How to implement user authentication in Rails?
Implementing user authentication in Rails is a common requirement for many web applications, ensuring that users have the appropriate permissions to access specific resources. While Rails does not provide out-of-the-box user authentication, there are well-established gems that facilitate this task. Here’s a concise guide using the popular `Devise` gem:
- Installing Devise:
Begin by adding the Devise gem to your `Gemfile`:
```ruby gem 'devise' ```
After adding the gem, run `bundle install` to install it.
- Setting up Devise:
After installation, run the Devise generator:
```bash rails generate devise:install ```
This command provides setup instructions and creates an initializer in `config/initializers/devise.rb`.
- Generate User Model:
Devise can generate a user model for authentication. Execute:
```bash rails generate devise User ```
This will generate a `User` model with default fields such as email and password. It also provides a migration to create the `users` table in your database.
- Migrate Database:
To apply the changes to your database, run:
```bash rails db:migrate ```
- Routes, Views, and Controllers:
– Devise automatically updates your `routes.rb` file, adding routes for user authentication.
– By default, Devise uses its views, but you can customize them. To generate views that you can edit, run `rails generate devise:views`.
– While Devise handles most controller actions, you can override them for customized behavior.
- Using Authentication:
In your controllers, you can now use methods like `authenticate_user!` (ensures a user is logged in) or `current_user` (returns the logged-in user). For views, helpers like `user_signed_in?` check if a user is authenticated.
- Customizing:
Devise is highly customizable, allowing you to add fields to the User model, configure modules (like `:recoverable` for password recovery), or adjust the authentication flow.
Devise provides a robust solution for user authentication in Rails, encapsulating many best practices and security considerations. While the setup is streamlined, always familiarize yourself with the generated code and documentation, ensuring your authentication meets your application’s specific requirements.
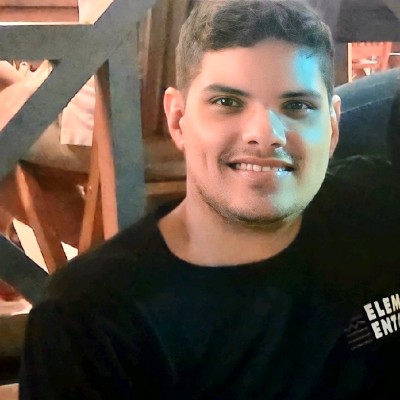
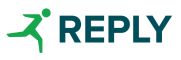