What are jobs in Rails and how to use them?
Jobs in Rails:
In the context of Ruby on Rails, “jobs” refer to units of work that can be executed asynchronously, outside the flow of the main application. This is particularly useful when handling operations that can be time-consuming, such as sending emails, processing files, or interacting with external APIs, where you wouldn’t want the user to wait for the task to complete.
Using Jobs in Rails:
- Active Job Framework: Rails provides the Active Job framework, which serves as a unified interface for creating background jobs. It allows you to write job classes and their execution logic. One of the advantages of Active Job is its flexibility in allowing you to switch between different background processing frameworks (queues) like Sidekiq, Resque, or Delayed Job.
- Creating a Job: You can generate a new job using Rails generators:
``` rails generate job MyJobName ```
This will create a file in the `app/jobs` directory where you can define the tasks to be performed.
- Writing a Job: Inside the generated job file, you’ll see a `perform` method. This is where you place the logic of the task to be executed:
```ruby def perform(*args) # Your job logic here end ```
- Enqueuing Jobs: Once the job is defined, you can enqueue it to be run immediately or at a specific future time:\
```ruby MyJobName.perform_later(arg1, arg2) # Enqueue the job to be performed as soon as the queueing system is free. MyJobName.set(wait: 5.minutes).perform_later(arg1, arg2) # Enqueue the job to be run in 5 minutes. ```
- Processing Frameworks: While Active Job provides the structure for creating jobs, you’ll need a backend like Sidekiq or Resque to process them. This involves additional setup, like managing Redis for storing job data.
- Monitoring and Errors: Many background processing tools come with dashboards for monitoring job status. They handle job retries and provide mechanisms to deal with failed jobs.
Jobs in Rails allow developers to perform lengthy or resource-intensive tasks in the background, ensuring the user isn’t left waiting. Utilizing the Active Job framework in combination with a processing backend helps in managing and executing these tasks efficiently.
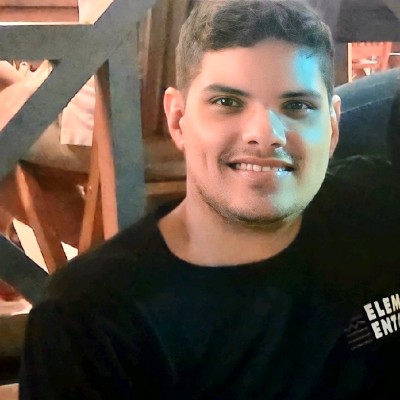
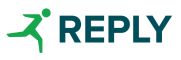