What are Rails migrations and how do they work?
Rails migrations are a powerful feature of the framework that lets developers make changes to the database schema in a structured and version-controlled manner. Think of them as a set of instructions for evolving your database over time. They allow for adding, modifying, or removing tables, columns, and indexes, ensuring that the structure of your database remains consistent with the state of your application.
Here’s how they work:
- Creating Migrations: You can generate a new migration using Rails’ command-line tools. For example, if you want to create a new table called `users`, you might run:
```bash rails generate migration CreateUsers ```
This will generate a new file in the `db/migrate/` directory, which will have a name like `20231010123456_create_users.rb` (the numbers at the beginning represent a timestamp).
- Writing Migrations: Open the generated file. Inside, you’ll see a Ruby class with methods like `change`, `up`, or `down`. These methods define the actions to perform. For instance, to add a table:
```ruby def change create_table :users do |t| t.string :name t.string :email t.timestamps end end ```
- Running Migrations: Once the migration is defined, you apply it using:
```bash rails db:migrate ```
This command will run all pending migrations, applying the changes to the database. Rails keeps track of which migrations have been run, ensuring each is executed only once.
- Rolling Back Migrations: If you need to undo a migration, Rails provides the ability to rollback:
```bash rails db:rollback ```
This will undo the last migration applied. If you’ve made a series of changes and need to rollback multiple steps, you can specify how many with an additional argument, like `rails db:rollback STEP=3`.
Migrations in Rails provide a systematic, version-controlled approach to database schema changes. They ensure that developers, regardless of their environment, have a consistent and updated database schema, facilitating collaboration and reducing potential bugs related to database discrepancies.
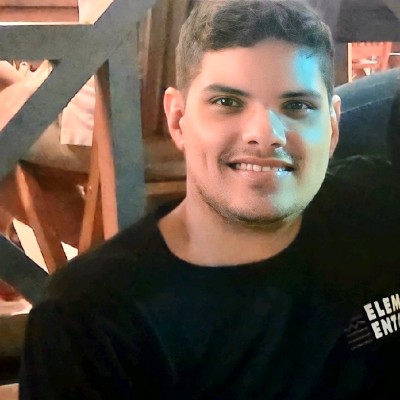
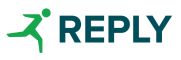