Ruby on Rails Q & A
What is metaprogramming in Rails?
Metaprogramming is a powerful feature of Ruby that allows developers to write code that writes or manipulates other code. In simpler terms, it’s the art of creating code that operates on code, rather than on data. This can lead to more dynamic, flexible, and DRY (Don’t Repeat Yourself) code. Given Ruby’s object-oriented nature and its introspection capabilities, metaprogramming techniques can be applied elegantly.
- Dynamic Method Creation**: One common metaprogramming technique in Ruby is the dynamic creation of methods. Using `define_method`, you can programmatically create methods. For instance, if you have various attributes and you want to define a method for each attribute, instead of writing each method manually, you can generate them.
```ruby class Book attributes = [:title, :author, :isbn] attributes.each do |attribute| define_method(attribute) do puts "This is the book's #{attribute}" end end end ```
- `method_missing`: This is another popular metaprogramming technique in Ruby. Whenever you call a method that doesn’t exist on an object, Ruby invokes the `method_missing` method. You can override this method to catch those calls and handle them in custom ways.
- Introspection: Ruby provides various methods to inspect an object or class at runtime. Methods like `respond_to?`, `methods`, and `instance_variables` allow you to check the capabilities and states of objects, aiding in metaprogramming tasks.
- Evaluating Strings as Code with `eval`: The `eval` method lets you execute a string as Ruby code, which can be powerful but should be used with caution due to potential security risks.
- Class Macros: In Ruby, class-level methods can be used to define custom DSLs or other constructs, like the `attr_accessor`, `attr_reader`, and `attr_writer` macros that are used to create getter and setter methods.
Metaprogramming in Ruby offers ways to write more dynamic and concise code. While powerful, developers should approach it judiciously, as overuse or misuse can lead to code that’s hard to read, maintain, or can introduce security vulnerabilities.
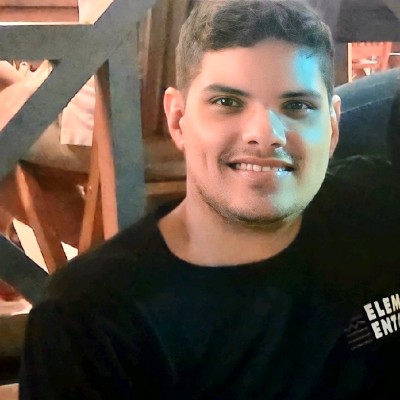
Previously at
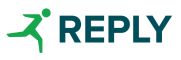
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.