How to use partials in views on Rails?
In Rails, partials are special view templates that promote DRY (Don’t Repeat Yourself) principles by allowing you to reuse portions of your views across different templates. Using partials can enhance the maintainability and readability of your views. Let’s delve into how they function:
- Naming Convention: Partials are prefixed with an underscore when named. For example, a partial handling user information might be named `_user.html.erb`.
- Rendering Partials: You can include a partial in a view using the `render` method. For our `_user.html.erb` partial, it would look like:
```ruby <%= render 'user' %> ```
Note that you don’t include the leading underscore or the file extension when referencing the partial.
- Passing Local Variables: Often, you’ll want to pass data to a partial. You can do this using local variables:
```ruby <%= render 'user', user: @user %> ```
Inside the `_user.html.erb` partial, you can then access the `user` variable directly.
- Collection Rendering: If you want to render a partial multiple times for items in a collection, Rails provides a shorthand:
```ruby <%= render partial: 'user', collection: @users %> ```
This will render the `_user.html.erb` partial for each user in the `@users` array. Inside the partial, the current item from the collection will be available as a local variable named after the partial (in this case, `user`).
- Shared Partials: If you find that a partial is used across multiple controllers, it’s a good practice to place it in a shared directory, like `app/views/shared/`. You can then render it with:
```ruby <%= render 'shared/user' %> ```
- Layouts with Partials: You can also use partials to break down complex layouts into more manageable pieces. For instance, you might have partials for the header, footer, or sidebar components of a site layout.
Partials in Rails views are instrumental in organizing view code, promoting code reuse, and simplifying complex views. They allow developers to create modular and maintainable view structures, ensuring that updates or changes to the UI can be implemented efficiently and consistently.
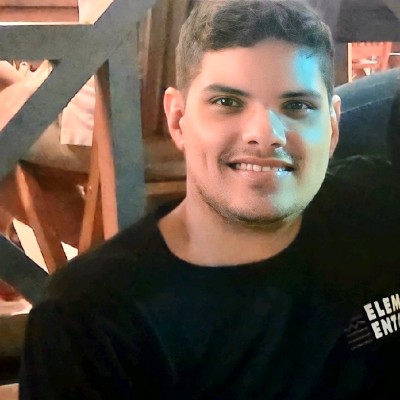
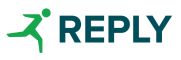