiveness.
Table of Contents
1. Dalli:
Dalli is a popular Ruby Gem that provides a robust interface to connect your Ruby application with the Memcached caching system. Memcached is an in-memory caching system that stores key-value pairs to alleviate the load on your database and speed up access to frequently used data. Dalli’s efficient API and native support for Rails make it a top choice for caching your app’s data.
Code Sample:
ruby # Connect to Memcached server cache = Dalli::Client.new('localhost:11211') # Store data in cache cache.set('user:123', { name: 'John Doe', email: 'john@example.com' }) # Retrieve data from cache user_data = cache.get('user:123')
2. Redis-Rails:
Redis-Rails is a powerful Gem that integrates Redis, an in-memory data structure store, with Ruby on Rails applications. Redis allows you to cache data, manage session storage, and create queues for background processing. Its speed and flexibility make it an excellent choice for caching frequently accessed data and reducing database queries.
Code Sample:
ruby # Configure Redis connection in config/initializers/redis.rb $redis = Redis.new(host: 'localhost', port: 6379) # Store data in Redis cache $redis.set('product:123', { name: 'Widget', price: 19.99 }) # Retrieve data from Redis cache product_data = $redis.get('product:123')
3. ActiveSupport::Cache:
ActiveSupport::Cache is a core component of Ruby on Rails that provides a unified caching interface. It supports various caching stores like Memcached, Redis, and file-based caching. It enables you to cache data, fragments, and even query results, improving performance across the application.
Code Sample:
ruby # Configure caching store in config/environments/development.rb config.cache_store = :mem_cache_store, 'localhost:11211' # Store data in cache Rails.cache.write('user:123', { name: 'Jane Smith', email: 'jane@example.com' }) # Retrieve data from cache user_data = Rails.cache.read('user:123')
4. Rack::Cache:
Rack::Cache is a middleware that provides HTTP caching for Ruby web applications. It works as an intermediary between the web server and your app, allowing you to cache responses based on their HTTP headers. By caching responses at the server level, Rack::Cache reduces the load on your application and significantly improves response times.
Code Sample:
ruby # Add Rack::Cache middleware in config.ru require 'rack/cache' use Rack::Cache # Define caching options in your app class MyApp < Sinatra::Base set :static_cache_control, [:public, max_age: 3600] set :dynamic_cache_control, [:public, max_age: 600] end
5. Cache Money:
Cache Money is a Ruby Gem specifically designed for caching Active Record models. It integrates with various caching stores, such as Memcached and Redis, and allows you to cache model objects and queries effectively. By reducing the number of database queries, Cache Money can significantly improve the performance of your Rails applications.
Code Sample:
ruby # Install Cache Money and configure caching store class Product < ActiveRecord::Base acts_as_cached version: 1, expires_in: 1.day end # Cache a model query result products = Product.cached { Product.where(category: 'Electronics') }
6. IdentityCache:
IdentityCache is another fantastic Gem tailored for caching Active Record models. It stores model objects in a compact format, minimizing memory usage while ensuring fast retrieval. IdentityCache is easy to integrate with existing Rails applications and can be a game-changer in improving query performance.
Code Sample:
ruby # Configure IdentityCache for a model class Product < ActiveRecord::Base include IdentityCache cache_index :category, :name end # Fetch data from cache products = Product.fetch_by_category('Electronics')
7. Cashier:
Cashier is a lightweight Ruby Gem that provides a straightforward caching solution for any Ruby application. It supports caching to files, Redis, and other stores. Its simplicity makes it a popular choice for developers seeking quick and easy caching implementation.
Code Sample:
ruby # Configure Cashier caching store Cashier::Store.config.cache_store = Cashier::RedisStore.new(redis: Redis.new) # Cache data with Cashier Cashier::Store.write('user:456', { name: 'Bob Johnson', email: 'bob@example.com' }) # Retrieve data from Cashier cache user_data = Cashier::Store.read('user:456')
8. Readthis:
Readthis is a high-performance caching Gem that utilizes Redis as its caching store. It offers various features like object marshaling, cache expiration, and automatic namespace management. Readthis is an excellent choice for caching in both Rails and non-Rails applications.
Code Sample:
ruby # Configure Readthis caching store Readthis::Cache.new(expires_in: 1.day, redis: Redis.new) # Cache data using Readthis Readthis.cache.write('order:789', { total: 100.0, status: 'processing' }) # Retrieve data from Readthis cache order_data = Readthis.cache.read('order:789')
9. Http::Cache:
Http::Cache is a Ruby Gem designed to add HTTP caching support to your applications. It caches HTTP responses based on their headers, effectively reducing the number of requests and improving the overall user experience.
Code Sample:
ruby # Configure Http::Cache middleware use Http::Cache # Define caching options for your app class MyApp < Sinatra::Base set :static_cache_control, [:public, max_age: 3600] set :dynamic_cache_control, [:public, max_age: 600] end
10. Rails Cache Digests:
Rails Cache Digests is a built-in feature in Ruby on Rails that enables you to cache fragments of your views. It utilizes the fragment’s name and its dependencies to generate a unique cache key, ensuring efficient cache management. By caching only the necessary parts of your views, you can significantly reduce rendering time and enhance performance.
Code Sample:
ruby # Cache a fragment in your view <% cache('products_index') do %> <% @products.each do |product| %> <div class="product"> <h2><%= product.name %></h2> <p><%= product.description %></p> </div> <% end %> <% end %>
Conclusion
Performance optimization is a critical aspect of building successful web applications, and these 10 Ruby Gems offer invaluable tools to achieve that goal. From in-memory caching with Dalli and Redis-Rails to Rails-specific caching with ActiveSupport::Cache and Cache Money, each Gem caters to specific caching needs. Additionally, Rack::Cache and Http::Cache take care of HTTP caching, significantly reducing server load.
By integrating these Gems into your Ruby applications, you can create blazing-fast, responsive, and efficient web experiences that keep your users engaged and satisfied. Embrace the power of caching and performance optimization with these fantastic Ruby Gems!
Table of Contents
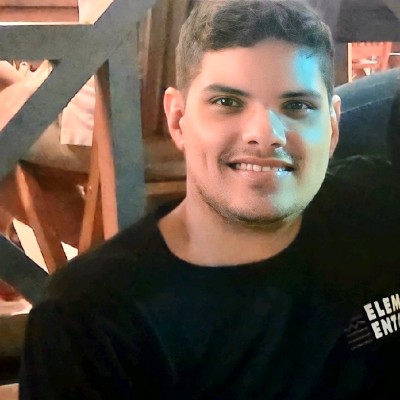
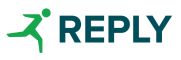