How to use polymorphic associations in Rails?
In Rails, polymorphic associations provide a way to create a model that can belong to multiple other types of models. This means that, instead of belonging to one specific model, a polymorphic model can belong to any model using a single association.
Imagine a scenario where you have multiple models, such as `Article`, `Photo`, and `Comment`, and you want all of them to be taggable. Instead of creating separate tagging associations for each model, you can create a `Tag` model with a polymorphic association, allowing you to have a more streamlined and DRY approach.
To set up a polymorphic association:
- Database Migration:
You’ll need two fields on the model that will be polymorphically associated (e.g., `Tag`): one to store the ID of the associated record (`taggable_id`) and another to store the type of the associated record (`taggable_type`).
```ruby create_table :tags do |t| t.references :taggable, polymorphic: true, null: false t.string :name t.timestamps end ```
- Model Configuration:
For the model that will be polymorphically associated (`Tag`):
```ruby class Tag < ApplicationRecord belongs_to :taggable, polymorphic: true end ```
For the models that will have the polymorphic association (`Article`, `Photo`, `Comment`):
```ruby class Article < ApplicationRecord has_many :tags, as: :taggable end class Photo < ApplicationRecord has_many :tags, as: :taggable end class Comment < ApplicationRecord has_many :tags, as: :taggable end ```
With these configurations in place, you can now associate tags with any of the models:
```ruby article = Article.first tag = article.tags.create(name: 'informative') ```
Polymorphic associations simplify and generalize relationships across different models, making the codebase more maintainable and logical. However, be cautious when using them, as they can complicate queries and sometimes lead to performance issues if not used judiciously.
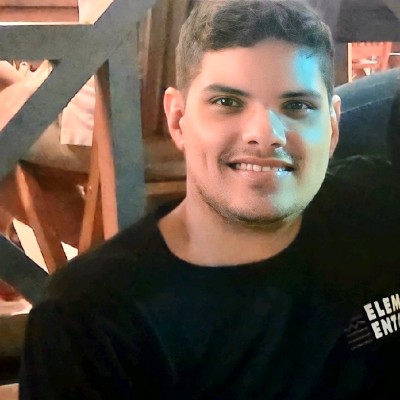
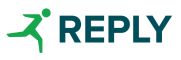