Ruby on Rails Tutorial: Getting Started with MVC Architecture
If you’re new to web development or just starting with Ruby on Rails, understanding the MVC architecture can feel like a daunting task. This architectural pattern, which stands for Model-View-Controller, is the backbone of many web applications, and Rails is no exception.
In this tutorial, we’ll explore the MVC architecture and how it works in the Rails ecosystem, guiding you through the building of a simple web application.
What is MVC Architecture?
MVC stands for Model-View-Controller. It’s a design pattern that separates an application into three interconnected components. This separation allows for efficient code organization, modularization, and scalability.
Model: This is the part of the system that handles logic for data manipulation and database interaction. It communicates with the database, carrying out the necessary CRUD (Create, Read, Update, Delete) operations.
View: It is responsible for everything the user visually interacts with – the UI. Views are typically made of HTML, CSS, JavaScript, and embedded Ruby (.erb) files.
Controller: As the name implies, this acts as the middleman between the Model and the View. It receives user input from the View, processes it (often involving interactions with the Model), and returns the output to the View.
Setting up Your Environment
Before we start, ensure that you have Ruby and Rails installed in your development environment. If you haven’t, you can refer to the official Rails guide for [installation instructions] (https://guides.rubyonrails.org/getting_started.html).
Creating a New Rails Project
Once you’ve got everything set up, open your terminal, navigate to your projects directory, and create a new Rails project by running:
rails new my_first_rails_app
This command will create a new Rails application named ‘my_first_rails_app‘ with a default directory structure. The structure automatically adheres to the MVC pattern, which we will explore next.
Understanding the MVC Pattern in Rails
In the Rails directory structure, you’ll see three folders corresponding to MVC: `app/models`, `app/views`, and `app/controllers`. Each of these folders is intended to house files corresponding to their respective components.
Models
In your Rails project, create a model by running:
rails generate model Article title:string body:text
This command will create an `Article` model with a title and body. It also creates a migration file for the database table ‘articles’. To run the migration, use the following command:
rails db:migrate
Controllers
To generate a controller for your articles, run:
rails generate controller Articles
This will generate an `ArticlesController` with its own directory under `app/views`. In your `articles_controller.rb` file, you can define methods (also called actions) for various operations. For example, the `index` action retrieves all articles:
def index @articles = Article.all end
Views
Under `app/views/articles`, create a new file `index.html.erb`. This file will be used to display all articles. Here, you can write HTML and embedded Ruby to display each article’s title and body:
<% @articles.each do |article| %> <h2><%= article.title %></h2> <p><%= article.body %></p> <% end %>
This view will be automatically rendered when the `index` action of `ArticlesController` is called.
Routing in Rails
Rails also provides a robust routing system. In `config/routes.rb`, define a route for your articles:
Rails.application.routes .draw do get 'articles', to: 'articles#index' end
This tells Rails to route GET requests for ‘/articles’ to the `index` action of `ArticlesController`.
Starting Your Server
Start your Rails server by running:
rails server
Now, open your browser and navigate to `localhost:3000/articles`. You will see your articles displayed as per your `index` action and view.
Conclusion
Through the creation of this simple Rails application, we’ve explored the MVC architecture’s role and how it helps structure our application effectively. We’ve seen how Models communicate with the database, how Views present data, and how Controllers act as intermediaries, directing the flow of information and control.
Mastering the MVC architecture will provide you with a solid foundation for further exploration into the vast and powerful world of Ruby on Rails. Happy coding!
Table of Contents
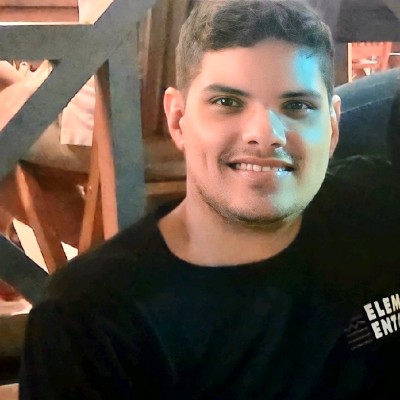
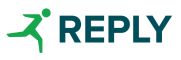