What are Rails helpers?
Rails helpers are specialized methods designed to assist views in rendering their content. Their primary purpose is to abstract away repetitive tasks, simplify views, and manage presentation logic, ensuring that views remain clean and focused on display matters. Here’s a deeper dive into Rails helpers:
- Nature and Purpose: Helpers are essentially Ruby methods, and they exist in the context of views. They handle tasks ranging from formatting dates to creating links, forms, or even custom logic that you don’t want to clutter your view templates with.
- Built-in Helpers: Rails comes equipped with a plethora of built-in helpers. Some common ones include:
– Form Helpers: Assist in creating forms, form fields, and other form elements. For example, `form_for`, `text_field`, and `submit_tag`.
– URL Helpers: Generate paths or URLs for your application. Examples include `link_to`, which creates a hyperlink, and named route helpers like `root_path` or `edit_user_path`.
– Asset Helpers: Help incorporate assets like images, JavaScripts, or stylesheets. Examples are `image_tag`, `javascript_include_tag`, and `stylesheet_link_tag`.
– Number and Date Helpers: Format numbers or dates in specific ways. For instance, `number_to_currency` or `time_ago_in_words`.
- Custom Helpers: While built-in helpers are extremely versatile, there will be times when you need custom logic specific to your application. Rails allows you to define your own helper methods. These typically reside in a module within the `app/helpers` directory. Once defined, they’re automatically available to all views.
For example, you might have:
```ruby module ApplicationHelper def highlight(text) content_tag(:strong, text) end end ```
In your views, you could then use:
```ruby <%= highlight('Important!') %> ```
This would render the text “Important!” wrapped in a `<strong>` tag.
- Keep It Clean: While helpers are powerful, it’s important not to overload them with too much logic. They should remain presentation-oriented. Business logic should be kept in models or services.
Rails helpers are a developer’s toolkit for managing view-centric tasks. They ensure that view templates remain legible and maintainable while offering a centralized way to handle presentation logic. They’re a testament to Rails’ philosophy of convention over configuration and its commitment to clean, organized code.
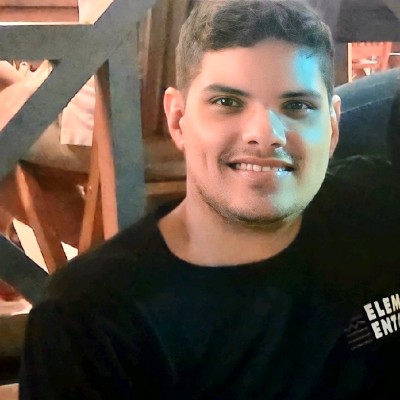
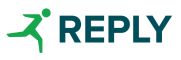