How to implement a search feature in Rails?
Implementing a search feature in a Rails application can be approached in a few ways, depending on the complexity and performance needs.
- Simple Queries using Active Record: For basic searches, you can use SQL-like queries with Active Record. For example, if you wanted to search for a user by name:
```ruby @users = User.where("name LIKE ?", "%#{params[:search_term]}%") ```
This method is straightforward but may become slow with large datasets.
- Using a Search Gem: There are several gems that provide robust searching and filtering capabilities:
– pg_search: If you’re using PostgreSQL, `pg_search` leverages its full-text search capabilities. It’s easy to set up and integrates smoothly with Rails.
– ransack: This gem provides a simple way to create search forms. It supports multiple search criteria and can also handle sorting.
- Advanced Search Systems: For larger applications where search performance and advanced features (like faceting or multilingual support) are needed, more powerful solutions like Elasticsearch or Solr can be used. The `searchkick` gem, for example, integrates Elasticsearch with Rails, providing a rich and fast search experience.
To integrate `searchkick`:
– Add the gem to your Gemfile.
– In your model:
– Reindex your model with `Product.reindex`.
– Now, you can perform a search with `Product.search(“search_term”)`.
```ruby class Product < ApplicationRecord searchkick end ```
Regardless of the approach, it’s essential to consider performance and the user experience. Ensure search results are returned quickly, and consider implementing features like auto-suggestions or “Did you mean?” corrections for improved usability.
Rails offers several avenues to implement search, from simple database queries to advanced search systems. Choose the one that best matches your application’s needs and scalability concerns.
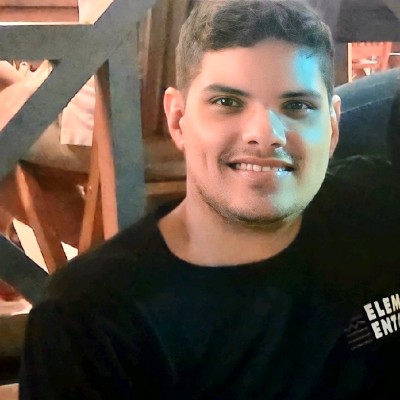
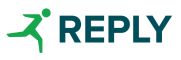