10 Ruby Gems for Chatbot Development
Chatbots have become an integral part of modern applications, enhancing user interactions and automating customer support. Ruby, a versatile programming language, offers a variety of gems (libraries) that can simplify chatbot development. In this blog, we’ll explore 10 Ruby Gems that can help you create powerful and efficient chatbots. Whether you’re a seasoned Ruby developer or just getting started, these gems will make your chatbot development journey smoother and more enjoyable.
Table of Contents
Why Use Ruby for Chatbot Development?
Before we delve into the Ruby Gems, let’s briefly discuss why Ruby is a great choice for chatbot development. Ruby is known for its simplicity and readability, making it an excellent language for beginners and experienced developers alike. It has a vibrant community and a wealth of open-source libraries that can accelerate development.
Ruby’s elegance and expressiveness also make it suitable for crafting chatbots with natural language processing (NLP) capabilities. Additionally, Ruby-on-Rails, a popular web framework, integrates seamlessly with these chatbot gems, making it easier to build web-based chatbot applications.
Now, let’s dive into the world of Ruby Gems for chatbot development.
1. ChatterBot
GitHub Repository: ChatterBot
ChatterBot is a versatile and easy-to-use Ruby Gem for creating chatbots. It provides an intuitive interface for training your chatbot using pre-existing datasets or custom data. ChatterBot supports various machine learning algorithms for natural language understanding, making it suitable for building chatbots with advanced NLP capabilities.
ruby # Example of using ChatterBot require 'chatterbot' bot = ChatterBot::Bot.new bot.train("What's your name?", "I'm a chatbot.") response = bot.get_response("What's your name?") puts response
2. Telegram Bot Ruby
GitHub Repository: Telegram Bot Ruby
Telegram is a popular messaging platform, and if you want to create chatbots for Telegram, this gem is a must-have. Telegram Bot Ruby simplifies the process of building Telegram bots by providing an easy-to-use API wrapper. You can create interactive bots with minimal effort, making it perfect for developing chatbots that engage users within the Telegram ecosystem.
ruby # Example of using Telegram Bot Ruby require 'telegram/bot' Telegram::Bot::Client.run('YOUR_TELEGRAM_API_KEY') do |bot| bot.listen do |message| case message.text when '/start' bot.api.send_message(chat_id: message.chat.id, text: 'Hello, I am your Telegram bot!') end end end
3. Wit-Ruby
GitHub Repository: Wit-Ruby
Wit.ai is a powerful NLP platform developed by Facebook. Wit-Ruby is a gem that allows you to integrate Wit.ai into your Ruby chatbot projects seamlessly. With Wit.ai, you can easily add NLP capabilities to your chatbot, making it smarter and more context-aware.
ruby # Example of using Wit-Ruby with Wit.ai require 'wit' access_token = 'YOUR_WIT_AI_ACCESS_TOKEN' client = Wit.new(access_token: access_token) response = client.message('Tell me a joke') puts response
4. Messenger-Ruby
GitHub Repository: Messenger-Ruby
If you’re looking to create chatbots for Facebook Messenger, Messenger-Ruby is the gem for you. It simplifies the integration process by providing a straightforward API for building Messenger bots. You can easily handle user interactions, send messages, and create interactive chat experiences for Messenger users.
ruby # Example of using Messenger-Ruby for Facebook Messenger bot require 'messenger-ruby' Messenger::Bot.subscribe('YOUR_FACEBOOK_PAGE_TOKEN') Messenger::Bot.on :message do |message| message.reply(text: 'Hello, I am your Facebook Messenger bot!') end Messenger::Bot.deliver( recipient: 'USER_ID', message: { text: 'This is a message from your bot.' } )
5. Dialogflow Ruby
GitHub Repository: Dialogflow Ruby
Google’s Dialogflow is a popular platform for building conversational interfaces, and Dialogflow Ruby allows you to integrate Dialogflow into your Ruby applications effortlessly. With this gem, you can create chatbots that understand and respond to user queries using Dialogflow’s powerful NLP capabilities.
ruby # Example of using Dialogflow Ruby with Dialogflow require 'google/cloud/dialogflow' session_id = 'YOUR_SESSION_ID' project_id = 'YOUR_PROJECT_ID' session_client = Google::Cloud::Dialogflow.sessions session = session_client.session_path(project_id, session_id) query_input = { text: { text: 'Tell me the weather' } } response = session_client.detect_intent(session, query_input) puts response.query_result.fulfillment_text
6. Rasa Ruby SDK
GitHub Repository: Rasa Ruby SDK
Rasa is an open-source platform for building AI-powered chatbots, and the Rasa Ruby SDK enables you to interact with Rasa chatbots from your Ruby applications. With Rasa, you can create sophisticated chatbots with contextual understanding, and the Ruby SDK makes it easy to integrate them into your projects.
ruby # Example of using Rasa Ruby SDK require 'rasa' rasa = Rasa.new('http://localhost:5005') response = rasa.interpret('Tell me a joke') puts response['text']
7. Slack-Ruby-Bot
GitHub Repository: Slack-Ruby-Bot
If you’re building chatbots for Slack, Slack-Ruby-Bot is a fantastic gem to have in your toolbox. It simplifies the process of creating Slack bots and allows you to respond to messages, trigger actions, and create interactive Slack bot experiences.
ruby # Example of using Slack-Ruby-Bot require 'slack-ruby-bot' class MyBot < SlackRubyBot::Bot command 'hello' do |client, data, match| client.say(channel: data.channel, text: "Hello, @#{data.user}!") end end MyBot.run
8. Stanford NLP Ruby
GitHub Repository: Stanford NLP Ruby
Stanford NLP Ruby is a gem that allows you to leverage the Stanford NLP library in your Ruby chatbots. This gem provides a bridge between Ruby and the Stanford NLP library, enabling you to perform various NLP tasks, such as tokenization, named entity recognition, and sentiment analysis.
ruby # Example of using Stanford NLP Ruby require 'stanford-core-nlp' text = 'I love Ruby chatbot development!' pipeline = StanfordCoreNLP.load result = pipeline.annotate(text) puts result.get(:sentiment)
9. Twitter Ruby Gem
GitHub Repository: Twitter Ruby Gem
If you’re building a chatbot that interacts with Twitter, the Twitter Ruby Gem is a valuable tool. It simplifies the process of accessing Twitter’s API, allowing you to create bots that can tweet, follow users, and retrieve tweets.
ruby # Example of using the Twitter Ruby Gem require 'twitter' client = Twitter::REST::Client.new do |config| config.consumer_key = 'YOUR_CONSUMER_KEY' config.consumer_secret = 'YOUR_CONSUMER_SECRET' config.access_token = 'YOUR_ACCESS_TOKEN' config.access_token_secret = 'YOUR_ACCESS_TOKEN_SECRET' end client.update('Hello, Twitter! #RubyChatbot')
10. Nokogiri
GitHub Repository: Nokogiri
Nokogiri is a powerful gem for parsing and manipulating HTML and XML documents. While it may not be a chatbot-specific gem, it’s incredibly useful when your chatbot needs to scrape information from web pages or interact with websites to gather data.
ruby # Example of using Nokogiri for web scraping in a chatbot require 'nokogiri' require 'open-uri' url = 'https://example.com' document = Nokogiri::HTML(URI.open(url)) puts document.title
Conclusion
These 10 Ruby Gems open up a world of possibilities for chatbot development. Whether you’re creating chatbots for messaging platforms like Telegram and Facebook Messenger or integrating powerful NLP capabilities using platforms like Wit.ai and Dialogflow, these gems can significantly streamline your development process.
Choose the gems that align with your project’s requirements and start building intelligent and interactive chatbots with Ruby. Remember, the Ruby community is vast, and you’ll find plenty of resources and support as you embark on your chatbot development journey. Happy coding!
Table of Contents
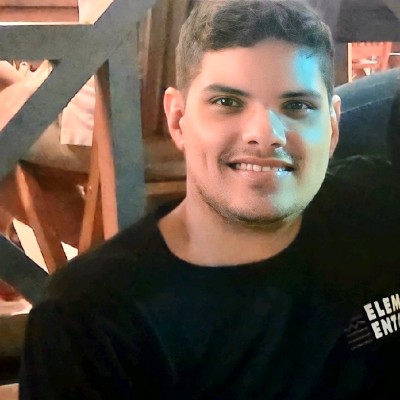
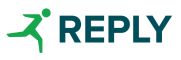