How to Use Ruby Functions for Payment Processing
In today’s digital age, online payments have become an integral part of e-commerce and various other web applications. Whether you’re running an online store, a subscription service, or a donation platform, the ability to process payments efficiently and securely is essential. Ruby, a dynamic and versatile programming language, is well-equipped to handle payment processing tasks. In this blog post, we’ll explore how to use Ruby functions for payment processing, including integrating payment gateways, working with APIs, and ensuring secure transactions.
Table of Contents
1. Choosing the Right Payment Gateway
1.1 Understanding Payment Gateways
A payment gateway is a service that acts as a bridge between your application and the financial institutions that process payments. It facilitates the transfer of payment information between your application, the customer, and the bank. When choosing a payment gateway for your Ruby application, consider factors like:
- Fees: Different gateways have different fee structures. Be sure to understand their pricing and how it fits your budget.
- Geographic Coverage: Ensure that the payment gateway supports transactions in the regions you operate.
- Integration: Look for gateways that offer easy integration with Ruby. Many providers offer Ruby gems for seamless integration.
- Security: Prioritize security. The gateway should comply with industry-standard security practices to protect sensitive customer data.
1.2 Popular Payment Gateways for Ruby
Ruby developers have access to a wide range of payment gateways, each with its own set of features and integrations. Some popular payment gateways that work well with Ruby include:
- Stripe: Stripe offers a robust Ruby gem that simplifies integration. It supports one-time payments, subscriptions, and more.
- PayPal: PayPal provides both RESTful APIs and classic APIs for Ruby integration. It’s widely recognized and trusted by users.
- Braintree: A subsidiary of PayPal, Braintree offers a Ruby gem and focuses on providing a frictionless payment experience.
- Authorize.Net: Authorize.Net offers a Ruby SDK for integrating payments into your application. It’s known for its reliability.
Choose the payment gateway that best suits your project’s requirements and budget.
2. Setting Up Your Development Environment
Before you dive into coding, make sure you have a Ruby development environment set up. Here are the basic steps to get started:
- Install Ruby: You can download and install Ruby from the official website or use a version manager like RVM or rbenv.
- Install a Code Editor: Choose a code editor that you are comfortable with, such as Visual Studio Code, Sublime Text, or RubyMine.
- Set Up Version Control: Use Git for version control to track changes in your codebase.
- Install Dependencies: Ensure you have Bundler installed, which is a gem manager for Ruby. You’ll use it to manage project dependencies.
Once your development environment is ready, you can proceed with integrating the chosen payment gateway into your Ruby application.
3. Installing Necessary Gems
Ruby gems are packages that provide pre-built functionality and can save you a significant amount of development time. To integrate a payment gateway into your Ruby application, you’ll need to install the corresponding gem. For instance, if you’re using Stripe, you can install the Stripe gem with the following command:
ruby gem install stripe
Make sure to check the documentation of the payment gateway for specific installation instructions.
4. Building Payment Processing Functions
Now comes the exciting part—building the payment processing functions in Ruby. Depending on your application’s needs, you might need to handle one-time payments, refunds, or subscriptions.
4.1 Handling Payments
To handle one-time payments, you’ll need to create a function that captures payment information, communicates with the payment gateway, and confirms the transaction’s success. Here’s a simplified example using the Stripe gem:
ruby require 'stripe' Stripe.api_key = 'your_secret_api_key' def process_payment(amount, card_token) begin charge = Stripe::Charge.create( amount: amount, currency: 'usd', source: card_token ) # Payment successful return true rescue Stripe::CardError => e # Payment failed return false end end
In this example, we set up the Stripe API key, create a process_payment function, and handle exceptions for failed payments.
4.2 Handling Refunds
Refunds are an essential part of payment processing. You might need to refund customers for various reasons. Here’s a basic refund function using the Stripe gem:
ruby def process_refund(charge_id) begin charge = Stripe::Refund.create( charge: charge_id ) # Refund successful return true rescue Stripe::InvalidRequestError => e # Refund failed return false end end
This function takes the charge ID as an argument and initiates the refund process.
4.3 Handling Subscriptions
If your application offers subscription-based services, you’ll need to set up functions to manage subscriptions. Using the Stripe gem, you can create a subscription like this:
ruby def create_subscription(customer_id, plan_id) begin subscription = Stripe::Subscription.create( customer: customer_id, items: [{ plan: plan_id }] ) # Subscription successful return true rescue Stripe::InvalidRequestError => e # Subscription failed return false end end
This function takes the customer’s ID and the plan ID as arguments and creates a subscription.
5. Handling Webhooks
Webhooks are essential for handling events related to payments, such as successful payments, failed payments, or subscription cancellations. Payment gateways typically send these events to your server in real-time, allowing you to update your application’s database or take specific actions.
To handle webhooks in Ruby, you can use a web framework like Ruby on Rails or Sinatra. Here’s a simplified example of a Sinatra route to handle a Stripe webhook:
ruby require 'sinatra' require 'stripe' Stripe.api_key = 'your_secret_api_key' post '/webhook/stripe' do payload = request.body.read sig_header = request.env['HTTP_STRIPE_SIGNATURE'] begin event = Stripe::Webhook.construct_event( payload, sig_header, 'your_webhook_secret' ) rescue JSON::ParserError => e return 400 rescue Stripe::SignatureVerificationError => e return 400 end # Handle the event based on its type case event.type when 'payment_intent.succeeded' # Handle successful payment when 'payment_intent.payment_failed' # Handle failed payment when 'customer.subscription.deleted' # Handle subscription cancellation end 200 end
In this example, we set up a Sinatra route to listen for Stripe webhook events and handle them accordingly.
6. Ensuring Security
Security is paramount when handling payments. Here are some best practices to ensure the security of your payment processing functions:
- Use HTTPS: Always transmit payment data over HTTPS to encrypt the information in transit.
- Tokenization: Avoid storing sensitive card information on your server. Use tokenization services provided by the payment gateway.
- PCI Compliance: Comply with Payment Card Industry Data Security Standard (PCI DSS) requirements to safeguard cardholder data.
- Regular Updates: Keep your Ruby gems and dependencies up to date to patch any security vulnerabilities.
7. Testing Your Payment Functions
Testing is crucial to ensure that your payment processing functions work as expected. Use testing frameworks like RSpec or MiniTest to write unit tests for your functions. Create test cases that cover different scenarios, including successful payments, failed payments, refunds, and subscription management.
Here’s an example of testing a payment function using RSpec:
ruby require 'rspec' require 'your_payment_module' describe 'Payment Processing' do it 'successfully processes a payment' do result = process_payment(1000, 'valid_card_token') expect(result).to be(true) end it 'fails to process an invalid payment' do result = process_payment(1000, 'invalid_card_token') expect(result).to be(false) end end
Running automated tests will help you catch and fix issues early in the development process.
Conclusion
In this guide, we’ve explored how to use Ruby functions for payment processing. We discussed the importance of choosing the right payment gateway, setting up your development environment, installing necessary gems, and building payment processing functions. Additionally, we covered handling webhooks, ensuring security, and testing your payment functions.
Payment processing can be a complex task, but Ruby and its ecosystem of gems make it more manageable. With the right tools and practices in place, you can build secure and efficient payment processing systems for your web applications. So, go ahead and integrate payment functionality into your Ruby projects, and empower your users with seamless online transactions.
Table of Contents
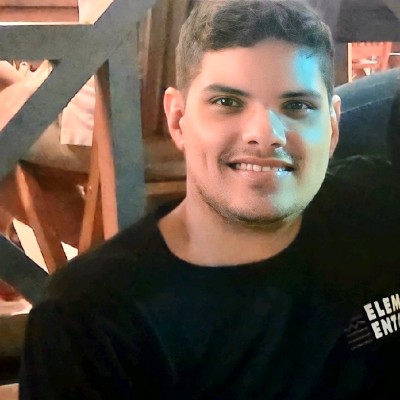
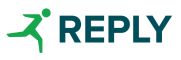