Ruby on Rails Q & A
How to handle exceptions in Rails?
Handling exceptions appropriately in Rails is essential for providing a stable and user-friendly application experience. Here’s how to manage exceptions effectively in Rails:
- Exception Handling in Controllers: In Rails controllers, you can use the `rescue_from` method to catch specific exceptions and handle them. For instance:
```ruby class ApplicationController < ActionController::Base rescue_from ActiveRecord::RecordNotFound, with: :record_not_found private def record_not_found render plain: "404 Not Found", status: 404 end end ```
The above code captures any instance of the `ActiveRecord::RecordNotFound` exception and responds with a 404 status.
- Use the `begin-rescue-end` Block: In Ruby, you can wrap potentially error-prone code in a `begin-rescue-end` block:
```ruby begin # code that might raise an exception rescue => exception # handle the exception end ```
- Custom Exceptions: For application-specific errors, you can define custom exceptions. This allows for clearer, more granular error handling. Create custom exception classes in the `lib` directory or within your app’s model/service.
- Centralized Exception Management: Tools like `exception_notification` or `sentry-raven` gems allow centralized exception tracking. They can notify developers via email, Slack, or other platforms when an exception occurs.
- Public Error Pages: Rails provides default error pages for responses like 404 and 500. However, you can customize these pages by creating your own in the `public` directory.
- Logging: Always log exceptions to help with debugging. Rails automatically logs exceptions in the `log` directory, but you can also add custom logging messages using the Rails logger (`Rails.logger.error`).
- Development vs. Production: Remember, Rails behaves differently in development and production environments. In development, Rails provides detailed exception messages for debugging. In production, it displays generic error messages to users. Ensure you test exception handling in both environments.
Effectively handling exceptions in Rails is a blend of utilizing built-in Rails methods, adopting Ruby’s exception mechanisms, and incorporating third-party tools for comprehensive exception management. Properly handled exceptions lead to a more robust application and a better user experience.
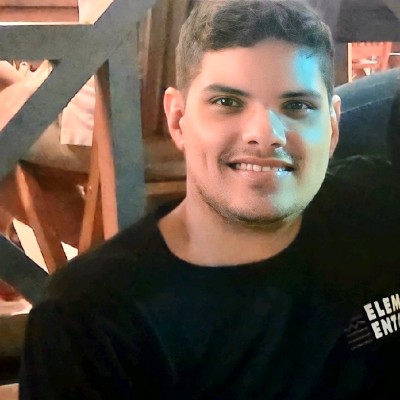
Previously at
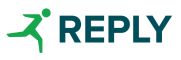
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.