Ruby on Rails Tutorial: Understanding Model Validations
Data integrity is a crucial aspect of any web application. As a developer, you want to ensure that the data entered into your application is accurate, consistent, and valid. Ruby on Rails provides a robust set of features for enforcing data integrity, and one of the key tools in your arsenal is model validations.
In this tutorial, we will dive deep into understanding model validations in Ruby on Rails. We will explore different types of validations, their purpose, and how to implement them effectively. By the end of this tutorial, you will have a solid understanding of how to validate data in your Rails models and ensure that your application maintains data integrity.
Why are Model Validations Important?
Ensuring data integrity is crucial in any application. By validating data at the model level, we can prevent the insertion of invalid or inconsistent data into our database. Model validations provide a way to define rules and constraints that our data must adhere to, allowing us to catch errors early and maintain clean and reliable data.
Getting Started with Model Validations
1. Adding a Simple Validation
Let’s start by adding a simple validation to our model. Suppose we have a User model with a name attribute, and we want to ensure that the name is present. We can achieve this by adding the following line to our model:
ruby class User < ApplicationRecord validates :name, presence: true end
2. Validation Options and Customization
Model validations offer a range of options and customization to suit various scenarios. For instance, we can specify a custom error message for a validation rule, restrict the length of a string attribute, or define a regular expression pattern for a format validation. Understanding these options allows us to fine-tune our validations to meet the specific requirements of our application.
Common Validation Types
1. Presence Validation
The presence validation ensures that a particular attribute is not empty or nil. It is commonly used to validate the presence of required fields. Here’s an example:
ruby class Article < ApplicationRecord validates :title, presence: true end
2. Length Validation
The length validation enables us to set constraints on the length of string attributes. We can specify minimum, maximum, or exact length requirements. Let’s consider an example where we want to limit the length of a password attribute:
ruby class User < ApplicationRecord validates :password, length: { minimum: 8, maximum: 20 } end
3. Format Validation
The format validation allows us to validate the format of a string attribute using regular expressions. It is useful when we need to enforce specific patterns, such as validating email addresses or phone numbers. Here’s an example of validating an email format:
ruby class User < ApplicationRecord validates :email, format: { with: URI::MailTo::EMAIL_REGEXP } end
4. Uniqueness Validation
The uniqueness validation ensures that a particular attribute is unique within the context of the model. It is commonly used to validate unique usernames or email addresses. Here’s an example:
ruby class User < ApplicationRecord validates :username, uniqueness: true end
5. Numericality Validation
The numericality validation ensures that an attribute is a valid number. It allows us to validate attributes based on conditions such as greater than, less than, or equal to a certain value. Let’s consider an example where we want to ensure that the age attribute is a positive integer:
ruby class Person < ApplicationRecord validates :age, numericality: { only_integer: true, greater_than: 0 } end
Conditional Validations
Sometimes we need to apply validations only under certain conditions. Ruby on Rails provides multiple ways to achieve conditional validations, such as using :if and :unless options, methods, or Procs.
1. Conditional Validation using ‘:if’ and ‘:unless’
The :if and :unless options allow us to specify conditions under which a validation should be applied. We can use these options to check other attribute values or call methods to determine whether a validation should be executed. Here’s an example:
ruby class Order < ApplicationRecord validates :shipping_address, presence: true, if: :requires_shipping? def requires_shipping? shipping_required == true end end
2. Conditional Validation using Methods
We can also use methods to define our own conditions for validations. By convention, if the method name matches the validation name (without the “validate” part), Rails automatically applies the validation. Let’s consider an example where we want to validate the expiration_date attribute only if the credit_card attribute is present:
ruby class Payment < ApplicationRecord validate :expiration_date, if: :credit_card_present? def credit_card_present? credit_card.present? end end
3. Conditional Validation using Proc
For more complex scenarios, we can use a Proc object to define custom conditions. The Proc should return a boolean value to indicate whether the validation should be executed. Here’s an example where we want to validate the phone_number attribute only if the country is set to “United States”:
ruby class Contact < ApplicationRecord validates :phone_number, presence: true, if: Proc.new { |c| c.country == 'United States' } end
Validation Error Messages
1. Displaying Validation Error Messages
When a validation fails, Rails automatically adds an error message to the corresponding attribute. We can access these error messages and display them to the user. Let’s explore how to display validation error messages in our views:
erb <% if @user.errors.any? %> <div id="error_explanation"> <h2><%= pluralize(@user.errors.count, "error") %> prohibited this user from being saved:</h2> <ul> <% @user.errors.full_messages.each do |message| %> <li><%= message %></li> <% end %> </ul> </div> <% end %>
2. Customizing Validation Error Messages
Rails provides ways to customize validation error messages according to our application’s needs. We can modify the error messages for specific attributes or add custom error messages for complex validations. Here’s an example:
ruby class User < ApplicationRecord validates :username, presence: { message: "Please provide a username." } validates :email, format: { with: URI::MailTo::EMAIL_REGEXP, message: "Please provide a valid email address." } end
3. Composite Validations and Callbacks
3.1 Combining Validations
In complex scenarios, we often need to combine multiple validations to ensure data integrity. Rails allows us to chain multiple validations together using logical operators like && and ||. Let’s consider an example where we want to validate a Post model that requires both a title and content:
ruby class Post < ApplicationRecord validates :title, presence: true validates :content, presence: true end
3.2 Using Callbacks with Validations
Callbacks provide a way to trigger certain actions before or after validations. They are useful when we need to perform additional tasks, such as formatting data or generating unique identifiers, during the validation process. Rails provides several callbacks, including before_validation, after_validation, before_save, and after_save.
Handling Validations in Controller and Views
1. Checking Validity in the Controller
In our Rails controllers, we can use the valid? or invalid? methods to check if the model’s data passes all validations. These methods return a boolean value indicating the validity of the model. Here’s an example:
ruby class UsersController < ApplicationController def create @user = User.new(user_params) if @user.valid? # Process the data else render :new end end end
2. Displaying Validation Errors in the Views
We have already seen how to display validation error messages, but there are other ways to provide feedback to the user. For instance, we can use CSS styling to highlight the invalid fields or show validation errors inline. Implementing such visual cues can improve the user experience and make error handling more intuitive.
Best Practices for Model Validations
1. Keeping Validations Simple
While validations are powerful, it’s essential to strike a balance and avoid overcomplicating them. As a best practice, keep your validations focused, concise, and easy to understand. Split complex validations into smaller, reusable methods and use validation helpers whenever possible.
2. Testing Validations
Unit tests play a crucial role in ensuring the correctness of our validations. By writing tests that cover various scenarios, we can catch potential issues early and have confidence in the behavior of our validations. Use testing frameworks like RSpec or MiniTest to create comprehensive test suites for your models.
3. Organizing Validations
As your application grows, managing validations can become challenging. It is a good practice to group related validations together in your model classes. You can also consider using concerns or modules to extract common validations and include them in multiple models.
Conclusion
Model validations in Ruby on Rails provide a powerful way to enforce data integrity in your applications. By implementing appropriate validations, you can ensure that the data entered into your application meets the required criteria, preventing data inconsistencies and enhancing the overall user experience. Throughout this tutorial, we have explored various types of validations, conditional validations, error messages, composite validations, and best practices for using validations effectively. Armed with this knowledge, you can confidently build Rails applications that maintain data integrity and provide a reliable user experience.
Table of Contents
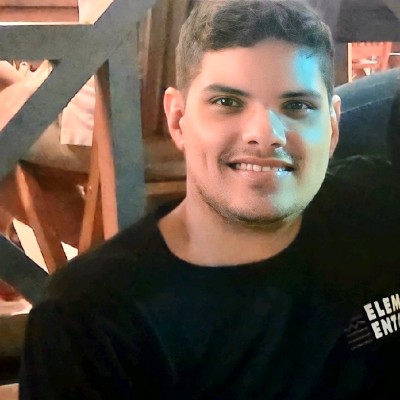
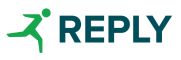