10 Ruby Gems for Real-Time Communication
Real-time communication is a fundamental aspect of modern web applications. Whether you’re building a chat application, a live-streaming platform, or a collaborative workspace, enabling real-time interactions is crucial. Fortunately, the Ruby community offers a wide range of powerful gems that make implementing real-time features a breeze.
Table of Contents
In this blog post, we’ll explore 10 essential Ruby gems for real-time communication. From WebSocket libraries to chat engines, these gems will help you add interactivity and responsiveness to your Ruby on Rails or Sinatra applications. Let’s dive in!
1. Action Cable
Action Cable is a part of the Ruby on Rails framework, making it an excellent choice for developers building Rails applications. It seamlessly integrates WebSocket support into your application, allowing you to create real-time features like chat applications, notifications, and more.
Installation:
ruby # Add to your Gemfile gem 'actioncable' # Install bundle install
Action Cable provides a high-level API for managing WebSocket connections and broadcasting messages to connected clients. It’s a robust solution for building real-time applications within the Rails ecosystem.
Usage Example:
ruby # app/channels/chat_channel.rb class ChatChannel < ApplicationCable::Channel def subscribed stream_from "chat_#{params[:room_id]}" end def receive(data) # Handle incoming messages ActionCable.server.broadcast("chat_#{params[:room_id]}", message: data['message']) end end
2. Faye
Faye is a simple and efficient WebSocket server for Ruby. It’s not tied to any specific framework, making it a versatile choice for adding real-time capabilities to both Rails and non-Rails applications.
Installation:
ruby # Add to your Gemfile gem 'faye' # Install bundle install
Faye provides a minimalistic API for creating WebSocket servers and clients. It’s lightweight and easy to set up, making it a great choice for small to medium-sized projects.
Usage Example:
ruby # Create a Faye server require 'faye' faye_server = Faye::RackAdapter.new( mount: '/faye', timeout: 25 ) # Mount the server in your app run faye_server
3. Pusher
Pusher is a hosted WebSocket service that simplifies real-time communication. It provides a Ruby gem that makes it easy to integrate real-time features into your application, such as live notifications and chat.
Installation:
ruby # Add to your Gemfile gem 'pusher' # Install bundle install
Pusher handles the WebSocket infrastructure, allowing you to focus on building the interactive features of your application. It offers an easy-to-use API for broadcasting messages and handling client connections.
Usage Example:
ruby require 'pusher' pusher = Pusher::Client.new( app_id: 'your_app_id', key: 'your_api_key', secret: 'your_api_secret', cluster: 'your_cluster', encrypted: true ) # Trigger an event pusher.trigger('my-channel', 'my-event', { message: 'Hello, World!' })
4. AnyCable
AnyCable is a WebSocket server that works seamlessly with Ruby on Rails. It’s designed to replace Action Cable’s built-in WebSocket server with a more efficient solution, allowing you to handle a higher number of connections.
Installation:
ruby # Add to your Gemfile gem 'anycable-rails' # Install bundle install
AnyCable is compatible with Action Cable, meaning you can use it as a drop-in replacement without changing your existing WebSocket code. It’s an excellent choice for applications that require high concurrency and low latency.
Usage Example:
ruby # config/cable.yml production: adapter: any_cable url: redis://localhost:6379/1
5. CableReady
CableReady is a gem that works hand in hand with Action Cable to provide a set of tools for updating the client’s UI in real-time. It simplifies the process of pushing updates from the server to the client, making your real-time features more interactive and engaging.
Installation:
ruby # Add to your Gemfile gem 'cable_ready' # Install bundle install
CableReady provides a simple and expressive API for performing operations on the client’s DOM, such as adding elements, removing elements, or modifying attributes. It’s especially useful for building interactive interfaces that respond to real-time data changes.
Usage Example:
ruby # app/channels/comments_channel.rb class CommentsChannel < ApplicationCable::Channel def subscribed stream_from "comments_#{params[:post_id]}" end def create_comment(comment) # Save the comment comment.save # Send a CableReady operation to update the client's UI cable_ready["comments_#{comment.post_id}"].insert_adjacent_html( selector: "#comments", position: "beforeend", html: render(comment) ) cable_ready.broadcast end end
6. WebSockets-Rails
WebSockets-Rails is a gem that adds WebSocket support to your Rails application. It provides a WebSocket server that integrates seamlessly with your existing Rails infrastructure.
Installation:
ruby # Add to your Gemfile gem 'websocket-rails' # Install bundle install
WebSockets-Rails allows you to define WebSocket controllers and events, making it easy to handle WebSocket connections and events in a structured manner.
Usage Example:
ruby # app/channels/chat_channel.rb class ChatChannel < WebsocketRails::BaseController def initialize_session # Perform initialization here end def client_connected # Handle new WebSocket connections end def new_message # Handle incoming messages end end
7. Slack-Ruby-Bot
Slack-Ruby-Bot is a gem for building Slack bots in Ruby. While it’s not a WebSocket library per se, it enables real-time interactions within the Slack ecosystem. You can use it to create custom Slack bots that respond to messages, automate tasks, and provide real-time information.
Installation:
ruby # Add to your Gemfile gem 'slack-ruby-bot' # Install bundle install
Slack-Ruby-Bot provides a straightforward API for creating Slack bots and defining their behavior. It’s a fun and practical way to incorporate real-time communication into your Slack workspace.
Usage Example:
ruby require 'slack-ruby-bot' class MyBot < SlackRubyBot::Bot command 'hello' do |client, data, match| client.say(text: "Hello, #{data.user}!", channel: data.channel) end end MyBot.run
8. Sync
Sync is a gem for building real-time collaborative applications like Google Docs. It provides a set of tools and a server for handling real-time data synchronization among clients.
Installation:
ruby # Add to your Gemfile gem 'sync' # Install bundle install
Sync allows you to create collaborative features in your application, such as real-time document editing and collaborative drawing tools. It’s an excellent choice for projects that require seamless real-time synchronization of data.
Usage Example:
ruby # Set up the Sync server Sync.setup do |config| config.secret_key = 'your_secret_key' end # Define a document class Document include Sync::Model sync_attrs :title, :content end
9. Sneakers
Sneakers is a background processing framework for Ruby that supports real-time task processing. While it’s not a traditional real-time communication gem, it can be used to process real-time tasks efficiently.
Installation:
ruby # Add to your Gemfile gem 'sneakers' # Install bundle install
Sneakers is particularly useful for handling time-consuming tasks in the background, allowing your application to remain responsive in real-time. It’s a great choice for offloading tasks that could otherwise impact the user experience.
Usage Example:
ruby require 'sneakers' class MyWorker include Sneakers::Worker from_queue 'my_queue' def work(msg) # Process the task in the background end end MyWorker.run
10. Chatwoot
Chatwoot is an open-source customer support and messaging platform that provides a Ruby gem for integration. While it’s not a standalone real-time gem, it allows you to add real-time chat and messaging features to your application.
Installation:
ruby # Add to your Gemfile gem 'chatwoot' # Install bundle install
Chatwoot offers an extensive API that enables you to create custom chat interfaces and integrate them seamlessly into your application. It’s a powerful tool for enhancing customer support and real-time communication.
Usage Example:
ruby # Initialize Chatwoot client client = Chatwoot::Client.new(token: 'your_api_token') # Create a new conversation conversation = client.conversations.create( inbox_id: 'inbox_id', contact_id: 'contact_id' ) # Send a message message = conversation.messages.create(content: 'Hello, how can I help you?') # Receive and process incoming messages in real-time client.messages.on_message do |message| # Handle incoming messages end
Conclusion
Real-time communication is a game-changer for modern web applications, and Ruby offers a rich ecosystem of gems to help you implement it seamlessly. Whether you’re building a chat application, a collaborative tool, or any other real-time feature, these Ruby gems will empower you to create interactive and engaging user experiences. Choose the one that best fits your project’s requirements, and start building real-time magic today!
Table of Contents
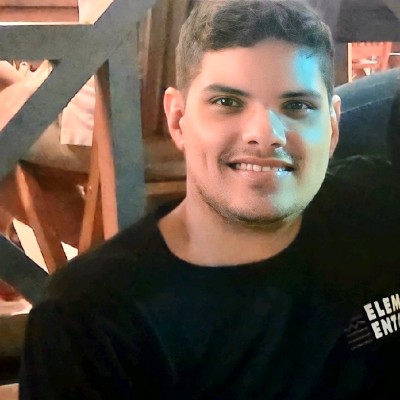
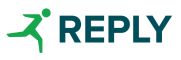