Introduction to Object-Oriented Programming with Ruby on Rails
Ruby on Rails, often referred to as Rails, is a popular web development framework written in Ruby. It promotes the use of web standards and is famed for its ‘convention over configuration’ approach to development. What’s more, Rails is built around the concept of Object-Oriented Programming (OOP), a fundamental paradigm in software development that structures a program around ‘objects’, instances of classes representing certain entities.
What is Object-Oriented Programming (OOP)?
Object-Oriented Programming (OOP) is a programming paradigm that organizes data and methods into structures called objects. These objects are instances of classes, blueprints that define the properties (attributes) and behaviors (methods) that the instantiated objects will have. OOP facilitates code organization, reusability, and maintainability.
The Four Pillars of OOP
OOP is built upon four main principles: encapsulation, inheritance, polymorphism, and abstraction.
- Encapsulation: This principle is about wrapping data (variables) and methods into a single unit called a class. It provides a way to restrict access to certain components and prevent the data from being directly accessed or modified.
- Inheritance: This is a mechanism in which one class acquires the properties and behaviors of another class. It allows developers to create new classes that reuse, extend, and modify the behavior defined in other classes.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a superclass. It provides a way to use a class exactly like its parent, so there’s no need to overload the function.
- Abstraction: Abstraction is the process of simplifying complex systems by modeling classes appropriate to the problem, and working at the most appropriate level of inheritance for a particular aspect of the problem.
Now, let’s delve into how Ruby on Rails applies these concepts.
Classes and Objects in Ruby
In Ruby, classes are defined using the ‘class’ keyword. Here’s an example:
class Dog def bark puts 'Woof!' end end fido = Dog.new fido.bark # Outputs: 'Woof!'
In this case, `Dog` is the class, and `fido` is an instance (or object) of that class. `bark` is a method available to objects of the `Dog` class. When `fido.bark` is called, it executes the `bark` method, outputting ‘Woof!’.
Encapsulation in Ruby
Encapsulation is implemented in Ruby using methods and instance variables. Instance variables are defined using the `@` symbol and are only accessible from within the class. Here’s an example of encapsulation in Ruby:
class Dog def initialize(name) @name = name end def get_name @name end end fido = Dog.new('Fido') puts fido.get_name # Outputs: 'Fido'
In this case, the `Dog` class has an instance variable `@name`, which is encapsulated within the class. It can be accessed using the `get_name` method.
Inheritance in Ruby
Ruby uses the `<` symbol to denote inheritance. For example:
class Animal def initialize(name) @name = name end def move puts "#{@name} is moving." end end class Dog < Animal def bark puts 'Woof!' end end fido = Dog.new('Fido') fido.move # Outputs: 'Fido is moving.' fido.bark # Outputs: 'Woof!'
Here, the `Dog` class inherits from the `Animal` class, which means that `Dog` instances have access to methods defined in `Animal`.
Polymorphism in Ruby
In Ruby, polymorphism is achieved through inheritance and the use of the ‘duck typing’ philosophy (if it quacks like a duck, it’s a duck). Here’s an example:
class Animal def initialize(name) @name = name end def speak "#{@name} says " end end class Dog < Animal def speak super + 'Woof!' end end class Cat < Animal def speak super + 'Meow!' end end fido = Dog.new('Fido') whiskers = Cat.new('Whiskers') puts fido.speak # Outputs: 'Fido says Woof!' puts whiskers.speak # Outputs: 'Whiskers says Meow!'
In this case, both `Dog` and `Cat` are subclasses of `Animal` and redefine the `speak` method. This is polymorphism in action.
Abstraction in Ruby
Abstraction in Ruby can be implemented using modules, which are like classes but cannot be instantiated. They’re used to group related methods that can be included in multiple classes. Here’s an example:
module Movable def move puts "#{@name} is moving." end end class Animal include Movable def initialize(name) @name = name end end fido = Animal.new('Fido') fido.move # Outputs: 'Fido is moving.'
In this case, the `Movable` module contains the `move` method, which is included in the `Animal` class.
Conclusion
Understanding the principles of Object-Oriented Programming is fundamental to becoming a proficient Ruby on Rails developer. By applying the concepts of encapsulation, inheritance, polymorphism, and abstraction, we can write code that is reusable, maintainable, and easy to understand. Ruby’s simplicity and expressiveness make it a great language for learning and applying these concepts. Happy coding!
Table of Contents
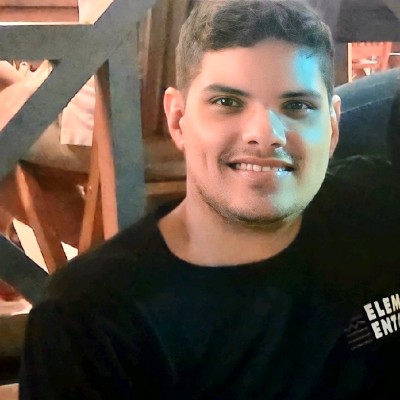
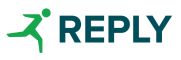