Ruby on Rails Tutorial: Understanding Internationalization and Localization
In today’s interconnected world, creating web applications that cater to a diverse global audience is essential for success. When developing a Ruby on Rails application, one crucial aspect to consider is internationalization (i18n) and localization (l10n). These concepts allow you to adapt your application to different languages, regions, and cultures, making it accessible and user-friendly for users worldwide.
Table of Contents
In this tutorial, we’ll dive into the concepts of internationalization and localization in Ruby on Rails. We’ll explore how to implement them step by step, and along the way, we’ll see code samples to understand the process better. So, let’s get started!
1. Understanding Internationalization (i18n)
1.1. What is Internationalization?
Internationalization, often abbreviated as i18n, is the process of designing and developing an application in a way that makes it easy to adapt to various languages and cultures. The primary goal of internationalization is to separate the content from the code, enabling the application to be used by speakers of different languages without altering the core functionality.
1.2. The Importance of Internationalization
Implementing internationalization is crucial for reaching a broader audience and expanding your user base. By providing content in different languages, you can engage users who prefer to interact with the application in their native tongue. This enhances user experience and fosters a sense of inclusivity.
Additionally, internationalization also benefits businesses by opening up opportunities in new markets. It allows you to tailor your application to specific regions, which can lead to increased customer satisfaction and higher conversion rates.
1.3. Rails and Internationalization
Ruby on Rails provides excellent support for internationalization out of the box. It offers a powerful set of tools and conventions to handle translations and localization efficiently.
2. Implementing Internationalization in Ruby on Rails
2.1. Setting Up the Application
Let’s start by creating a new Ruby on Rails application. If you haven’t installed Rails yet, you can do so by following the official installation guide (https://guides.rubyonrails.org/getting_started.html).
Once Rails is installed, open your terminal and run the following command:
bash rails new InternationalApp
This command will generate a new Rails application named “InternationalApp.” Now, navigate to the application directory:
bash cd InternationalApp
2.2. Configuring Internationalization
The first step to enable internationalization in our Rails application is to configure the default locale. Open the config/application.rb file and add the following line inside the Application class:
ruby config.i18n.default_locale = :en
In this example, we’ve set the default locale to English (:en). However, Rails supports many other locales out of the box, such as Spanish (:es), French (:fr), and German (:de). You can choose the default locale that best fits your application’s needs.
2.3. Creating Translation Files
Now that we’ve set up the default locale, let’s create translation files for different languages. Rails uses YAML (YAML Ain’t Markup Language) files to store translations. The YAML format is easy to read and write, making it ideal for handling translations.
In your Rails application’s root directory, navigate to the config/locales folder. Inside this folder, create a new file named en.yml for English translations:
yaml # config/locales/en.yml en: hello: "Hello, welcome to our application!" about_us: "About Us" contact_us: "Contact Us" # Add more translations as needed
Similarly, you can create translation files for other languages. For example, to add Spanish translations, create a file named es.yml:
yaml # config/locales/es.yml es: hello: "¡Hola, bienvenido a nuestra aplicación!" about_us: "Acerca de Nosotros" contact_us: "Contáctenos" # Add more translations as needed
2.4. Implementing Translations in Views
With the translation files in place, we can now use the translations in our views. Open the app/views/layouts/application.html.erb file and modify the navigation menu to include the translated links:
html <!-- app/views/layouts/application.html.erb --> <!DOCTYPE html> <html> <head> <title>InternationalApp</title> </head> <body> <nav> <ul> <li><%= link_to t('hello'), root_path %></li> <li><%= link_to t('about_us'), about_us_path %></li> <li><%= link_to t('contact_us'), contact_us_path %></li> </ul> </nav> <%= yield %> </body> </html>
In this example, we use the t method to look up translations from the appropriate YAML file based on the current locale. The t method takes a key (e.g., ‘hello’, ‘about_us’) and returns the corresponding translation.
2.5. Setting the Current Locale
By default, Rails determines the current locale based on the user’s browser settings. However, you may want to allow users to manually select their preferred language.
To achieve this, we can add a language switcher in our application. Create a new file named _language_switcher.html.erb inside the app/views/layouts folder:
html <!-- app/views/layouts/_language_switcher.html.erb --> <ul class="language-switcher"> <li><%= link_to "English", root_path(locale: :en) %></li> <li><%= link_to "Español", root_path(locale: :es) %></li> <!-- Add more language options as needed --> </ul>
Next, include the language switcher in the application layout:
html <!-- app/views/layouts/application.html.erb --> <!DOCTYPE html> <html> <head> <title>InternationalApp</title> </head> <body> <%= render 'layouts/language_switcher' %> <nav> <ul> <li><%= link_to t('hello'), root_path %></li> <li><%= link_to t('about_us'), about_us_path %></li> <li><%= link_to t('contact_us'), contact_us_path %></li> </ul> </nav> <%= yield %> </body> </html>
With this language switcher, users can now select their preferred language, and the application will change the locale accordingly.
2.6. Implementing Localization (l10n)
While internationalization focuses on making the application ready for translation, localization (l10n) is the process of providing translations for different languages and adapting the application to the specific conventions of each target locale. This includes formats for dates, times, currencies, and more.
2.7. Adding Locale-Specific Formats
To handle localization, Rails provides the config/locales folder, which we’ve used to store translation files. However, in addition to translations, you can also specify locale-specific formats in these files.
For example, if we want to format dates differently in English and Spanish locales, we can modify the translation files as follows:
yaml # config/locales/en.yml en: date: formats: default: "%m/%d/%Y" # config/locales/es.yml es: date: formats: default: "%d/%m/%Y"
In the example above, we’ve specified the date format for English (“%m/%d/%Y”) and Spanish (“%d/%m/%Y”) locales.
2.8. Localizing Views
Now that we have defined the locale-specific formats, we can use them in our views. Let’s modify the app/views/posts/show.html.erb file to display the post’s creation date using the localized format:
html <!-- app/views/posts/show.html.erb --> <h1><%= @post.title %></h1> <p><%= t('posted_on', date: l(@post.created_at)) %></p> <p><%= @post.content %></p>
In the example above, we’ve used the l method to format the created_at date using the locale-specific date format. The t method is used to translate the “posted_on” key and insert the formatted date in the translation.
2.9. Localizing Model Data
In addition to localizing views, you may also want to localize data in your models. Rails provides a convenient way to achieve this using the I18n.localize method.
For instance, suppose we have a Product model with a price attribute that we want to display in the appropriate currency format for each locale:
ruby # app/models/product.rb class Product < ApplicationRecord def formatted_price I18n.localize(price, format: :currency) end end
In this example, we’ve defined a formatted_price method that uses I18n.localize to format the price attribute as a currency. Rails will automatically pick the appropriate currency format based on the current locale.
2.10. Pluralization in Translations
When translating content, you may encounter scenarios where the text varies depending on the quantity. For example, in English, we say “1 book” but “2 books.” Pluralization rules vary between languages, so Rails provides a mechanism to handle this.
In your translation files, you can use the count key to handle pluralization. Let’s illustrate this with an example:
yaml # config/locales/en.yml en: book: one: "1 book" other: "%{count} books" # config/locales/es.yml es: book: one: "1 libro" other: "%{count} libros"
Now, in your views or models, you can use the t method with the count option to handle pluralization:
html <!-- app/views/books/index.html.erb --> <p><%= t('book', count: @books.count) %></p>
2.11. Date and Time Formats
In addition to handling pluralization, Rails also provides support for localizing date and time formats. You can specify different formats for dates, times, and datetimes in your translation files.
yaml # config/locales/en.yml en: date: formats: short: "%m/%d/%y" time: formats: short: "%m/%d/%y %H:%M" # config/locales/es.yml es: date: formats: short: "%d/%m/%y" time: formats: short: "%d/%m/%y %H:%M"
Now, when you display dates and times in your views using the l method, Rails will use the appropriate format based on the current locale.
2.12. Handling Missing Translations
When adding translations, it’s possible to miss a translation for a specific key or locale. To avoid showing raw keys in your application, Rails provides a fallback mechanism.
By default, Rails will fall back to the default_locale (set in config/application.rb) if a translation is missing for the current locale. If the default_locale translation is also missing, Rails will show the original key.
However, you can customize the fallback behavior by specifying a custom fallback chain in your application configuration. This allows you to define a sequence of locales to use as fallbacks when a translation is missing:
ruby # config/application.rb config.i18n.fallbacks = [:en, :es, :fr, :de]
In this example, if a translation is missing for the current locale, Rails will try to use the translation from English (:en), followed by Spanish (:es), French (:fr), and German (:de).
Conclusion
Congratulations! You’ve successfully learned how to implement internationalization and localization in Ruby on Rails. By following the steps and using the provided code samples, you can create web applications that cater to a global audience, making them accessible and user-friendly for users worldwide.
Remember that internationalization is not just about translating text; it’s also about adapting the application to different cultures, formats, and conventions. By embracing internationalization and localization, you’ll open up new opportunities, reach a broader audience, and improve user experience for users from different parts of the world.
Start implementing internationalization in your Rails projects today and make your applications global-ready! Happy coding!
Table of Contents
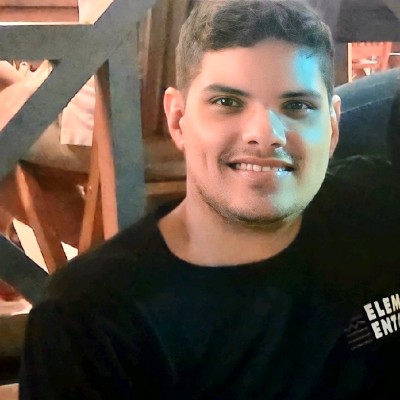
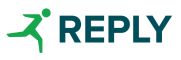