How to handle multiple databases in Rails?
Handling multiple databases in Rails has become increasingly streamlined, especially with the enhancements introduced in Rails 6. Here’s a succinct overview:
- Database Configuration:
Begin by defining your multiple databases in the `config/database.yml` file. Each database will have its configuration for different environments (development, test, production). Here’s a basic structure:
```yaml development: primary: database: primary_development adapter: sqlite3 secondary: database: secondary_development adapter: sqlite3 ```
- Model Configuration:
Once you have your databases set up in the `database.yml` file, you can specify which models should connect to which database. Use the `connects_to` method in your model:
```ruby class MyModel < ApplicationRecord connects_to database: { writing: :primary, reading: :secondary } end ```
- Migrations:
For migrations, you’ll need to specify which database you’re targeting. This is done using a directory structure in your `db/migrate` directory, with each database getting its folder. Running migrations will then look like:
``` rails db:migrate:up DATABASE=secondary ```
- Database Tasks:
Rails 6 introduced namespaced database tasks, allowing you to run tasks for a specific database. For instance, to create both databases, you’d use:
``` rails db:create:primary rails db:create:secondary ```
- Middleware Consideration:
If you’re using a request-based middleware (e.g., for switching databases based on the domain or subdomain), ensure that it is correctly set up to switch between your databases as needed.
- Database Connection Management:
Always be mindful of connection pool sizes and configurations. Managing multiple databases means more connections, and you’ll need to ensure that your app isn’t exhausting available connections, especially in multi-threaded environments.
While Rails has introduced tools and structures for handling multiple databases more straightforwardly, it requires careful planning and understanding of your application’s needs. Make sure to consider the trade-offs and complexities that come with introducing multiple databases to your app.
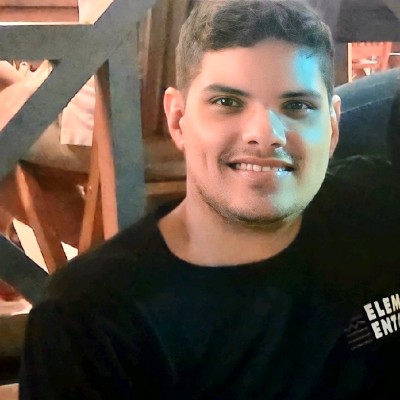
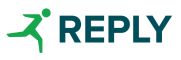