Ruby on Rails Q & A
How to optimize database queries in Rails?
Optimizing Rails database queries is essential for maintaining a performant application, especially as it scales. Here are key strategies to achieve this:
- Eager Loading: One common issue in Rails applications is the “N+1 query problem.” This happens when you load a parent object and its associated child objects separately. The solution is to use `includes` or `eager_load` to load associated records in one or a few queries rather than many.
```ruby # Instead of posts = Post.all posts.each { |post| post.author.name } # Use posts = Post.includes(:author) posts.each { |post| post.author.name } ```
- Select Only What You Need: Instead of selecting all columns, which is the default behavior of `ActiveRecord::Base#all`, use `select` to only pick the columns you need.
```ruby User.select(:id, :name) ```
- Use Indices: Ensure that columns used frequently in WHERE clauses, JOIN operations, or as foreign keys have database indices. This can drastically speed up query times.
- Batch Processing: When dealing with large datasets, `find_each` or `find_in_batches` can be used to retrieve records in batches, preventing memory bloat.
- Caching: Cache results of expensive or frequently-run queries using Rails caching mechanisms.
- Review the SQL: The `to_sql` method can be used to see the actual SQL that will be executed. This can be useful for identifying inefficiencies.
```ruby User.where(name: 'Alice').to_sql ```
- Database Engine Tuning: While not specific to Rails, fine-tuning your database configuration settings can have significant impacts on performance.
- Utilize `EXPLAIN`: Most databases provide an `EXPLAIN` command that gives insights into the SQL query execution plan. This can be invaluable in understanding what the database is doing and where potential bottlenecks might be.
Optimizing Rails database queries is about understanding the SQL generated by ActiveRecord and making intelligent decisions on data retrieval and database structure. Regularly reviewing and refactoring queries, combined with monitoring and profiling tools, will ensure your Rails app remains efficient as it grows.
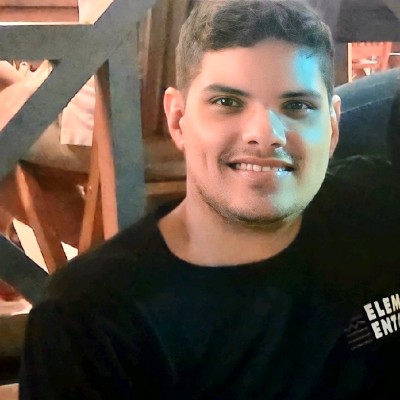
Previously at
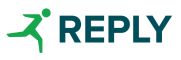
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.