Ruby on Rails Q & A
How to handle emails in Rails?
Handling emails in Rails is facilitated by the framework’s built-in library called Action Mailer. Action Mailer allows you to send emails using mailer classes and views, making it seamlessly integrated with the rest of your Rails application. Here’s a brief overview of how to set up and use Action Mailer:
- Setup: First, you need to configure the email settings. In the `config/environments` folder, you’ll find configurations for development, test, and production. You can specify email delivery methods, SMTP settings, and other necessary details. For example:
```ruby config.action_mailer.delivery_method = :smtp config.action_mailer.smtp_settings = { address: 'smtp.example.com', port: 587, user_name: 'user@example.com', password: 'password', authentication: 'plain', enable_starttls_auto: true } ```
- Mailer Class: Generate a new mailer using the Rails generator, like `rails generate mailer UserMailer`. This will create a `UserMailer` class in `app/mailers/` where you define methods for different types of emails.
- Mailer Views: For each mailer method, you can create a corresponding view (HTML or plain text) under `app/views/user_mailer/` that dictates the email’s content. The beauty is you can use Rails templating just like for any other view.
- Sending Emails: Once your mailer class and views are set up, sending an email is as simple as calling a method. For example:
```ruby UserMailer.welcome_email(@user).deliver_now ```
- Testing: Rails offers ways to test your emails to ensure they are being sent and received as expected. In your test environment, emails are not actually sent but added to an `ActionMailer::Base.deliveries` array.
- Background Jobs: For scalability, especially with a large volume of emails, it’s a good practice to process email delivery in the background using tools like Sidekiq or DelayedJob.
Action Mailer in Rails provides a comprehensive, yet intuitive way to handle emails, making it easy to send, receive, and even test them. Integration with the broader Rails ecosystem means you can leverage all your existing tools and knowledge when working with emails.
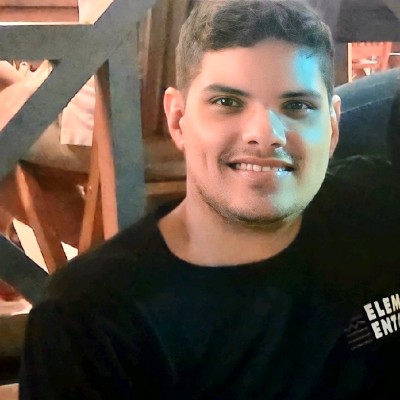
Previously at
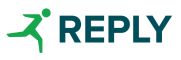
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.