Ruby on Rails Q & A
How to integrate third-party APIs in Rails?
Integrating third-party APIs in a Rails application is a common task, allowing your app to leverage external services for added functionality. Here’s a step-by-step approach:
- Research the API: Start by reading the API documentation thoroughly. Most reputable APIs will provide comprehensive documentation detailing endpoints, required parameters, authentication mechanisms, and response formats.
- Choose a HTTP Client: Rails does not come with an in-built HTTP client, so you’ll need to choose one. The `net/http` library is built into Ruby and can be used for this purpose, but many developers prefer gems like `httparty` or `faraday` due to their simplicity and additional features.
- Authentication: Most APIs require authentication. This can range from a simple API key to more complex OAuth procedures. Depending on the API’s requirements, you might embed the key in the header or send it as a parameter. Always ensure that sensitive keys are not hard-coded or exposed. Use Rails’ encrypted credentials for this purpose.
```ruby # Using HTTParty with an API key in the header response = HTTParty.get('https://api.example.com/data', headers: { "Authorization" => "Bearer #{Rails.application.credentials.api_key}" }) ```
- Making Requests: Construct your requests based on the API’s endpoints. Ensure you handle different HTTP methods (GET, POST, PUT, DELETE) as required by the API.
- Handle Responses: Once you receive a response, process the data as needed. This can involve parsing JSON, error handling, and storing relevant data in your database.
- Error Handling: It’s crucial to anticipate and handle errors gracefully. This can include rate limits exceeded, data not found, or internal server errors from the API. Utilize exception handling to manage these scenarios and provide useful feedback to users.
- Caching: To reduce the number of requests and enhance performance, consider caching frequent API responses using Rails’ caching mechanisms.
Integrating third-party APIs in Rails involves understanding the API, choosing an appropriate HTTP client, and ensuring robust error handling and response processing. Always follow best practices for security, especially when dealing with authentication and sensitive data.
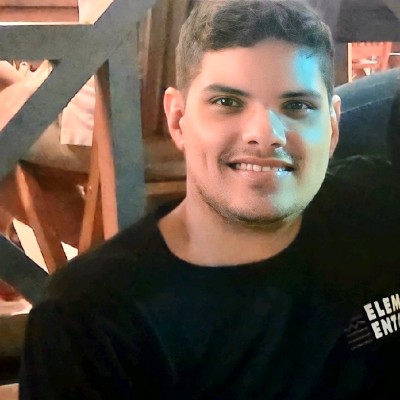
Previously at
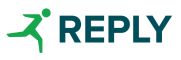
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.