Ruby on Rails Q & A
How to manage database seeds in Rails?
Database seeding is the process of populating your database with initial data, which can be very helpful during the development phase or when setting up a new instance of an application. In Rails, the framework provides a built-in mechanism for this purpose through the `db/seeds.rb` file.
- Setting up Seeds: The `db/seeds.rb` file is where you write the code to seed your database. Here, you can use the standard ActiveRecord methods to create or find and update records. For instance:
```ruby User.create!(name: 'John Doe', email: 'john.doe@example.com') ```
- Running Seeds: Once you’ve defined your seeds in the `db/seeds.rb` file, you can run them with the following command:
``` rake db:seed ```
- Idempotency: It’s essential to ensure your seeding process is idempotent, meaning you can run it multiple times without causing duplicate entries or errors. To achieve this, you can use `find_or_create_by` or `first_or_create` methods:
```ruby User.find_or_create_by(email: 'john.doe@example.com') do |user| user.name = 'John Doe' end ```
- Seeding in Different Environments: Sometimes, you may want different seeds for different environments (development, test, production). While Rails does not provide a built-in way for this, you can use conditional statements in `db/seeds.rb` based on the current environment, like:
```ruby if Rails.env.development? # seeding data for development end ```
- External Libraries: If your seeding needs become more complex, there are gems like ‘Faker’ to generate fake data or ‘FactoryBot’ which can be combined with seeds for more structured and randomized data creation.
Managing database seeds in Rails is straightforward with the `db/seeds.rb` file. By efficiently leveraging this feature, developers can ensure a consistent initial state for their databases across different environments or development stages.
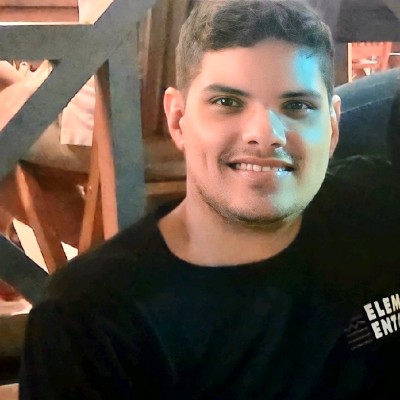
Previously at
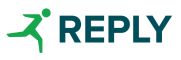
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.