10 Ruby Gems for Frontend Development
Ruby, known for its elegance and readability, is not just limited to backend development. With the right set of Ruby Gems, frontend development becomes a breeze, allowing you to create captivating web interfaces effortlessly. In this blog, we will delve into 10 Ruby Gems that are invaluable assets for frontend developers. These Gems cover a wide range of areas, including CSS frameworks, asset management, testing tools, and more. By incorporating these Gems into your workflow, you can optimize your development process, streamline code organization, and deliver exceptional user experiences. Whether you’re a seasoned Ruby developer or just getting started with frontend development, this comprehensive guide will introduce you to essential Gems that will boost your productivity and elevate your web development projects.
1. SASS-Rails: Supercharge CSS Development with SASS
SASS-Rails is a Ruby Gem that seamlessly integrates the power of the SASS (Syntactically Awesome Style Sheets) preprocessor with the Rails asset pipeline. It empowers frontend developers to write more maintainable and modular CSS code using variables, mixins, and nested selectors. Let’s see a code snippet to understand its usage:
ruby # app/assets/stylesheets/application.scss @import 'variables'; @import 'layout'; @import 'components';
By splitting the CSS code into separate files and using @import directives, SASS-Rails helps organize stylesheets efficiently. Additionally, it offers features like variable scoping, inheritance, and functions that enable code reuse and promote clean architecture.
2. Bourbon: A Lightweight and Modular CSS Framework
Bourbon is a lightweight and modular CSS framework that brings a plethora of useful mixins to your frontend development arsenal. It focuses on simplicity and provides a robust set of cross-browser mixins for common CSS tasks. Let’s take a look at an example:
scss // app/assets/stylesheets/application.scss @import 'bourbon'; .my-element { @include border-radius(5px); @include box-shadow(0 2px 4px rgba(0, 0, 0, 0.1)); // ... }
Bourbon mixins such as border-radius and box-shadow abstract away the complexities of vendor prefixes and browser inconsistencies, allowing you to write concise and clean CSS code. The modular nature of Bourbon also lets you include only the mixins you need, keeping your stylesheets lightweight and optimized.
3. Slim: Clean and Elegant Template Engine for HTML
Slim is a clean and elegant template engine for HTML that aims to reduce the verbosity of traditional HTML markup. With its concise syntax and indentation-based structure, Slim allows you to write HTML templates quickly and efficiently. Consider the following example:
slim / app/views/posts/index.html.slim h1 Posts ul - @posts.each do |post| li = link_to post.title, post_path(post)
In this snippet, we use Slim’s syntax to generate an unordered list of posts with their respective links. The indentation-based structure eliminates the need for opening and closing tags, resulting in more readable and maintainable code.
4. Simple Form: Streamline Form Building Process
Building forms in Ruby on Rails can be a repetitive and time-consuming task. Simple Form is a Ruby Gem that simplifies form building by providing a higher-level DSL (Domain-Specific Language) to generate form markup. Let’s see a code example:
ruby # app/views/posts/_form.html.erb <%= simple_form_for @post do |f| %> <%= f.input :title %> <%= f.input :content, as: :text %> <%= f.button :submit %> <% end %>
With Simple Form, you can define form fields using f.input, which automatically generates the appropriate HTML markup based on the field type. It also offers numerous customization options, such as input validation, form styling, and error handling.
5. RSpec-Rails: Behavior-Driven Development for Rails
RSpec-Rails is a Ruby Gem that enables behavior-driven development (BDD) testing in Ruby on Rails applications. It provides a domain-specific language for writing expressive and readable tests, allowing you to define the expected behavior of your application’s components. Let’s take a look at an example:
ruby # spec/models/post_spec.rb RSpec.describe Post, type: :model do it "is valid with a title and content" do post = Post.new(title: "Hello, World!", content: "Lorem ipsum...") expect(post).to be_valid end it "is invalid without a title" do post = Post.new(content: "Lorem ipsum...") expect(post).not_to be_valid end # ... end
In this snippet, we define RSpec examples using the it syntax, making the expected behavior explicit. RSpec-Rails provides a wide range of matchers and testing utilities that facilitate comprehensive testing of your Rails models, controllers, and views.
6. Capybara: Integration Testing Made Easy
Capybara is a Ruby Gem that simplifies integration testing in Ruby on Rails applications. It provides a clean and intuitive DSL for interacting with web pages, simulating user actions, and verifying expected outcomes. Consider the following code:
ruby # spec/features/post_spec.rb RSpec.feature "Posts", type: :feature do scenario "User creates a new post" do visit new_post_path fill_in "Title", with: "Hello, World!" fill_in "Content", with: "Lorem ipsum..." click_button "Create Post" expect(page).to have_text("Post successfully created.") expect(page).to have_content("Hello, World!") end # ... end
In this example, Capybara enables us to simulate a user creating a new post by filling in form fields, clicking a button, and asserting the expected content on the resulting page. Capybara’s expressive syntax and powerful assertions make it an essential tool for frontend integration testing.
7. Webpacker: Advanced JavaScript and Asset Management
Webpacker is a Ruby Gem that brings the power of Webpack to Ruby on Rails applications. It simplifies JavaScript and asset management, allowing you to bundle, transpile, and optimize frontend assets efficiently. Let’s see how Webpacker can be used:
javascript // app/javascript/packs/application.js import 'bootstrap/dist/js/bootstrap'; // ...
In this example, we import the JavaScript functionality of the Bootstrap framework using the ES6 import syntax. Webpacker automatically handles the bundling and includes the necessary dependencies in the final output. With Webpacker, you can also leverage advanced features like code splitting, hot module replacement, and tree shaking to optimize frontend performance.
8. Bootstrap: Responsive and Feature-Rich CSS Framework
Bootstrap, a widely popular CSS framework, provides a rich set of pre-built components and utilities for frontend development. The Bootstrap Ruby Gem integrates this powerful framework seamlessly with Ruby on Rails applications. Let’s look at an example:
scss // app/assets/stylesheets/application.scss @import 'bootstrap';
By importing the Bootstrap stylesheets, you gain access to a wide range of responsive grids, typography, buttons, navigation bars, and more. This saves you time and effort in building commonly used UI components from scratch. The Bootstrap Gem also provides additional functionality like Sass variables for customization, JavaScript plugins, and easy integration with other Gems like Simple Form.
9. Font Awesome: Iconic Font and CSS Toolkit
Font Awesome is an iconic font and CSS toolkit that provides a vast library of scalable vector icons. The Font Awesome Ruby Gem allows you to effortlessly integrate these icons into your Ruby on Rails application. Here’s an example:
erb <!-- app/views/posts/show.html.erb --> <%= fa_icon 'star', class: 'text-warning' %>
In this snippet, we use the fa_icon helper to display a star icon from the Font Awesome library. The class option allows us to style the icon using Bootstrap classes or custom CSS. Font Awesome offers a comprehensive collection of icons for various use cases, making it an excellent choice for enhancing your application’s visual appeal.
10. Guard: Automate Development Workflows and Test Execution
Guard is a Ruby Gem that automates development workflows and provides continuous testing capabilities. It monitors file changes and triggers predefined actions, such as running tests, reloading the browser, or compiling assets, in response to those changes. Let’s see an example:
ruby # Guardfile guard :rspec, cmd: "bundle exec rspec -f doc" do watch(%r{^spec/.+_spec\.rb$}) watch(%r{^app/(.+)\.rb$}) { |m| "spec/#{m[1]}_spec.rb" } watch(%r{^lib/(.+)\.rb$}) { |m| "spec/lib/#{m[1]}_spec.rb" } # ... end
In this Guardfile configuration, we define a guard for RSpec that runs our tests whenever relevant files are modified. Guard saves development time by eliminating the need to manually run test suites or restart development servers, thereby providing a seamless and efficient development experience.
Conclusion
Frontend development with Ruby on Rails becomes even more powerful and enjoyable when you incorporate the right Ruby Gems into your workflow. In this blog, we explored 10 essential Gems for frontend development, ranging from CSS frameworks to testing tools. These Gems offer features like CSS pre-processing, modular CSS mixins, efficient HTML templating, simplified form building, behavior-driven testing, integration testing, asset management, UI component libraries, iconic fonts, and development automation. By leveraging these Gems, you can optimize your frontend development process, enhance code maintainability, and deliver exceptional user experiences. Whether you’re a beginner or an experienced developer, integrating these Gems into your Ruby on Rails projects will undoubtedly boost your productivity and make frontend development a delightful experience. So go ahead, explore these Gems, and supercharge your frontend development endeavors!
Table of Contents
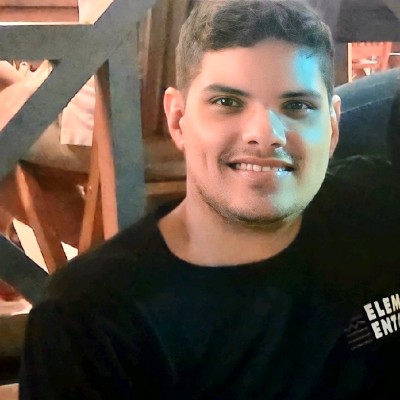
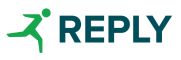