How to use Redis in Rails?
Redis, a powerful in-memory key-value data store, is often utilized with Rails applications to enhance performance, particularly for caching, real-time analytics, and as a job queue system. Here’s a concise guide to integrating Redis with your Rails application:
- Installation & Setup:
– Begin by installing Redis on your machine. Instructions differ based on your OS, but many package managers like `brew` (for macOS) or `apt` (for Linux) offer easy installation commands.
– Ensure the Redis server is running with `redis-server`.
- Rails Integration:
– Add the `redis` gem to your Gemfile: `gem ‘redis’`, and run `bundle install`.
– This gem provides Ruby bindings to interact with the Redis server.
- Caching:
– Rails supports Redis as a cache store. To utilize Redis for caching, update your `config/environments/production.rb` (or the specific environment you want caching in) with:
```ruby config.cache_store = :redis_cache_store, { url: "redis://localhost:6379/0/cache" } ```
- Job Queues with Sidekiq:
– If you aim to use Redis as a backend for job processing, `Sidekiq` is a popular option.
– Add the Sidekiq gem: `gem ‘sidekiq’`.
– Configure Sidekiq to use Redis by creating an initializer (`config/initializers/sidekiq.rb`) with:
```ruby Sidekiq.configure_server do |config| config.redis = { url: 'redis://localhost:6379/0' } end Sidekiq.configure_client do |config| config.redis = { url: 'redis://localhost:6379/0' } end ```
– You can then set Sidekiq as the active job queue adapter in `config/application.rb`:
```ruby config.active_job.queue_adapter = :sidekiq ```
- Data Storage & Retrieval:
– To store and retrieve data, create a Redis instance: `redis = Redis.new(host: “localhost”, port: 6379, db: 0)`.
– Use commands like `redis.set(“key”, “value”)` for setting data and `redis.get(“key”)` for retrieval.
It’s crucial to note that, while Redis is fast and efficient, it’s an in-memory store. Ensure you have sufficient memory for your dataset and implement persistence settings if you don’t want data loss during restarts.
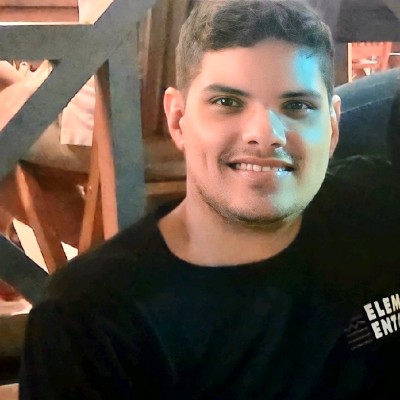
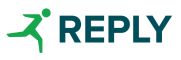