Ruby on Rails Q & A
How to integrate GraphQL in Rails?
GraphQL is a query language for APIs, and it provides a flexible and efficient way to fetch and update data. To integrate GraphQL into a Rails application, the “graphql” gem is typically used. This gem provides the essential tools to define, implement, and serve GraphQL queries.
- Setup: Begin by adding the `graphql` gem to your Gemfile and run `bundle install`:
```ruby gem 'graphql' ```
- Installation: After the gem is installed, run the installation generator:
```bash rails generate graphql:install ```
This command sets up the necessary directory structure, adds sample types, and creates a GraphQL controller to serve your queries.
- Defining Types: Inside the `app/graphql/types` directory, you can define various types. For example, if you have a `User` model, you might create a `user_type.rb` which defines what fields can be queried on a User.
- Creating Queries: In `app/graphql/types/query_type.rb`, you can define the available queries. For instance:
```ruby field :user, Types::UserType, null: false do argument :id, ID, required: true end def user(id:) User.find(id) end ```
- Execution: When a GraphQL query is sent to your server (typically via a POST request), it hits the `GraphqlController` that was generated earlier. This controller parses and executes the query against the types and resolvers you’ve defined.
- Development Tool: The installation also sets up a route to `graphiql-rails` (if the gem is included), which provides a development IDE for testing GraphQL queries.
- Performance and Security: As with any tool, be cautious about potential N+1 query problems. Tools like the `graphql-batch` gem can help with batch loading. Additionally, ensure proper authorization checks are in place before exposing sensitive data.
Integrating GraphQL with Rails allows you to create efficient, tailored APIs that fetch precisely the data they need, without over-fetching or under-fetching, providing an optimized client-server interaction experience.
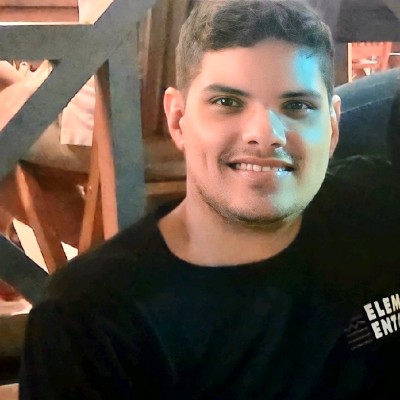
Previously at
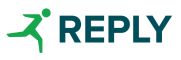
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.