10 Ruby Gems for Machine Learning
Machine learning has gained tremendous popularity in recent years, and for good reason. It allows us to create intelligent systems that can make predictions, classify data, and even generate creative content. While Python is often the language of choice for machine learning, Ruby enthusiasts need not feel left out. In this blog, we’ll introduce you to 10 Ruby Gems that can empower your machine learning endeavors. These Gems cover various aspects of the machine learning pipeline, from data preprocessing to model evaluation. Whether you’re a seasoned Rubyist or a newcomer to the language, these Gems will help you get started with machine learning in Ruby.
Table of Contents
1. Daru
Data Handling Made Easy
Before you dive into the world of machine learning, you’ll need to handle and preprocess your data efficiently. Daru is a Ruby library that simplifies the process of working with structured data. It provides powerful data manipulation capabilities similar to pandas in Python. With Daru, you can load, transform, and analyze your data effortlessly.
Installation
You can install Daru using RubyGems:
ruby gem install daru
Example Usage
ruby require 'daru' # Create a DataFrame df = Daru::DataFrame.new({ 'Age' => [25, 30, 35, 40, 45], 'Income' => [50000, 60000, 75000, 80000, 90000] }) # Perform operations on the DataFrame mean_age = df['Age'].mean max_income = df['Income'].max puts "Mean Age: #{mean_age}" puts "Max Income: #{max_income}"
Daru’s intuitive API makes it a valuable tool for data exploration and manipulation, essential for any machine learning project.
2. SciRuby
Scientific Computing with Ruby
SciRuby is a comprehensive ecosystem of scientific and numerical libraries for Ruby. It includes tools for linear algebra, statistics, optimization, and more. While not exclusively for machine learning, SciRuby provides the foundational building blocks that are essential for developing machine learning algorithms.
Installation
You can install SciRuby using RubyGems:
ruby gem install sciruby
Example Usage
ruby require 'sciruby' # Create a matrix matrix = Numo::DFloat.new(3, 3).seq # Perform matrix operations inverse_matrix = matrix.inv puts "Inverse Matrix:\n#{inverse_matrix}"
By leveraging SciRuby, you can perform advanced numerical computations and build custom machine learning algorithms in Ruby.
3. Numo-NArray
Numerical Computing in Ruby
Numo-NArray is another essential Gem for numerical computing in Ruby. It provides a powerful N-dimensional array class that is efficient and easy to use. This Gem is particularly handy when you need to work with multi-dimensional data, which is common in machine learning.
Installation
You can install Numo-NArray using RubyGems:
ruby gem install numo-narray
Example Usage
ruby require 'numo/narray' # Create a 2D array array = Numo::DFloat[[1, 2, 3], [4, 5, 6], [7, 8, 9]] # Perform array operations sum = array.sum mean = array.mean puts "Sum: #{sum}" puts "Mean: #{mean}"
Numo-NArray’s efficient numerical operations make it an indispensable tool for implementing machine learning algorithms.
4. Rblearn
Machine Learning in Ruby
Rblearn is a Ruby library that provides machine learning algorithms for classification and regression tasks. It is built on top of Liblinear and Libsvm, two popular C libraries for machine learning. Rblearn offers a simple and convenient API for training and using these algorithms in your Ruby projects.
Installation
You can install Rblearn using RubyGems:
ruby gem install rblearn
Example Usage
ruby require 'rblearn' # Create a dataset X = [[1, 2], [2, 3], [3, 4], [4, 5]] y = [0, 1, 0, 1] # Create a model and train it model = Rblearn::SVM.new model.train(X, y) # Make predictions predictions = model.predict([[5, 6], [6, 7]]) puts "Predictions: #{predictions}"
Rblearn simplifies the process of incorporating machine learning into your Ruby applications, making it accessible even for those with limited machine learning experience.
5. Rubystats
Statistical Analysis with Ruby
Rubystats is a Gem that provides a wide range of statistical functions and distributions for Ruby. While not specifically designed for machine learning, it can be incredibly useful when you need to perform statistical analysis on your data, a crucial step in the machine learning pipeline.
Installation
You can install Rubystats using RubyGems:
ruby gem install rubystats
Example Usage
ruby require 'rubystats' # Generate a random sample from a normal distribution normal_dist = Rubystats::NormalDistribution.new(0, 1) sample = normal_dist.rng(10) # Calculate the mean and standard deviation mean = Rubystats.mean(sample) std_dev = Rubystats.stddev(sample) puts "Mean: #{mean}" puts "Standard Deviation: #{std_dev}"
Rubystats allows you to perform various statistical analyses, helping you gain insights into your data before building machine learning models.
6. NMatrix
Advanced Linear Algebra
NMatrix is a Ruby library that provides a high-performance N-dimensional array class, specifically designed for scientific and numerical computing. It is a powerful tool for handling large datasets and performing complex linear algebra operations efficiently.
Installation
You can install NMatrix using RubyGems:
ruby gem install nmatrix
Example Usage
ruby require 'nmatrix' # Create a 2D matrix matrix = NMatrix.new([3, 3], [1, 2, 3, 4, 5, 6, 7, 8, 9]) # Perform matrix multiplication result = matrix.dot(matrix) puts "Matrix Multiplication:\n#{result}"
NMatrix’s high-performance capabilities make it an excellent choice for machine learning tasks that involve large datasets and complex mathematical operations.
7. Kramati
Data Preprocessing Made Easy
Kramati is a Ruby Gem designed to simplify data preprocessing tasks. In machine learning, data preprocessing is a critical step that involves cleaning, transforming, and scaling your data. Kramati provides a convenient API to perform these tasks efficiently.
Installation
You can install Kramati using RubyGems:
ruby gem install kramati
Example Usage
ruby require 'kramati' # Create a data preprocessing pipeline pipeline = Kramati::Pipeline.new do add Kramati::Imputer.new(strategy: 'mean') add Kramati::Scaler.new end # Fit and transform the data preprocessed_data = pipeline.fit_transform(X) puts "Preprocessed Data:\n#{preprocessed_data}"
Kramati simplifies the often cumbersome process of data preprocessing, allowing you to focus on building and training machine learning models.
8. Ai4r
Ruby AI Library
Ai4r (Artificial Intelligence for Ruby) is a library that provides a wide range of machine learning algorithms, including decision trees, neural networks, and genetic algorithms. It offers an easy-to-use interface for creating and training these algorithms for various tasks.
Installation
You can install Ai4r using RubyGems:
ruby gem install ai4r
Example Usage
ruby require 'ai4r' # Create a decision tree classifier tree = Ai4r::Classifiers::ID3.new tree.build(X, y) # Make predictions predictions = tree.eval([5, 6]) puts "Predictions: #{predictions}"
Ai4r is a versatile Gem that allows you to experiment with different machine learning algorithms in Ruby.
9. MLBG
Machine Learning with Gradient Boosting
MLBG is a Ruby Gem that provides an implementation of gradient boosting, a powerful ensemble learning technique. Gradient boosting can be used for both regression and classification tasks. MLBG offers an easy-to-use API for training gradient boosting models in Ruby.
Installation
You can install MLBG using RubyGems:
ruby gem install mlbg
Example Usage
ruby require 'mlbg' # Create a gradient boosting classifier clf = MLBG::GradientBoostingClassifier.new clf.fit(X, y) # Make predictions predictions = clf.predict([[5, 6], [6, 7]]) puts "Predictions: #{predictions}"
MLBG is a valuable Gem for those looking to harness the power of gradient boosting in their machine learning projects.
10. Mlperf
Machine Learning Benchmarking
Mlperf is a Ruby Gem designed for benchmarking machine learning models. It allows you to evaluate the performance of your machine learning algorithms and compare them with industry-standard benchmarks. This Gem is essential for ensuring that your models meet the required quality and efficiency standards.
Installation
You can install Mlperf using RubyGems:
ruby gem install mlperf
Example Usage
ruby require 'mlperf' # Create a benchmark for your machine learning model benchmark = Mlperf::Benchmark.new # Evaluate the model's performance accuracy = benchmark.evaluate(model, test_data) puts "Model Accuracy: #{accuracy}"
Mlperf simplifies the process of benchmarking your machine learning models, helping you ensure they meet the highest standards of quality and efficiency.
Conclusion
Ruby might not be the first language that comes to mind when you think about machine learning, but these 10 Ruby Gems prove that it has a lot to offer in this field. Whether you’re handling data, implementing custom algorithms, or building predictive models, these Gems provide the tools you need to excel in machine learning with Ruby. So, go ahead and explore the world of machine learning in Ruby, and see how these Gems can empower your projects. Happy coding!
Table of Contents
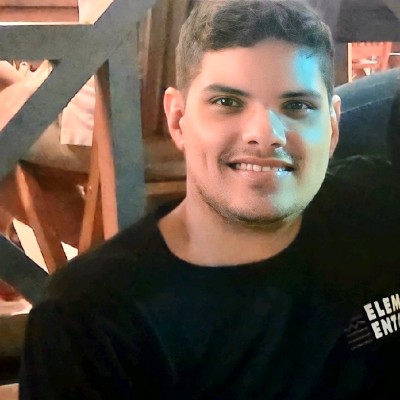
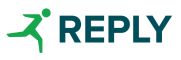