Ruby on Rails Tutorial: Understanding Model Associations and Joins
Ruby on Rails is a powerful web application framework known for its simplicity and convention-over-configuration philosophy. One of the key features that makes Rails a developer favorite is its robust support for database modeling through ActiveRecord. In this tutorial, we’ll explore the fundamental concepts of model associations and joins in Ruby on Rails, providing you with a solid foundation to build complex database relationships in your web applications.
Table of Contents
1. Setting up the Rails Environment
Before we dive into model associations and joins, make sure you have Ruby and Ruby on Rails installed on your system. You can check your Rails version by running:
ruby rails -v
If Rails is not installed, you can follow the official installation guide on the Ruby on Rails website. Once Rails is set up, you can create a new Rails application using the following command:
ruby rails new MyApp
Replace “MyApp” with your preferred application name. Navigate to your application’s directory:
ruby cd MyApp
Now that we have our Rails environment set up, let’s explore model associations.
2. Model Associations
Model associations in Rails allow you to define relationships between different models, making it easier to work with related data. There are three primary types of model associations:
2.1. One-to-One Associations
A one-to-one association represents a relationship where one record in a table is associated with one record in another table. Consider a simple example of a User model and a Profile model:
ruby # app/models/user.rb class User < ApplicationRecord has_one :profile end # app/models/profile.rb class Profile < ApplicationRecord belongs_to :user end
In this example, a user has one profile, and a profile belongs to a user. To set up a one-to-one association, we use the has_one and belongs_to methods. This association allows us to access a user’s profile or a profile’s user effortlessly.
2.2. One-to-Many Associations
A one-to-many association is used when one record in a table is associated with multiple records in another table. For instance, consider a User model and a Post model:
ruby # app/models/user.rb class User < ApplicationRecord has_many :posts end # app/models/post.rb class Post < ApplicationRecord belongs_to :user end
Here, a user can have multiple posts, but each post belongs to a single user. To establish a one-to-many association, we use the has_many method in one model and the belongs_to method in the other. This association simplifies tasks such as retrieving all posts by a specific user.
2.3. Many-to-Many Associations
A many-to-many association comes into play when multiple records in one table can be associated with multiple records in another table. Let’s consider a Tag model and a Post model:
ruby # app/models/tag.rb class Tag < ApplicationRecord has_and_belongs_to_many :posts end # app/models/post.rb class Post < ApplicationRecord has_and_belongs_to_many :tags end
In this scenario, a post can have multiple tags, and a tag can be associated with multiple posts. To create a many-to-many association, we use the has_and_belongs_to_many method in both models. This association enables us to easily retrieve posts associated with a specific tag or vice versa.
Now that you have a clear understanding of the three primary types of model associations, let’s move on to working with joins.
3. Working with Joins
Joins in Ruby on Rails allow you to fetch data from multiple tables simultaneously. Rails supports various types of joins, each serving a specific purpose. We’ll explore four common types of joins: inner joins, left joins, right joins, and outer joins.
3.1. Inner Joins
An inner join returns only the rows where there is a match in both tables being joined. Let’s say we want to retrieve all posts and their associated tags:
ruby # app/controllers/posts_controller.rb class PostsController < ApplicationController def index @posts = Post.joins(:tags) end end
In this example, we use the joins method to perform an inner join between the Post and Tag models. This retrieves only the posts that have associated tags.
3.2. Left Joins
A left join returns all rows from the left table and the matched rows from the right table. If there is no match, the result will contain NULL values for columns from the right table. To retrieve all users and their associated profiles (if they have one), we can use a left join:
ruby # app/controllers/users_controller.rb class UsersController < ApplicationController def index @users = User.left_joins(:profile) end end
By employing left_joins, we ensure that all users are included in the result, even if they don’t have a corresponding profile.
3.3. Right Joins
A right join is similar to a left join but returns all rows from the right table and the matched rows from the left table. To retrieve all tags and the posts associated with them (if any), we can use a right join:
ruby # app/controllers/tags_controller.rb class TagsController < ApplicationController def index @tags = Tag.right_joins(:posts) end end
This query ensures that all tags are included in the result, along with any associated posts.
3.4. Outer Joins
An outer join returns all rows when there is a match in one of the tables. To retrieve all users and their associated profiles (if they have one) or all profiles (if they exist without a user), we can use an outer join:
ruby # app/controllers/users_controller.rb class UsersController < ApplicationController def index @users = User.left_outer_joins(:profile) end end
By employing left_outer_joins, we get a result that includes all users and profiles, regardless of whether they have associated records.
Conclusion
In this tutorial, we’ve explored the essential concepts of model associations and joins in Ruby on Rails. These features empower you to build complex web applications that interact with your database efficiently.
By mastering one-to-one, one-to-many, and many-to-many associations, you can create rich relationships between your models, making it easier to retrieve and manipulate data. Additionally, understanding the different types of joins, such as inner joins, left joins, right joins, and outer joins, allows you to query your database with precision and flexibility.
As you continue your journey with Ruby on Rails, remember that model associations and joins are powerful tools in your development arsenal. By leveraging these features effectively, you’ll be able to create robust, data-driven web applications that meet the needs of your users and clients.
Happy coding!
Table of Contents
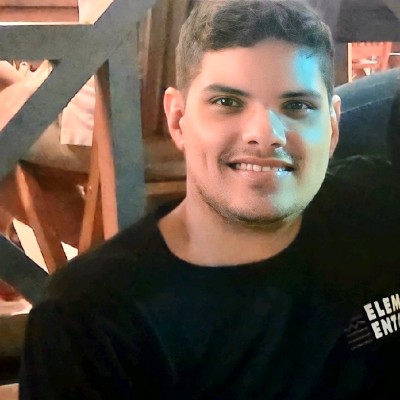
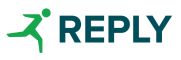