How to Use Ruby Functions for GraphQL API Development
GraphQL has gained immense popularity in recent years for its flexibility and efficiency in building APIs. If you’re a Ruby developer, you’ll be pleased to know that Ruby provides powerful tools for creating GraphQL APIs. In this blog, we’ll explore how to use Ruby functions effectively to develop a GraphQL API. We’ll cover everything from setting up a basic GraphQL server to more advanced concepts and best practices.
Table of Contents
1. Prerequisites
Before we dive into GraphQL API development with Ruby, make sure you have the following prerequisites:
- Basic knowledge of Ruby programming language.
- Familiarity with GraphQL concepts such as schemas, types, and resolvers.
If you’re new to GraphQL, it’s recommended to check out the official GraphQL documentation to get a better understanding of the fundamental concepts.
2. Setting up the Environment
To begin building our GraphQL API with Ruby, we need to set up our development environment. First, ensure you have Ruby installed on your system. You can check this by running the following command in your terminal:
ruby ruby -v
If you don’t have Ruby installed, visit the official Ruby website and follow the installation instructions.
Next, we’ll need to create a new Ruby project directory. Navigate to your preferred directory and create a new folder for your project:
bash mkdir my-graphql-api cd my-graphql-api
Inside this directory, we’ll set up a new Ruby project and install the required dependencies. We’ll use Bundler to manage our gems (Ruby libraries). If you don’t have Bundler installed, run:
bash gem install bundler
Now, create a new Gemfile using the following command:
bash bundle init
Open the Gemfile using a text editor and add the required gems for our GraphQL API:
ruby # Gemfile source 'https://rubygems.org' gem 'graphql' gem 'graphql-ruby' gem 'sinatra' gem 'sinatra-contrib'
Save the file and run the following command to install the gems:
bash bundle install
With our environment set up, we can now proceed to build our GraphQL API.
3. Creating the Schema
The first step in building a GraphQL API is defining the schema. The schema describes the types and their relationships in the API. Let’s create a basic schema for a simple blogging platform.
Inside your project directory, create a new file named schema.rb:
ruby # schema.rb require 'graphql' PostType = GraphQL::ObjectType.define do name 'Post' description 'A blog post' field :id, !types.ID field :title, !types.String field :body, !types.String end QueryType = GraphQL::ObjectType.define do name 'Query' description 'The root query type' field :post do type PostType argument :id, !types.ID resolve ->(obj, args, ctx) { Post.find(args[:id]) } end end Schema = GraphQL::Schema.define do query QueryType end
In the code above, we define a PostType, which represents a blog post, and a QueryType with a single field post, which allows querying a specific post by its ID.
4. Setting up the Server
With our schema defined, it’s time to set up the server to handle GraphQL queries. We’ll use Sinatra, a lightweight Ruby web framework, for this purpose.
Create a new file named app.rb in your project directory:
ruby # app.rb require 'sinatra' require 'sinatra/contrib' class MyGraphQLApp < Sinatra::Base post '/graphql' do result = Schema.execute( params[:query], variables: params[:variables] ) json result end end
The code above sets up a simple Sinatra app with a single POST endpoint at /graphql. When a query is received, it passes it to our schema for execution and returns the result as JSON.
5. Running the Server
To start the server, add the following line at the end of the app.rb file:
ruby MyGraphQLApp.run!
Now, run the following command to start the server:
bash ruby app.rb
The GraphQL server will be up and running at http://localhost:4567/graphql. You can test it using tools like GraphiQL or Insomnia by sending POST requests to http://localhost:4567/graphql with your GraphQL queries.
6. Creating Resolvers
Resolvers are functions that resolve the data requested by a query. In our example schema, we need to define resolvers for the post field. Resolvers take arguments (query variables) and fetch data accordingly. Let’s add some resolvers to fetch data for our blog posts.
Add the following code at the end of schema.rb:
ruby # schema.rb (continued) class Post attr_accessor :id, :title, :body def initialize(id, title, body) @id = id @title = title @body = body end # Simulated data for blog posts POSTS = [ Post.new(1, 'Hello, World!', 'This is my first blog post.'), Post.new(2, 'Ruby Functions for GraphQL', 'Learn how to use Ruby functions for GraphQL API development.'), ] def self.find(id) POSTS.find { |post| post.id == id.to_i } end end
In this code, we define a simple Post class with attributes id, title, and body. We also create a static array POSTS that holds two sample blog posts. The find method allows us to find a post by its ID.
Now, update the QueryType in schema.rb to use the Post class and fetch data using the find method:
ruby # schema.rb (continued) QueryType = GraphQL::ObjectType.define do name 'Query' description 'The root query type' field :post do type PostType argument :id, !types.ID resolve ->(obj, args, ctx) { Post.find(args[:id]) } end end
7. Mutations
In GraphQL, mutations are used to modify data on the server. Mutations are similar to queries but are executed with POST requests instead of GET. Let’s add a mutation to create a new blog post.
Update the schema.rb file to include the MutationType:
ruby # schema.rb (continued) MutationType = GraphQL::ObjectType.define do name 'Mutation' description 'The root mutation type' field :createPost do type PostType argument :title, !types.String argument :body, !types.String resolve ->(obj, args, ctx) do new_post = Post.new(POSTS.length + 1, args[:title], args[:body]) POSTS.push(new_post) new_post end end end
In this code, we define a MutationType with a single field createPost. The createPost mutation takes two arguments, title and body, both of type String. The resolve block creates a new Post object with the provided title and body, assigns it a new ID, and adds it to the POSTS array.
Update the Schema to include the MutationType:
ruby # schema.rb (continued) Schema = GraphQL::Schema.define do query QueryType mutation MutationType end
With these changes, our GraphQL API now supports creating new blog posts using the createPost mutation.
Conclusion
Congratulations! You’ve learned how to use Ruby functions effectively for GraphQL API development. We covered setting up the development environment, defining the schema, setting up a server with Sinatra, creating resolvers, and implementing mutations. This is just the beginning; GraphQL offers a vast array of features and possibilities for building robust APIs.
Keep experimenting with GraphQL and Ruby, and you’ll find yourself developing powerful APIs that serve your application’s needs efficiently. Happy coding!
Table of Contents
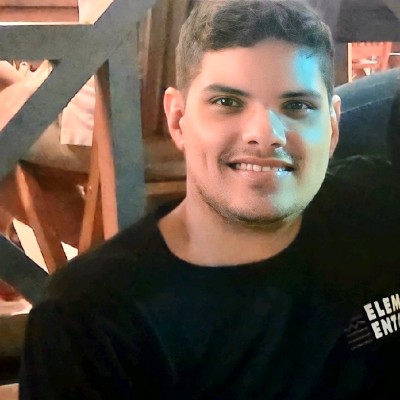
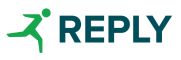