10 Ruby Gems for Testing and Debugging Your Application
Testing and debugging are crucial aspects of software development, ensuring that your application functions as intended and remains bug-free. Ruby, being a popular programming language, offers a vast ecosystem of gems that can simplify and streamline your testing and debugging process. In this blog post, we will explore 10 essential Ruby gems that can boost your productivity and help you deliver high-quality software. From unit testing frameworks to debugging tools, these gems cover a wide range of functionalities that every Ruby developer should be aware of.
1. RSpec
RSpec is a widely used and highly powerful testing framework for Ruby. It provides a domain-specific language (DSL) for expressive and readable specifications. With RSpec, you can write clear and concise tests that describe the expected behavior of your application. Here’s an example of an RSpec test:
ruby describe Calculator do it "adds two numbers correctly" do calculator = Calculator.new result = calculator.add(2, 3) expect(result).to eq(5) end end
2. Capybara
Capybara is an integration testing tool that simulates user interaction with your application. It allows you to write high-level acceptance tests that interact with your application’s UI. Capybara supports multiple drivers, including Selenium and headless browsers, giving you flexibility in choosing the environment for your tests. Here’s an example of a Capybara test:
ruby feature "User registration" do scenario "User signs up successfully" do visit "/signup" fill_in "Name", with: "John Doe" fill_in "Email", with: "john.doe@example.com" click_button "Sign Up" expect(page).to have_content "Welcome, John Doe!" end end
3. Pry
Pry is a feature-rich alternative to the standard IRB (Interactive Ruby) console. It enhances the debugging experience by providing additional features such as syntax highlighting, tab completion, and powerful introspection capabilities. Pry allows you to pause the execution of your code and interactively explore the current state of your application. Here’s an example of using Pry for debugging:
ruby require 'pry' def calculate_sum(a, b) binding.pry sum = a + b puts "The sum is #{sum}" end calculate_sum(2, 3)
4. FactoryBot
FactoryBot, formerly known as FactoryGirl, is a fixture replacement library that allows you to define and create test data with ease. It provides a flexible and concise syntax for defining factories, making it simple to create objects with realistic data for your tests. Here’s an example of a FactoryBot factory:
ruby FactoryBot.define do factory :user do name { Faker::Name.name } email { Faker::Internet.email } password { 'secret' } end end
5. Shoulda Matchers
Shoulda Matchers is a collection of matchers for RSpec that provides a convenient way to write expressive and readable test assertions. It simplifies common testing scenarios, such as validating associations, presence of attributes, and more. Here’s an example of using Shoulda Matchers:
ruby describe User do it { should validate_presence_of(:name) } it { should have_many(:articles) } it { should belong_to(:team).optional } end
6. SimpleCov
Code coverage is an essential metric in testing. SimpleCov is a code coverage analysis tool that helps you identify areas of your code that are not adequately covered by tests. It generates HTML reports that show which lines of code were executed during your test suite. Here’s an example of how to integrate SimpleCov into your test suite:
ruby require 'simplecov' SimpleCov.start
7. VCR
VCR is a library that records HTTP interactions and replays them during tests. It allows you to stub external HTTP requests, reducing the dependencies on external services and speeding up your test suite. VCR also provides a flexible API for customizing and managing your recorded interactions. Here’s an example of using VCR:
ruby VCR.configure do |config| config.cassette_library_dir = 'spec/fixtures/vcr_cassettes' config.hook_into :webmock end describe MyService do it "fetches data from an API" do VCR.use_cassette('my_service_api') do # Test code that interacts with the API end end end
8. RuboCop
RuboCop is a popular static code analyzer and formatter for Ruby. It enforces a set of rules and guidelines to ensure consistent code style and best practices. By integrating RuboCop into your development workflow, you can catch potential issues and improve the overall quality of your codebase. Here’s an example of running RuboCop on your project:
shell rubocop
9. Bullet
Bullet is a gem that helps you identify and optimize N+1 query issues in your ActiveRecord-based applications. It provides notifications and suggestions for reducing database queries and improving performance. Bullet can be integrated into your test suite to catch N+1 query problems early on. Here’s an example of configuring Bullet:
ruby if Rails.env.development? Bullet.enable = true Bullet.bullet_logger = true Bullet.raise = true end
10. Guard
Guard is a command-line tool that watches files for changes and triggers tasks automatically. It is commonly used in combination with testing frameworks to run tests whenever a relevant file is modified. With Guard, you can eliminate the need for manual test execution and ensure that your tests are always up to date. Here’s an example of configuring Guard for RSpec:
shell guard init rspec
Conclusion
Testing and debugging are essential for building robust and reliable software. By leveraging the power of these 10 Ruby gems, you can enhance your testing and debugging workflow, save time, and improve the quality of your code. Whether you are writing unit tests, integration tests, or debugging your application, these gems provide the necessary tools to make your development process more efficient and effective. So go ahead, explore these gems, and take your Ruby development to the next level!
Remember, the gems mentioned in this blog post are just the tip of the iceberg. Ruby’s vast ecosystem offers many more gems for various testing and debugging scenarios. Stay curious, keep exploring, and leverage the power of Ruby gems to build exceptional software. Happy coding!
Table of Contents
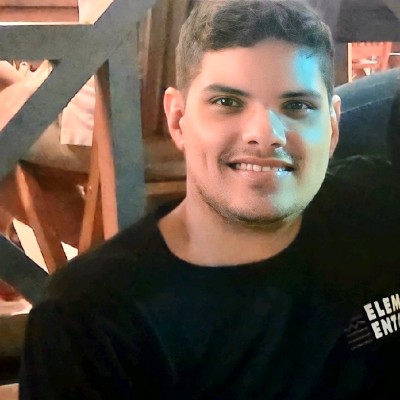
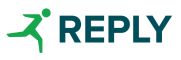