Ruby on Rails Tutorial: Understanding Model Inheritance and Polymorphism
When working on Ruby on Rails applications, creating efficient and scalable database structures is crucial. Model inheritance and polymorphism are two powerful concepts that can help you achieve this goal. In this tutorial, we will dive deep into these concepts, exploring what they are, why they are essential, and how to implement them effectively in your Rails projects.
Table of Contents
1. Understanding Model Inheritance
1.1. What is Model Inheritance?
Model inheritance in Ruby on Rails allows you to create a hierarchy of models, where child models inherit properties and behavior from a parent model. This concept mirrors real-world relationships and is incredibly useful when you have entities with shared attributes.
For example, consider an e-commerce application where you have various types of products like clothing, electronics, and books. Instead of creating separate models for each product type, you can create a parent model called Product with common attributes like name, price, and description. Child models like Clothing, Electronics, and Books can inherit from the Product model, inheriting its attributes and methods.
1.2. Why Use Model Inheritance?
- Reusability: Model inheritance promotes code reuse. You define common attributes and methods in the parent model, reducing duplication and making your code more maintainable.
- Scalability: As your application grows, you can easily add new child models without affecting the existing codebase. This makes your application more scalable and adaptable to changing requirements.
- Organization: Model inheritance provides a clean and organized structure for your database schema. It reflects the relationships between different entities, making your codebase more intuitive.
1.3. Implementing Model Inheritance in Rails
Let’s implement model inheritance in a Rails application using the example of an e-commerce platform.
ruby # app/models/product.rb class Product < ApplicationRecord # Common attributes and methods for all products end # app/models/clothing.rb class Clothing < Product # Additional attributes and methods specific to clothing products end # app/models/electronics.rb class Electronics < Product # Additional attributes and methods specific to electronics products end # app/models/books.rb class Books < Product # Additional attributes and methods specific to book products end
In the code above:
- We define a parent model, Product, which contains common attributes and methods for all products.
- Child models like Clothing, Electronics, and Books inherit from the Product model.
- Each child model can have its specific attributes and methods in addition to those inherited from the parent.
2. Embracing Polymorphism
2.1. What is Polymorphism?
Polymorphism is a fundamental concept in object-oriented programming that allows objects of different classes to be treated as instances of a common superclass. In Rails, polymorphism often comes into play when dealing with associations between models, such as when a model can belong to multiple other models.
For instance, consider a commenting system where users can comment on both articles and photos. Instead of creating separate tables for article comments and photo comments, you can use polymorphism to create a single Comment model that can be associated with both Article and Photo models.
2.2. Advantages of Polymorphism
- Simplicity: Polymorphism simplifies your data model by eliminating the need for multiple tables to represent similar concepts. This leads to a cleaner and more maintainable database schema.
- Flexibility: With polymorphism, you can easily extend your application to support new types of associations without modifying the database structure.
- Code Reusability: By using a common interface (e.g., commentable), you can write generic code that works with different types of objects.
2.3. Implementing Polymorphism in Rails
Let’s see how to implement polymorphism in a Rails application using the example of a commenting system.
ruby # app/models/comment.rb class Comment < ApplicationRecord belongs_to :commentable, polymorphic: true end # app/models/article.rb class Article < ApplicationRecord has_many :comments, as: :commentable end # app/models/photo.rb class Photo < ApplicationRecord has_many :comments, as: :commentable end
In this example:
- We define a Comment model with a commentable association, marked as polymorphic.
- The Article and Photo models each have a has_many association with comments, specifying as: :commentable. This indicates that they can be commented on.
3. Combining Model Inheritance and Polymorphism
3.1. Building a Practical Example
Let’s combine model inheritance and polymorphism to build a practical example: a content management system (CMS) that handles various types of content, including articles and photos.
ruby # app/models/content.rb class Content < ApplicationRecord belongs_to :author end # app/models/article.rb class Article < Content has_many :comments, as: :commentable end # app/models/photo.rb class Photo < Content has_many :comments, as: :commentable end
In this example:
- We have a parent model, Content, representing generic content with an author association.
- The Article and Photo models inherit from Content, inheriting the author association.
- Both Article and Photo can have comments associated with them using polymorphism.
3.2. Leveraging the Power of Inheritance and Polymorphism
By combining model inheritance and polymorphism, we’ve created a flexible and extensible system for managing various types of content. Here’s how we can leverage the power of these concepts:
- Extensibility: If you need to add new content types in the future, you can create additional child models of Content without modifying existing code. This keeps your application scalable and adaptable.
- Consistency: Since all content types share common attributes like author, you can write generic code for tasks like displaying the author’s name, making your codebase more DRY (Don’t Repeat Yourself).
- Comments: Thanks to polymorphism, you can use the same Comment model to handle comments on both articles and photos. This simplifies your database structure and makes it easier to work with comments.
Conclusion
In this tutorial, we’ve explored the concepts of model inheritance and polymorphism in Ruby on Rails. Model inheritance allows you to create hierarchies of models, promoting code reusability and organization. Polymorphism, on the other hand, simplifies your data model by allowing objects of different classes to be treated as instances of a common superclass.
By combining these two powerful concepts, you can create efficient and scalable database structures in your Rails applications. Whether you’re building a content management system, an e-commerce platform, or any other application with diverse data types, model inheritance and polymorphism are valuable tools in your Rails development toolbox. Embrace these concepts, and you’ll be well-equipped to create robust and maintainable Rails applications.
Table of Contents
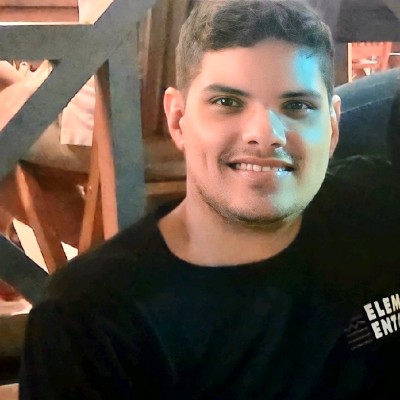
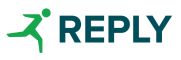