How to implement real-time features in Rails?
To implement real-time features in Rails, one popular and integrated approach is to use Action Cable. Action Cable seamlessly integrates WebSockets with the rest of a Rails application, allowing for real-time features such as chat rooms, notifications, and live updates.
- Setup:
Action Cable comes out-of-the-box with Rails. If it isn’t set up yet, ensure you have the necessary files and configurations by running `rails generate channel`. This will create the required directories and files.
- Channels:
The core of Action Cable is the concept of “channels”. A channel encapsulates a real-time feature you want to provide. For example, for a chat application, you might create a `ChatChannel`. Channels are similar to Rails controllers, but they operate synchronously.
- Frontend Integration:
On the frontend, you’ll integrate with Action Cable JavaScript to initiate the WebSocket connection. The `cable.js` file (found in the `javascripts` directory) initializes this. Here, you can subscribe to channels and define client-side behavior in response to real-time updates.
- Broadcasting:
Back on the server side, once some real-time event occurs (e.g., a new chat message), you “broadcast” a message to the channel. All subscribed clients will receive this broadcast and can act on it. For example:
```ruby ActionCable.server.broadcast 'chat_channel', message: 'Hello, World!' ```
- Deployment Considerations:
When deploying a Rails app with Action Cable, consider the requirements of WebSocket in terms of server configuration. Some platforms, like Heroku, require specific setup for WebSocket to work.
- Alternatives:
While Action Cable is integrated with Rails, there are other solutions like Pusher or AnyCable that might offer better scalability or specific features depending on your needs.
Action Cable provides an intuitive way to integrate real-time features in Rails, leveraging the WebSocket protocol. By creating channels and broadcasting updates, developers can create interactive, live-updating applications with ease.
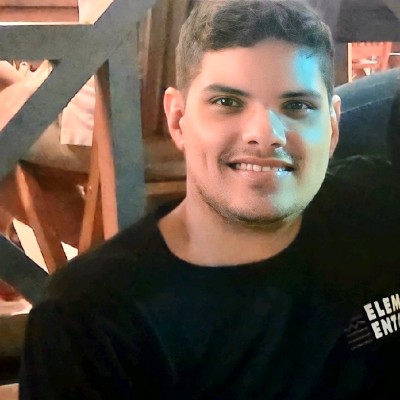
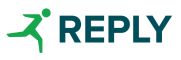