How to handle file uploads in Rails?
Handling file uploads is a common task in web applications, and Rails provides several tools and libraries to manage this process seamlessly:
- CarrierWave: One of the most popular gems for handling file uploads in Rails. It’s not only efficient in storing files but also offers functionalities like image processing through integration with the MiniMagick gem.
– Install it by adding to your Gemfile:
```ruby gem 'carrierwave' ``` - Then run `bundle install`.
– Generate an uploader:
``` rails generate uploader Image ```
– Mount the uploader in the desired model, e.g., for a `Profile` model with an image:
```ruby mount_uploader :image, ImageUploader ```
- ActiveStorage: Introduced in Rails 5.2, ActiveStorage provides a built-in solution for attaching files to Active Record objects.
– To set up, run:
``` rails active_storage:install ```
– This will generate migration files, which you then apply using `rails db:migrate`.
– Attach files to a model by declaring the attachment reference, e.g., for an `Article` model with images:
```ruby has_many_attached :images ```
- Shrine: Another flexible and robust file attachment toolkit for Ruby applications. It offers a rich plugin system and allows handling of various file storage scenarios.
– To integrate, add to your Gemfile:
```ruby gem 'shrine' ```
- Cloud Storage: Often, in production, you’ll want to store files in cloud services like Amazon S3, Google Cloud Storage, or Azure Blob Storage. Both CarrierWave and ActiveStorage support integrations with these services, ensuring scalable and reliable file storage.
- Direct Uploads: Especially when dealing with large files, direct uploads to cloud services improve user experience by reducing the time a user spends waiting for a server response. Libraries like ActiveStorage come with built-in support for direct uploads.
- Security: Always ensure that file uploads are secure. Limit file types and sizes, use secure naming practices, and scan for malware. Also, never trust user-provided filenames – always generate a secure random name for stored files.
Rails offers a variety of options to handle file uploads, each catering to different needs. Whether you opt for a solution like CarrierWave or ActiveStorage, it’s crucial to maintain best practices concerning file validation, storage, and security. Integrating with reliable cloud storage services further ensures scalability and durability for your application’s uploaded files.
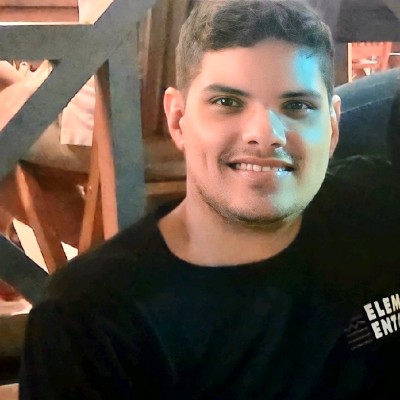
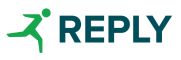