How to handle background jobs in Rails?
Handling background jobs is crucial in many Rails applications to ensure that time-consuming tasks don’t block the main application flow or slow down user experiences. Background jobs allow these tasks to run asynchronously, in the “background,” without interrupting the normal processing of web requests. Here’s a primer on managing background jobs in Rails:
- Why Background Jobs?: Imagine scenarios where an app needs to send a bulk email, process large files, or call external APIs. Running these operations synchronously could lead to long wait times for users. Background jobs offload these tasks, ensuring the main application remains snappy.
- Built-in Solution – Active Job: Rails introduced Active Job as a unified framework for declaring background jobs. It provides a common interface, allowing developers to switch between different background processors like Sidekiq, Resque, or Delayed Job. With Active Job, you define jobs that are enqueued and then processed by the chosen backend.
To create a new job:
```ruby rails generate job MyBackgroundJob ```
This will generate a file in `app/jobs`. Within this file, you’d implement the `perform` method with the logic of the task.
- External Libraries: While Active Job is versatile, sometimes specific requirements might lead developers to choose specific libraries:
– Sidekiq: A popular choice, Sidekiq uses threads, making it efficient for IO-bound tasks. It relies on Redis for storing jobs.
– Resque: Based on Redis, it’s a robust solution that can be easily scaled with multiple workers.
– Delayed Job: It stores jobs in the application’s database. While it doesn’t need an additional system like Redis, it might be slower for very large queues.
- Monitoring: Tools like Sidekiq come with web interfaces to monitor job status, retries, and failures. It’s important to keep an eye on this, especially in production, to ensure jobs run smoothly.
- Error Handling & Retries: Background jobs can fail for various reasons. Many libraries offer mechanisms to handle failures, like retries with backoff. Ensuring robust error handling is essential to make the most of background processing.
Background jobs in Rails allow developers to offload lengthy operations, ensuring user-facing components remain responsive. Through a combination of built-in tools like Active Job and third-party libraries, Rails offers a comprehensive ecosystem to handle asynchronous processing efficiently.
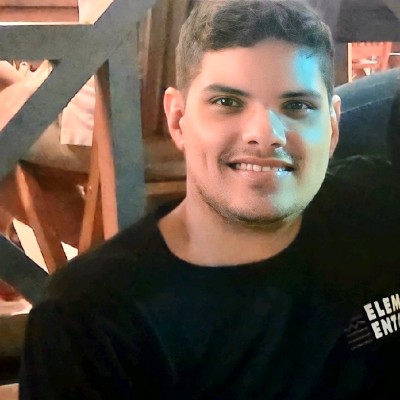
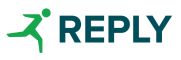